If you are developing and Android Application wherein you want to integrate WhatsApp App to send messages to your contacts, you can achieve it simply by explicit Intents.
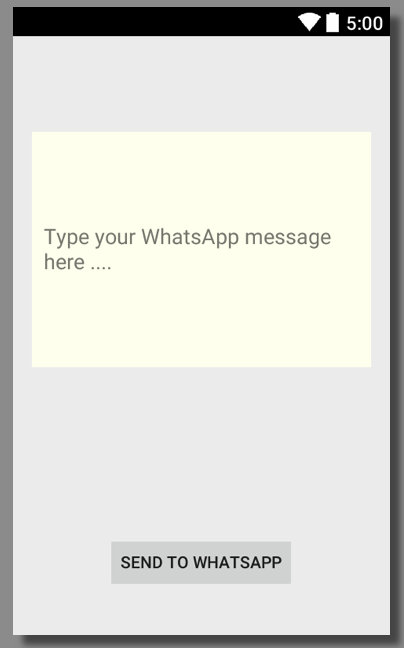
Sending WhatsApp Message form your App Tutorial.png
Let's take a simple example for demonstration,
We create an Android App with a Layout.xml containing an EditText to type a message and Button. When the button is clicked we fetch the value of the EditText and send it to WhatsApp application using Intent.
activity_main.xml
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.code2care.example.whatsappintegrationexample.MainActivity"
tools:ignore="HardcodedText" >
android:id="@+id/message"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:onClick="sendMessae"
android:layout_marginBottom="21dp"
android:text="Send to WhatsApp" />
android:id="@+id/editText1"
android:layout_width="fill_parent"
android:hint="Type your WhatsApp message here ...."
android:layout_alignParentTop="true"
android:background="#FFFFFFEF"
android:padding="10dp"
android:layout_centerHorizontal="true"
android:layout_marginTop="65dp"
android:layout_height="200dp"
android:ems="10" />
MainActivity.java
package com.code2care.example.whatsappintegrationexample;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.view.View;
import android.widget.EditText;
public class MainActivity extends ActionBarActivity {
private EditText message;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
message = (EditText) findViewById(R.id.message);
}
public void sendMessage(View v) {
String whatsAppMessage = message.getText().toString();
Intent sendIntent = new Intent();
sendIntent.setAction(Intent.ACTION_SEND);
sendIntent.putExtra(Intent.EXTRA_TEXT, whatsAppMessage);
sendIntent.setType("text/plain");
// Do not forget to add this to open whatsApp App specifically
sendIntent.setPackage("com.whatsapp");
startActivity(sendIntent);
}
}
More Posts related to WhatsApp,
- How to know if someone has read your WhatsApp message
- WhatsApp Web escanner
- How to resolve Certificate Expired WhatsApp Error
- Share Multiple Images in WhatsApp using Android Intent
- [Solution] Installing Whatsapp There's insufficient space on the device
- Share Image to WhatsApp with Caption from your Android App
- Can we move apps like WhatsApp, Facebook to external MicroSD card
- WhatsApp Keyboard shortcuts for Mac
- WhatsApp launches WhatsApp Web to Access Messages over web browser
- How to Install WhatsApp application on Mac
- Officially Send WhatsApp message using webpage (html)
- How to send WhatsApp message from your Android App using Intent
More Posts:
- How to run Java Unit Test cases with Apache Maven? - Java
- Notepad++ Happy vs Unhappy Versions - NotepadPlusPlus
- How to disable Wallpaper Tinting on macOS Sonoma 14 - MacOS
- List of Java JDK Major Minor Version Numbers - Java
- AWS CLI Change Default Output Format - AWS
- How to Skip or Ignore JUnit test cases in Java - Java
- Online JSON Validator Tool - Tools
- 42: Take a string as input and print its length. [1000+ Python Programs] - Python-Programs