In Java, you can make use of several ways to convert an array to a stream. Here are four ways to do it:
- Using Arrays.stream() method.
- Using Stream.of() method.
- Using Arrays.asList() method.
- Using Stream.Builder() method.
Let's take a look at each of them one by one,
1. Using Arrays.stream() method.
import java.util.Arrays;
import java.util.stream.Stream;
public class ArrayToSteamExample1 {
public static void main(String[] args) {
//Our String Array
String[] countryArray = {"China", "France", "USA", "Canada","Sweden"};
//String array converted to Stream using Arrays.stream()
Stream<String> countryStream = Arrays.stream(countryArray);
}
}
2. Using Stream.of() method
import java.util.stream.Stream;
public class ArrayToSteamExample2 {
public static void main(String[] args) {
//Our String Array
String[] countryArray = {"China", "France", "USA", "Canada","Sweden"};
//String array converted to Stream using Arrays.stream()
Stream<String> countryStream = Stream.of(countryArray);
}
}
3. Using Arrays.asList() method
import java.util.Arrays;
import java.util.List;
import java.util.stream.Stream;
public class ArrayToSteamExample3 {
public static void main(String[] args) {
//Our String Array
String[] countryArray = {"China", "France", "USA", "Canada","Sweden"};
//Step 1: Convert Array to List
List<String> countryList = Arrays.asList(countryArray);
//Step 2: Convert List to Steam
Stream<String> countryStream = countryList.stream();
}
}
4. Using Stream.Builder() method
import java.util.stream.Stream;
public class ArrayToSteamExample4 {
public static void main(String[] args) {
//Our String Array
String[] countryArray = {"China", "France", "USA", "Canada","Sweden"};
//Create a Stream Builder object
Stream.Builder<String> builder = Stream.builder();
for (String country : countryArray) {
builder.add(country);
}
Stream<String> countryStream = builder.build();
}
}
In the above example the Stream class in Java provides a Builder() method that can be used to create a stream of elements.
Example:
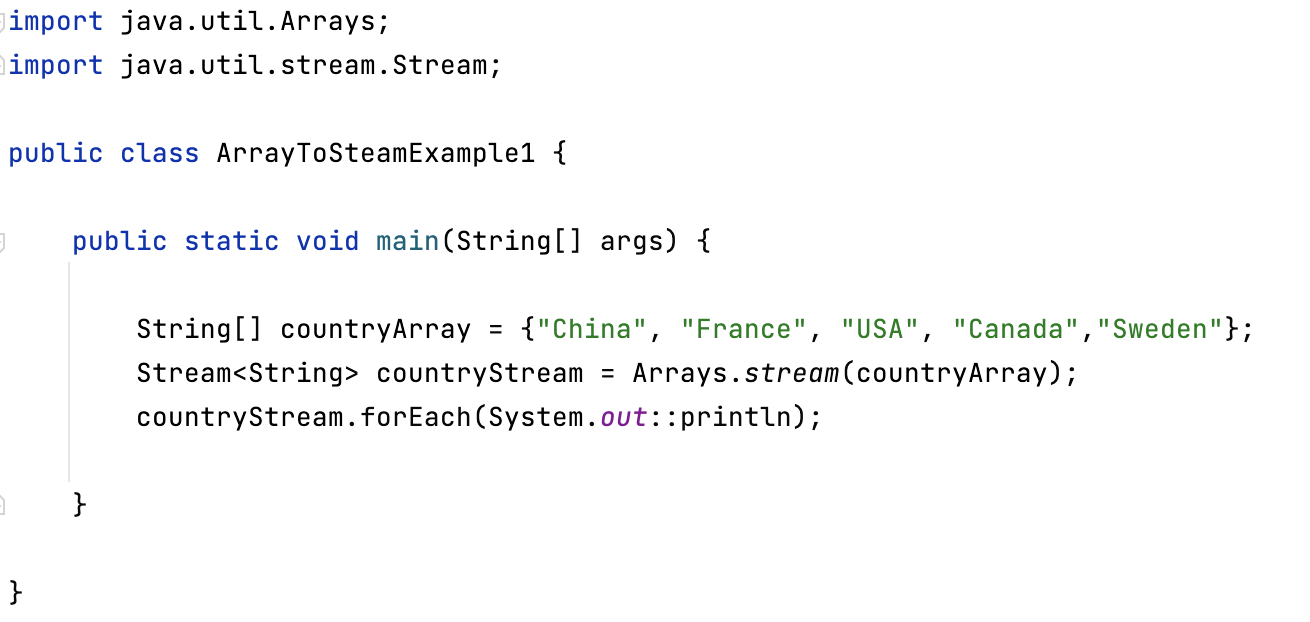
China
France
USA
Canada
Sweden
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- [Solution] Alpine Docker apt-get: not found - Docker
- Take Screenshot on Mac OS X (Keyboard Shortcuts) - Mac-OS-X
- How to change Ping TTL value on macOS - MacOS
- How to install AWS CLI on Ubuntu - AWS
- How to reload Zsh .zshrc Profile - zsh
- How to Get Substring from a String in Python using string slicing - Python
- JavaScript: Count Words in a String - JavaScript
- Calculate Area of a Rectangle - C-Program