The Functional Intrerface Predicate from the Java 8 java.util.function package has an abstract function test(T t) which takes in only one argument and returns a boolean value. If you have a requirement where you want Predicate to work with two arguments, then you should go for BiPredicate.
BiPredicate is a type of predicate (boolean-valued function) of two arguments. It is the two-arity specialization of Predicate.
It also has the same function called test but takes in two generic type arguments T and U.
Syntax: /**
* Evaluates this predicate on the given arguments.
*
* @param t the first input argument
* @param u the second input argument
* @return: if the input arguments match the predicate,
* otherwise false
*/
boolean test(T t, U u);
Let's take a look at an example of how to work with two arguments/parameters with this predicate.
package org.code2care.examples;
import java.util.function.BiPredicate;
public class PredicateWithTwoArguments {
public static void main(String... args) {
int no1 = 10;
int no2 = 10;
BiPredicate<Integer, Integer> checkEqual = Integer::equals;
if (checkEqual.test(no1, no2)) {
System.out.println(no1 + " and " + no2 + " are equal");
} else {
System.out.println(no1 + " and " + no2 + " are not equal");
}
}
}
Output:
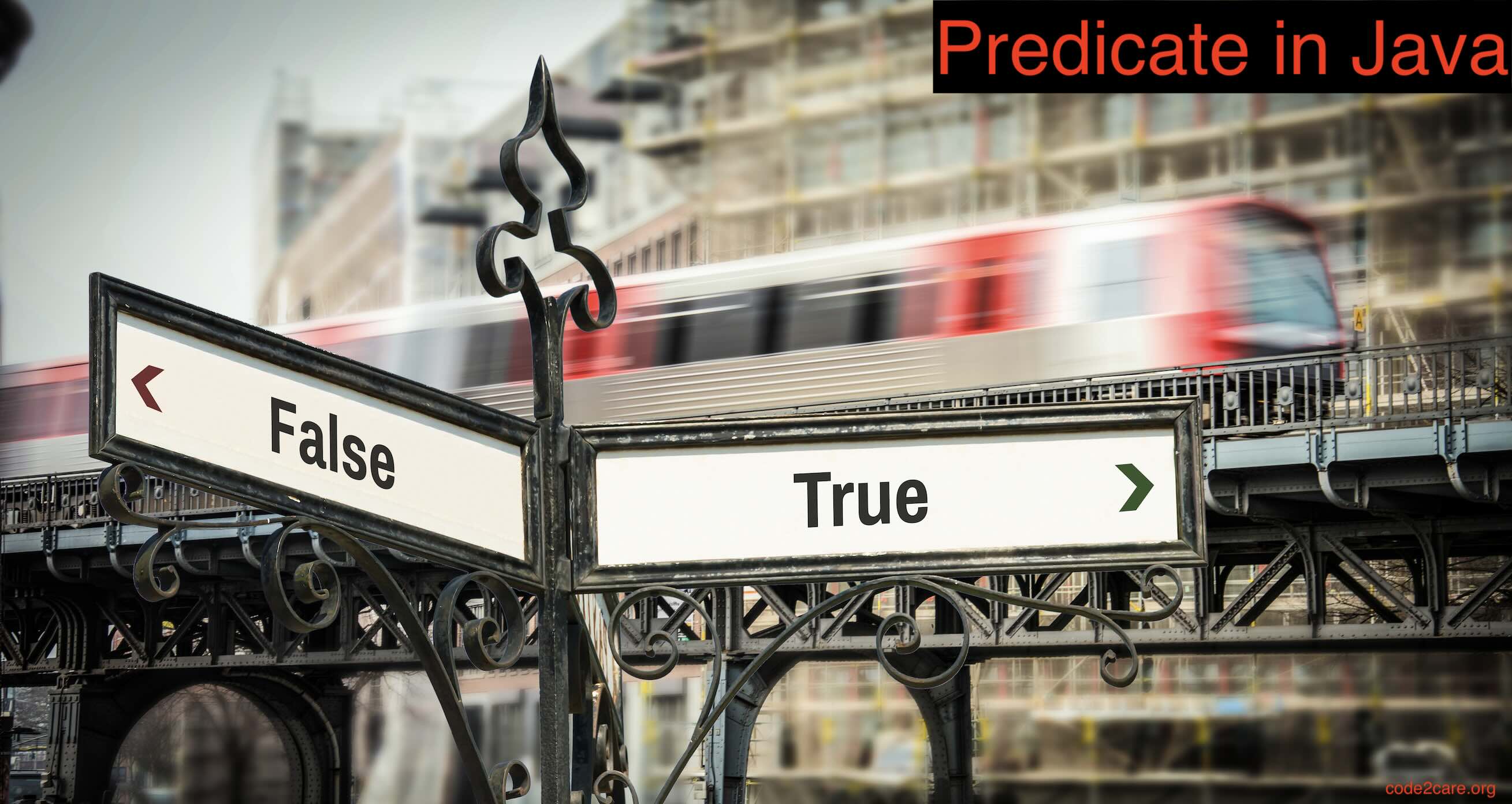
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Get List of all local branches git command - Git
- How to make div or text in html unselectable using CSS - CSS
- Compare two text files in Notepad++ - NotepadPlusPlus
- How to Use Command Prompt on a Mac? - MacOS
- macOS Big Sur compatible Macs List - MacOS
- [Docker] Install Git on Alpine Linux - Docker
- Bootstrap Nav Menu Dropdown on hover - Bootstrap
- How to find someone on Instagram - HowTos