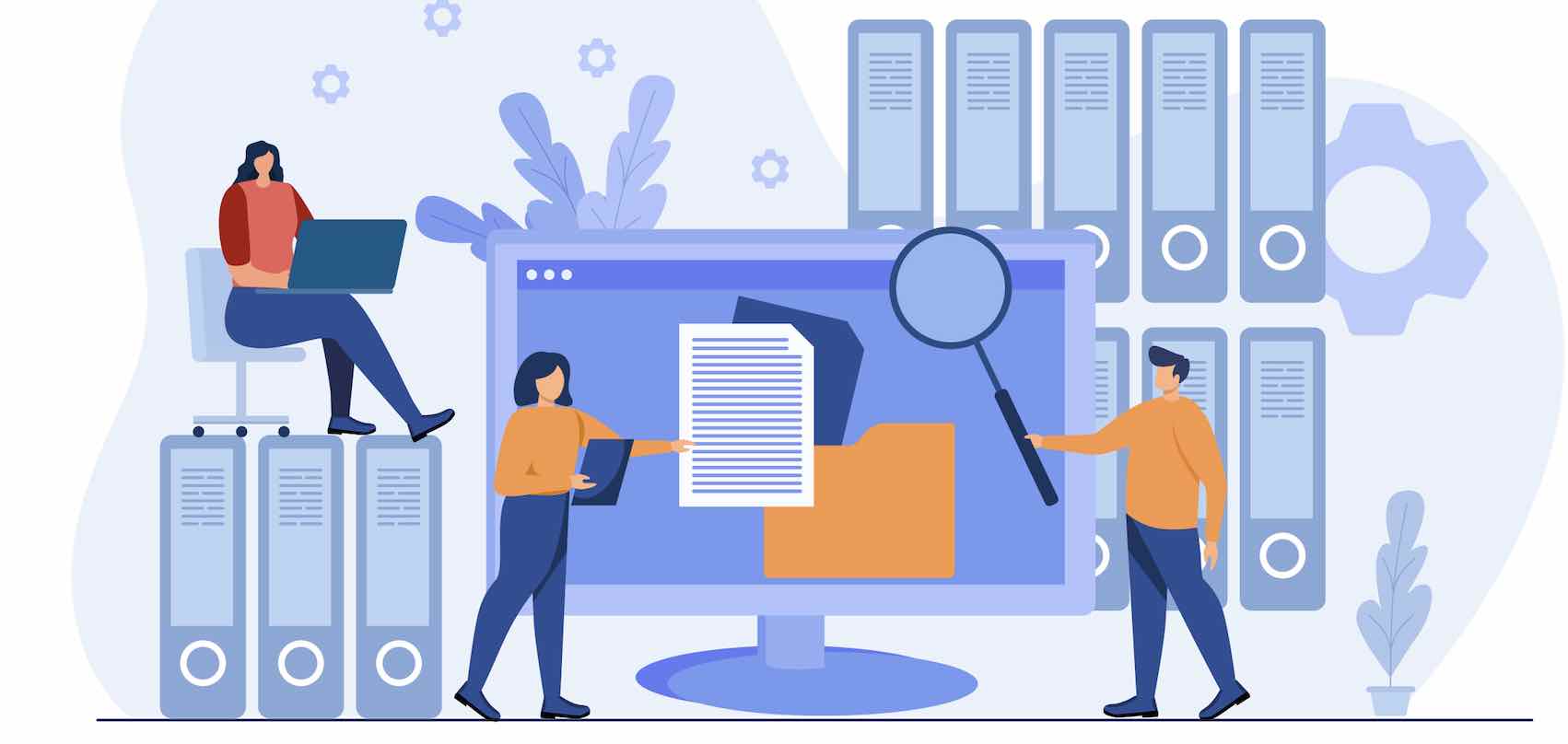
As you must be aware that createArrayOf() method from the Connection Interface will give you a java.sql.SQLFeatureNotSupportedException exception. One of the "not so ideal" ways to achieve the IN Clause in MySQL is to dynamically create the number of parameters as ? in your IN Clause.
import java.sql.Array;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class JDBCInClauseExample {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
//The elements for IN Clause
ArrayList<String> studentNames = new ArrayList<>();
studentNames.add("Harry");
studentNames.add("Ron");
//Create an array of same size
String[] arrayOfQuestionMarks = new String[studentNames.size()];
//Create a array of question marks ?
for(int i=0;i< studentNames.size();i++) {
arrayOfQuestionMarks[i] = "?";
}
//Create a string of question marks ?
String inClause = String.join(",", arrayOfQuestionMarks);
String url ="jdbc:mysql://localhost:3306/my_uat";
String userName="root";
String password ="root123";
String selectQuery ="Select * from students where student_name in ("+inClause+")";
Connection connection = DriverManager.getConnection(url,userName,password);
PreparedStatement preparedStatement = connection.prepareStatement(selectQuery);
for(int i=0;i< studentNames.size();i++) {
preparedStatement.setString(i+1, studentNames.get(i));
}
ResultSet resultSet = preparedStatement.executeQuery();
while(resultSet.next()) {
System.out.print(resultSet.getInt(1)+"\t");
System.out.print(resultSet.getString(2)+"\t");
System.out.print(resultSet.getString(3)+"\t");
System.out.print(resultSet.getString(4)+"\t");
System.out.println("");
}
}
}
Output
1 Harry 1997-01-03 00:00:00 London
2 Ron 1999-03-19 00:00:00 Paris
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Change Line Endings (Encoding Windows/Mac/Unix CR/LF/CRLF) Sublime Text - Sublime-Text
- How to uninstall GarageBand from Mac - MacOS
- Resolve System.IO.PathTooLongException [Sharepoint C# .Net] - SharePoint
- How to Install Windows Terminal Without the Store - Windows
- How to Set Up Dual Monitors on a Windows 11 PC - Windows-11
- How to add Back Button on Toolbar in Android [Tutorial] - Android
- Fix Generics: error unexpected type required: class found: type parameter - Java
- How to enable disable SharePoint Developer Dashboard for tracing troubleshooting - SharePoint