package org.code2care.generics;
public class Example<T,V> {
public static void main(String[] args) {
System.out.println("Generics Example!");
}
public void method() {
T t = new T();
V v = new V();
}
}
Compilation Error:
Code2care@Mac % javac Example.java
Example.java:10: error: unexpected type
T t = new T();
^
required: class
found: type parameter T
where T is a type-variable:
T extends Object declared in class Example
Example.java:11: error: unexpected type
V v = new V();
^
required: class
found: type parameter V
where V is a type-variable:
V extends Object declared in class Example
2 errors
This is a violation of the rules of dealing with Generic types in Java. You cannot create an instance of generic type parameters.
Hence the compilation error clearly states that it was expecting a class type but a type parameter was provided.
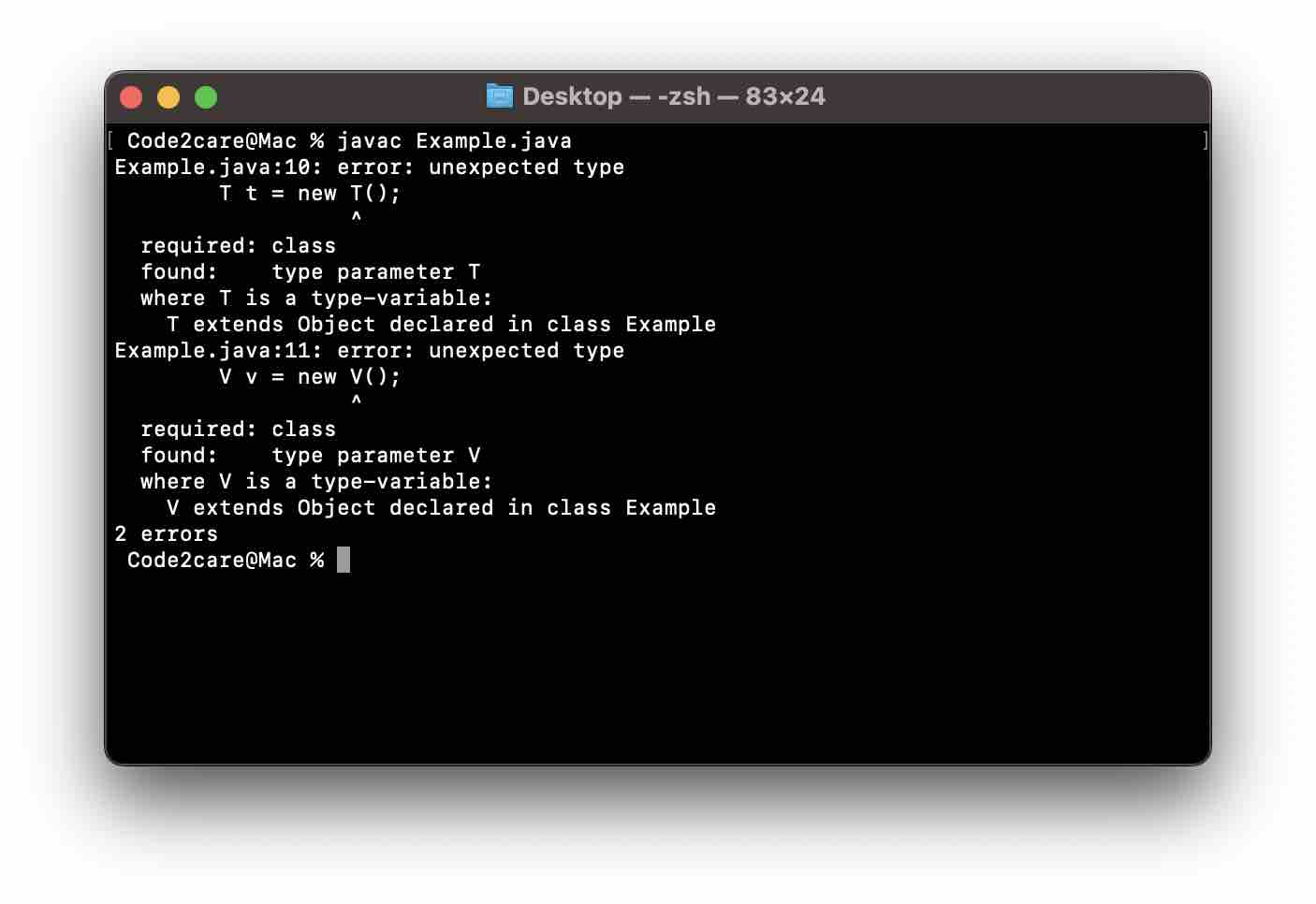
The ideal way would be to pass the type parameters as parameters to your method.
package org.code2care.generics;
public class Example<K,V> {
public static void main(String[] args) {
Example<String,Integer> example = new Example<>();
example.method("Sam",20);
}
public void method(K k, V v) {
System.out.println(k);
System.out.println(v);
}
}
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to Enable StandBy Mode on iPhone with iOS 17 - iOS
- How to URL Encode Data for a cURL Request Parameters - cURL
- Convert java.time.LocalTime to java.util.Date Object - Java
- Rounded Images in Bootstrap framework - Bootstrap
- Setting up Cloud feature with Notepad++ - NotepadPlusPlus
- List of 60 useful FTP Client Commands to access server - FTP
- Python: Replace the First Occurrence of String in a String - Python
- 34: Traverse a List in Reverse Order - 1000+ Python Programming - Python-Programs