In this Tutorial, we will take a look at how to do Batch Update using Java JDBC PreparedStatement,
Table: studentsCREATE TABLE `students` (
`student_id` int NOT NULL AUTO_INCREMENT,
`student_name` varchar(45) NOT NULL,
`student_dob` datetime NOT NULL,
`student_address` varchar(45) NOT NULL,
PRIMARY KEY (`student_id`);
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.time.LocalDate;
Java Code Example:
public class JDBCBatchUpdateExample {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
String url ="jdbc:mysql://localhost:3306/my_uat";
String userName="root";
String password ="root123";
String batchInsertQuery ="insert into students values(?,?,?,?)";
Connection connection = DriverManager.getConnection(url,userName,password);
PreparedStatement preparedStatement = connection.prepareStatement(batchInsertQuery);
preparedStatement.setObject(1, null);
preparedStatement.setObject(2, "Harry");
preparedStatement.setObject(3, LocalDate.of(1997, 01, 03));
preparedStatement.setObject(4, "London");
preparedStatement.addBatch();
preparedStatement.setObject(1, null);
preparedStatement.setObject(2, "Ron");
preparedStatement.setObject(3, LocalDate.of(1999, 03, 19));
preparedStatement.setObject(4, "Paris");
preparedStatement.addBatch();
preparedStatement.setObject(1, null);
preparedStatement.setObject(2, "Hermione");
preparedStatement.setObject(3, LocalDate.of(1995, 02, 14));
preparedStatement.setObject(4, "Paris");
preparedStatement.addBatch();
int[] resultSet = preparedStatement.executeBatch();
for(int result: resultSet) {
System.out.println("Result: " +result);
}
}
}
Make sure to add the records to the batch or else nothing will get executed.
Also a common mistake if you do preparedstatement.addBatch() at the end of each record only the last record will get written.
Result: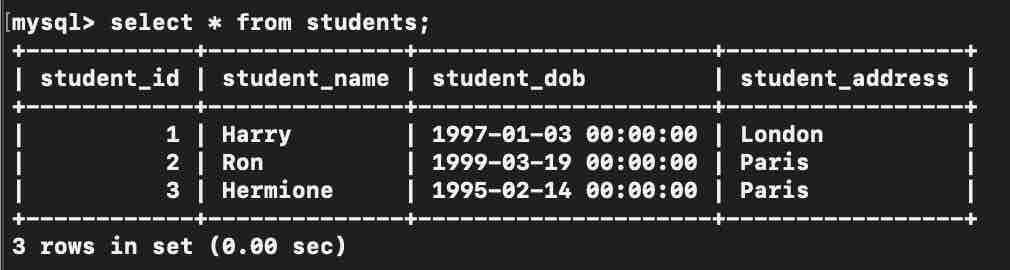
Java JDBC Batch Update Example
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Where does Notepad++ save temp files? - NotepadPlusPlus
- Drag drop files here option missing for SharePoint document library - SharePoint
- How to install Python Specific version (3.8, 3.9 or 3.10) using Brew - Python
- PHP Warning: Cannot modify header information - headers already sent - PHP
- Java: Convert a Text file into Array of Bytes Example - Java
- Whats new in Python 3.10 Pre-release - Python
- 5 Reasons Why Jupyter Notebook is Not Opening and Solutions - Python
- How to Pretty Print cURL JSON Output in Terminal - cURL