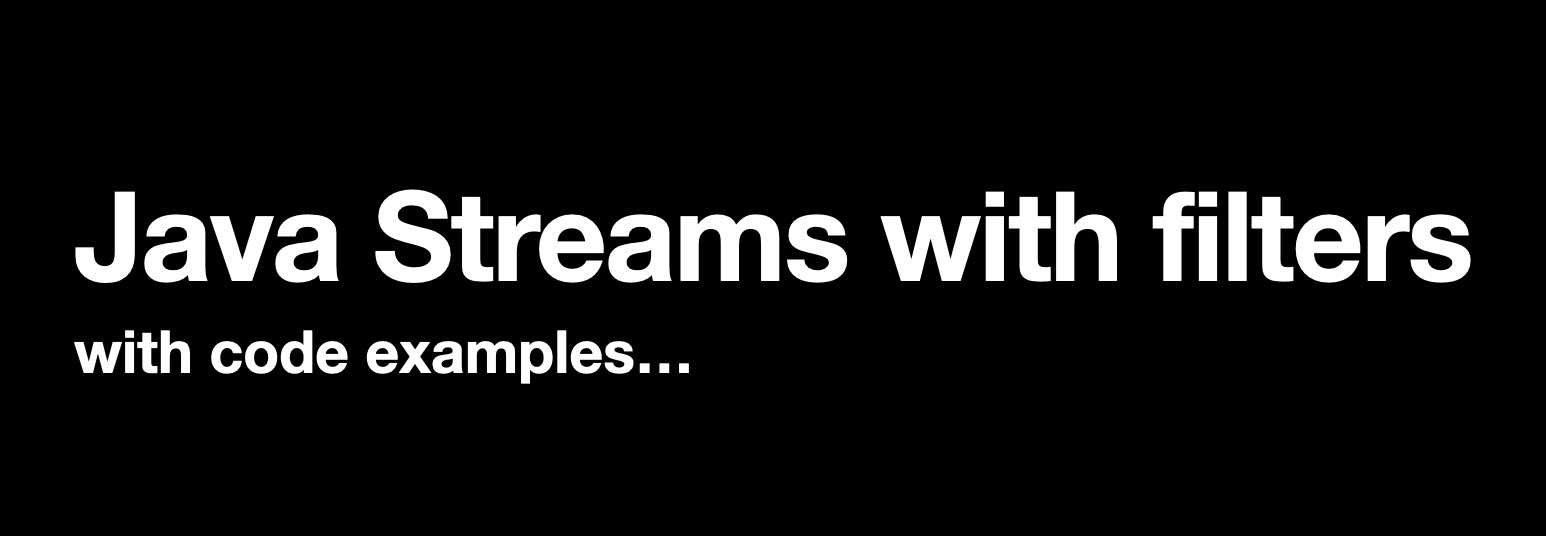
Streams is one of the most powerful feature that were added to Java core libraries in the Java 8 version. In this tutorial, we will take a look at various examples to make use of filter() with Streams and related methods to find a solution to a use-case,
Filter method signature:Stream<T> filter(Predicate<? super T> predicate);
Example 1: Use Stream filter to find even and off numbers
In this example, we have made use of filter() with collect() method to collect result as a List.
package org.code2care.java;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class JavaStreamFiltersExamples {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>();
numbers.addAll(Arrays.asList(1,2,3,4,5,6,7,8,9,10));
List<Integer> evenNumbers = numbers.stream().filter(number -> number %2 ==0).collect(Collectors.toList());
List<Integer> oddNumbers = numbers.stream().filter(number -> number %2 !=0).collect(Collectors.toList());
System.out.println("Even Numbers" + evenNumbers);
System.out.println("Odd Numbers" + oddNumbers);
}
}
Output:
Even Numbers[2, 4, 6, 8, 10]
Odd Numbers[1, 3, 5, 7, 9]
Example 2: Use Stream filter to all names starts with letter S
In this example, have make use of filter() with forEach() to print out the results on console,
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.addAll(Arrays.asList("Sam", "Chris", "Mike", "Eric", "Jose", "Samuel", "Scott"));
System.out.println("All Names starting with Letter S in list " + names + " are: ");
names.stream().filter(name -> name.startsWith("S")).forEach(System.out::println);
}
Output:
All Names starting with Letter S in the list [Sam, Chris, Mike, Eric, Jose, Samuel, Scott] are:
Sam
Samuel
Scott
Example 3: Use Stream filter to get the sum of all numbers > 3 in the list
In this example, have made use of filter() to filter out numbers > 3 and them used mapOfInt() and finally sum() to get the sum,
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(5,2,6,8,9,1);
int sum = numbers.stream().filter(number -> number > 3).mapToInt(i -> i).sum();
System.out.println("Sum of numbers > 3 is "+ sum);
}
Output:
Sum of numbers > 3 is 28
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Fix RabbitMQ: AuthenticationFailureException: ACCESS_REFUSED - Java
- [Tutorial] How to Customize Notepad++ Toolbar - NotepadPlusPlus
- SharePoint - The URL is invalid. It may refer to a nonexistent file or folder, or refer to a valid file or folder that is not in the current Web. - SharePoint
- How to create alias in macOS - MacOS
- How to install Packages in Sublime Text Editor - Sublime-Text
- Docker Desktop needs privileged access macOS - MacOS
- Building library Gradle Project Info: Downloading services.gradle.org - Android-Studio
- Convert String to LocalDate (using Java 8 Date Time API) - Java