In this tutorial we will take a look at how to convert a Java Collection List to a Map, there are multiple ways in which you can do so, we will take a look at using Streams,
Example: Student.javaLet us first create our Class called Student,
public class Student {
int studentId;
String studentName;
int studentAge;
public Student(int studentId, String studentName, int studentAge) {
this.studentId = studentId;
this.studentName = studentName;
this.studentAge = studentAge;
}
public int getStudentId() {
return studentId;
}
public void setStudentId(int studentId) {
this.studentId = studentId;
}
public String getStudentName() {
return studentName;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
public int getStudentAge() {
return studentAge;
}
public void setStudentAge(int studentAge) {
this.studentAge = studentAge;
}
@Override
public String toString() {
return "Student{" +
"studentId=" + studentId +
", studentName='" + studentName + '\'' +
", studentAge=" + studentAge +
'}';
}
}
ListToMapExample.java
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
/**
* Java 8 Example to Convert a
* Collection List Object into
* a Map
*
* Author: Code2care.org
* Date: 03-Apr-2022
* Version: 1.0
*
*/
public class ListToMapExample {
public static void main(String[] args) {
//Step 1: Create some Student Objects
Student student1 = new Student(1, "Sam", 19);
Student student2 = new Student(2, "Mike", 18);
Student student3 = new Student(3, "Alex", 21);
//Step 2: Add them to a List
List studentList = new ArrayList<>();
studentList.add(student1);
studentList.add(student2);
studentList.add(student3);
//Step 3: Converting Student List to a Map using Stream
Map<Integer, Student> studentMap = (Map<Integer, Student>) studentList.stream()
.collect(Collectors.toMap(Student::getStudentId, Function.identity()));
//Step 4: Let's print the results
for (Map.Entry<Integer, Student> entry : studentMap.entrySet()) {
System.out.println(entry.getKey() + ":" + entry.getValue());
}
}
}
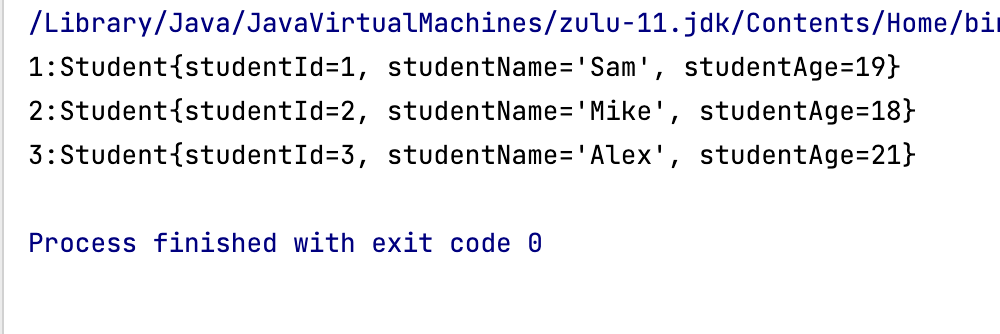
Java 8 - Convert List to Map Example Output
Note that the key that you identify from the List for the Map should be unique or else you will get java.lang.IllegalStateException: Duplicate key
Exception in thread "main" java.lang.IllegalStateException: Duplicate key 2 (attempted merging values Student{studentId=2, studentName='Mike', studentAge=18} and Student{studentId=2, studentName='Mike', studentAge=18})
at java.base/java.util.stream.Collectors.duplicateKeyException(Collectors.java:133)
at java.base/java.util.stream.Collectors.lambda$uniqKeysMapAccumulator$1(Collectors.java:180)
at java.base/java.util.stream.ReduceOps$3ReducingSink.accept(ReduceOps.java:169)
at java.base/java.util.ArrayList$ArrayListSpliterator.forEachRemaining(ArrayList.java:1655)
at java.base/java.util.stream.AbstractPipeline.copyInto(AbstractPipeline.java:484)
at java.base/java.util.stream.AbstractPipeline.wrapAndCopyInto(AbstractPipeline.java:474)
at java.base/java.util.stream.ReduceOps$ReduceOp.evaluateSequential(ReduceOps.java:913)
at java.base/java.util.stream.AbstractPipeline.evaluate(AbstractPipeline.java:234)
at java.base/java.util.stream.ReferencePipeline.collect(ReferencePipeline.java:578)
at ListToMapExample.main(ListToMapExample.java:35)
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Fix RabbitMQ: AuthenticationFailureException: ACCESS_REFUSED - Java
- [Tutorial] How to Customize Notepad++ Toolbar - NotepadPlusPlus
- SharePoint - The URL is invalid. It may refer to a nonexistent file or folder, or refer to a valid file or folder that is not in the current Web. - SharePoint
- How to create alias in macOS - MacOS
- How to install Packages in Sublime Text Editor - Sublime-Text
- Docker Desktop needs privileged access macOS - MacOS
- Building library Gradle Project Info: Downloading services.gradle.org - Android-Studio
- Convert String to LocalDate (using Java 8 Date Time API) - Java