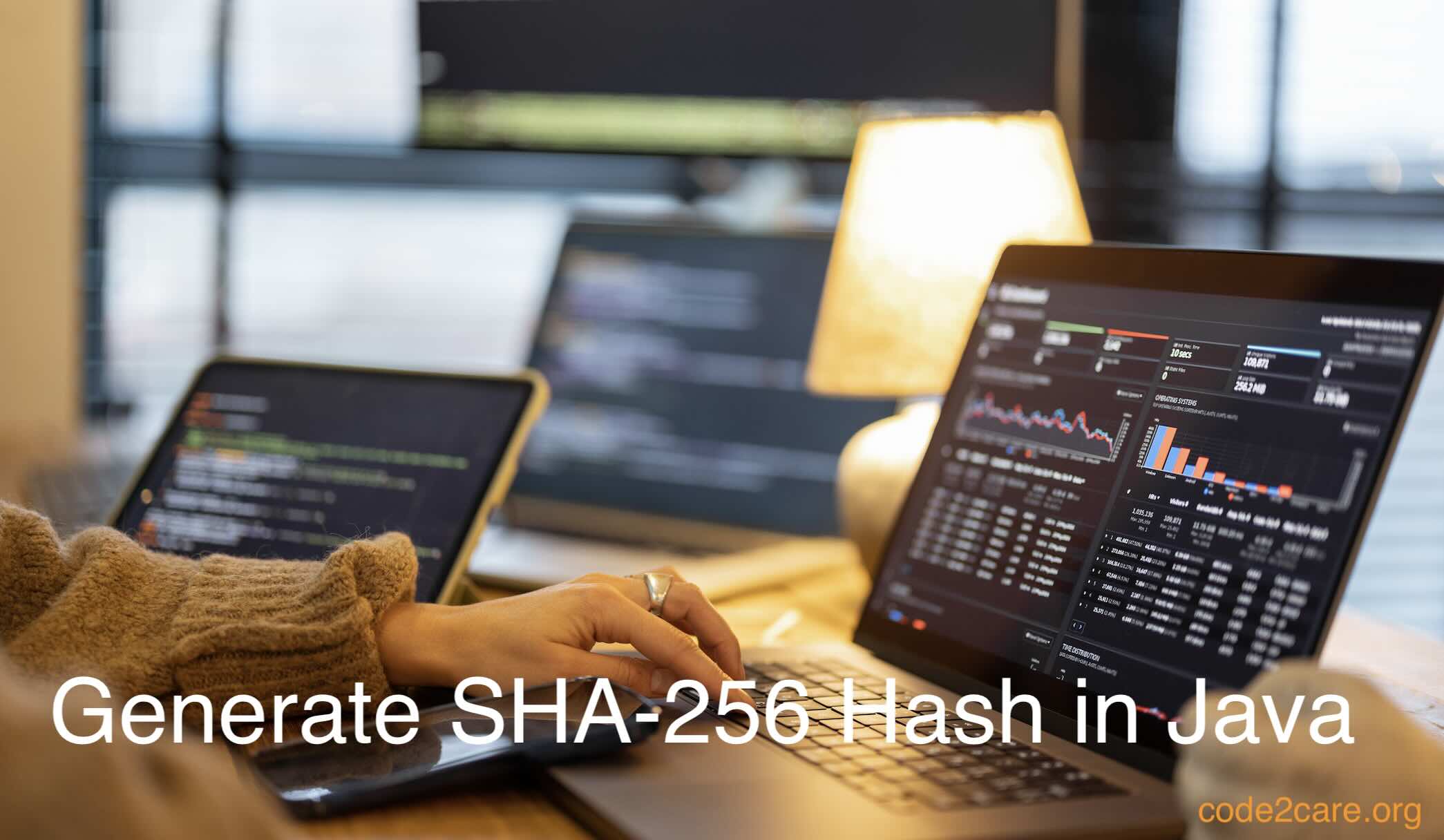
The SHA-256 stands for "Secure Hash Algorithm 256-bit" is a cryptographic "hash function" that takes input data and produces a fixed-size, 256-bit (32-byte) hash value.
SHA-256 is widely used for data integrity, digital signatures, and security applications.
Let's take a look at a few examples of how to generate a SHA-256 in Java.
Example:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class JavaSHA256HashExample {
public static void main(String[] args) throws NoSuchAlgorithmException {
String algorithm = "SHA-256";
String inputMessage = "Code2care";
MessageDigest sha256MessageDigest = MessageDigest.getInstance(algorithm);
byte[] hashBytes = sha256MessageDigest.digest(inputMessage.getBytes());
StringBuilder hexString = new StringBuilder();
for (byte b : hashBytes) {
hexString.append(String.format("%02x", b));
}
System.out.println("Message:" + inputMessage);
System.out.println("SHA-256 Hash: " + hexString.toString());
}
}
Output:
Message:Code2care
SHA-256 Hash: 96111e521eec4620d6da2006d39891a7c8099dba6861baca73a11037a9bf63bb
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to get query string in JavaScript HTML location.search - JavaScript
- PowerShell: The term Connect-AzureAD is not recognized as the name of a cmdlet - Powershell
- How to Run PowerShell Script as a Windows Scheduler Task - Powershell
- Android Eclipse This version of the rendering library is more recent than your version of ADT plug-in. Please update ADT plug-in - Android
- How to determine Gradle Version in Android Studio - Android-Studio
- Visual Studio Code available for Apple Mac ARM64 build for native M1 processor support - Microsoft
- How to restore closed file in Notepad++ - NotepadPlusPlus
- Unable to connect to the Internet : Google Chrome - Chrome