To create Date in yyyy-MM-dd format we will make use of,
the LocalDate from java.time package,
the DateTimeFormatter class from the java.time.format package.
Let's take a look at a few examples,
Example 1: From Current Date
package org.code2care.examples;
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class LocalDateFormattingyyyyMMddExample {
private static final String YYYY_MM_DD = "yyyy-MM-dd";
public static void main(String[] args) {
LocalDate currentDate = LocalDate.now();
DateTimeFormatter dataTimeFormatter = DateTimeFormatter.ofPattern(YYYY_MM_DD);
String formattedDate = currentDate.format(dataTimeFormatter);
System.out.println("Date Today: " + formattedDate);
}
}
Output: 2023-07-03
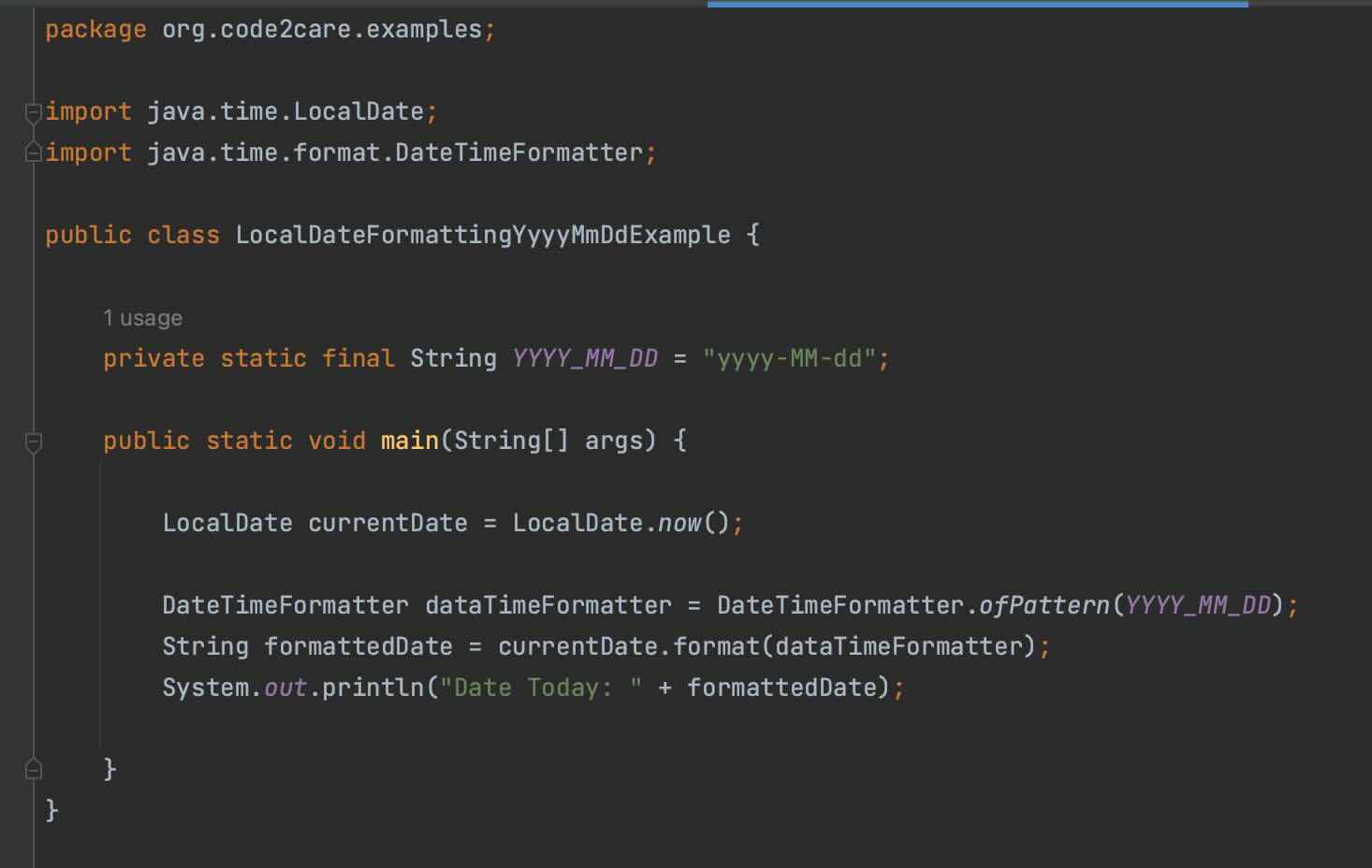
Example 2: Formatting an existing Date as String
package org.code2care.examples;
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class LocalDateFormattingyyyyMMddExample2 {
private static final String YYYY_MM_DD = "yyyy-MM-dd";
public static void main(String[] args) {
String dateString = "2023-07-03";
LocalDate localDate = LocalDate.parse(dateString);
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(YYYY_MM_DD);
String formattedDate = localDate.format(formatter);
System.out.println("Date: " + formattedDate);
}
}
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- tkinter - Hello World! Program - Python
- How to Auto Fill Down in Excel for Mac - MacOS
- 573 List of reserved keywords in AWS DynamoDB - AWS
- How to Provide Full Disk Access to App on Mac - MacOS
- How to write hello world different languages syntax - HowTos
- How to Transpose a Square Matrix - Java Program - Java
- Java -Day of the week using Java 8 DayOfWeek Enum - Java
- Keyboard shortcuts to format Source code in Microsoft Visual Studio Code IDE - Microsoft