We will cover examples for both older Java Date class as well as the preferred new Java Date Time API LocalDate class.
Example: Java Date Class (before Java 8)
package org.code2care.examples;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Example {
public static void main(String... args) {
Date date = new Date();
SimpleDateFormat sdfForYear = new SimpleDateFormat("yyyy");
String year = sdfForYear.format(date);
System.out.println("Year from date: " + date + " is " + year);
}
}
Output:
Example: Using LocalDate (Java 8 or above)
package org.code2care.examples;
import java.time.LocalDate;
public class Example {
public static void main(String... args) {
LocalDate localDate = LocalDate.now();
int year = localDate.getYear();
System.out.println("Year from date: " + localDate + " is " + year);
}
}
Output:
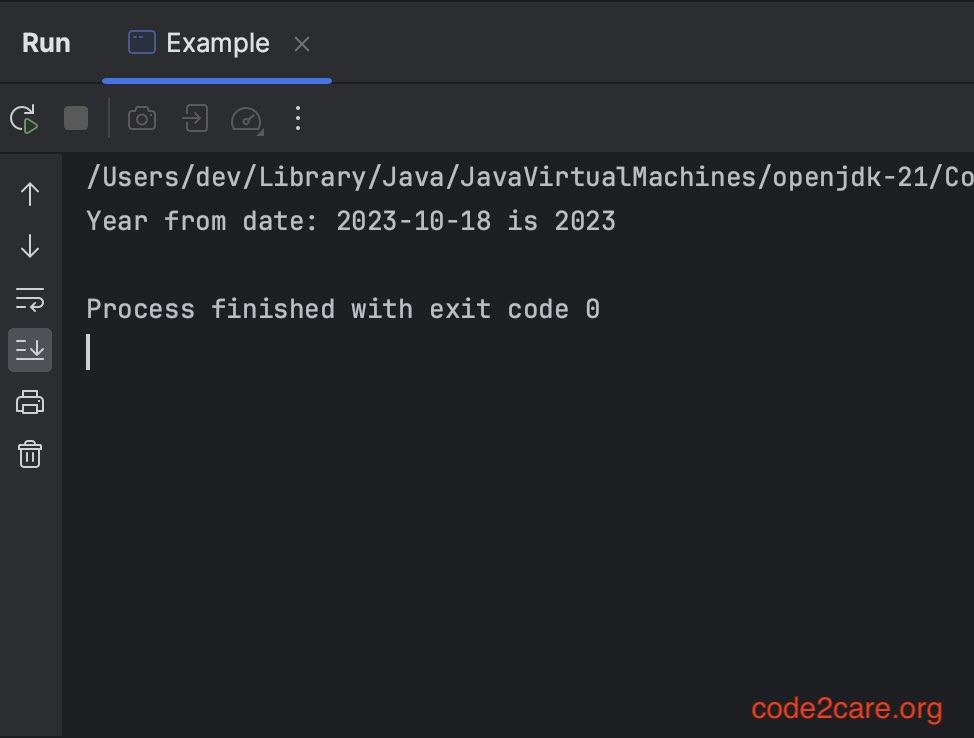
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- [fix] openssl No such file or directory error C++ - Ubuntu
- [Solution] macOS could not be installed on your computer OSInstall.mpkg appears to be missing or damaged - MacOS
- macOS: Change Weather App Temperature unit from Fahrenheit to Degree - MacOS
- Adding Sub Headings to Bootstrap Header tags - Html
- Facebook Graph API Unavailable - Facebook
- How to run bash command in background - Bash
- Installing Android Studio Dolphin on Mac with Apple (M1/M2) Chip - Android-Studio
- How to Disable EditText Keyboard Android App - Android