You can get the current date using LocalDate in Java by using the static now() method of the LocalDate class.
Let's see a few examples:
import java.time.LocalDate;
public class DateExample {
public static void main(String... args) {
LocalDate currentDate = LocalDate.now();
System.out.println("The Current Date Is: " + currentDate);
}
}
We have made use of the LocalDate class from the java.time package, which was introduced in Java 8 as a part of the new Date Time API.
If you want to know the date of a specific city, you will need to make use of the ZoneId class.
Example:
import java.time.LocalDate;
import java.time.ZoneId;
LocalDate currentDateInNewYork = LocalDate.now(ZoneId.of("America/New_York"));
LocalDate currentDateInLondon = LocalDate.now(ZoneId.of("Europe/London"));
System.out.println("Current Date in New York: " + currentDateInNewYork);
System.out.println("Current Date in London: " + currentDateInNewYork);
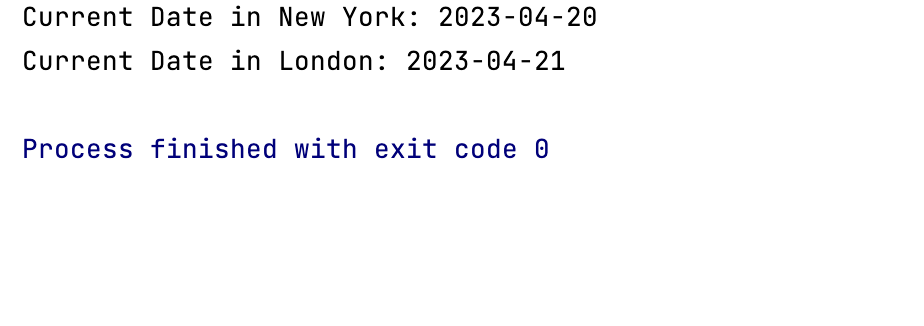
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to add password to pdf file for opening, editing, printing, copying - HowTos
- How to call a SQL StoredProcedure from Hibernate - Java
- Save current timestamp in MySQL using PHP mysqi binding - PHP
- Merge-SPlogfile PowerShell - SharePoint Correlation ID error - SharePoint
- How to change user image icon macOS Big Sur - MacOS
- How to Sync Microsoft Teams Calendar with Mac Calendar - Microsoft
- Difference between using Scanner Class and String args for user input in Java - Java
- Mac - Steam Needs to Be Online to Update. Please confirm your network connection and try again error - News