In order to get the Date in Java for a specific TimeZone, you can make use of the LocalDate class from the Date-Time API and pass in the ZoneId for the timezone in the static now method.
Example:
import java.time.LocalDate;
import java.time.ZoneId;
public class GetDateTimeZoned {
public static void main(String[] args) {
LocalDate dateInChicago = LocalDate.now(ZoneId.of("America/Chicago"));
LocalDate dateInMumbai = LocalDate.now(ZoneId.of("Asia/Kolkata"));
LocalDate dateInTokyo = LocalDate.now(ZoneId.of("Asia/Tokyo"));
LocalDate dateInLondon = LocalDate.now(ZoneId.of("Europe/London"));
System.out.println("Date in Chicago " + dateInChicago);
System.out.println("Date in Mumbai: " + dateInMumbai);
System.out.println("Date in Tokyo: " + dateInTokyo);
System.out.println("Date in London: " + dateInLondon);
}
}
Output:
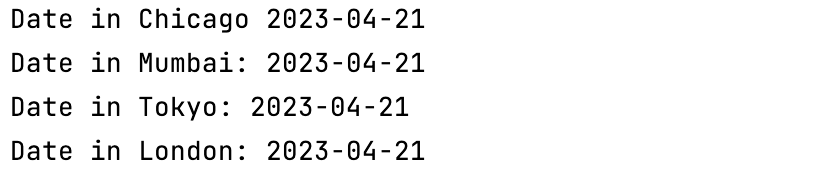
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to Vertically Center Align Text in a Div using CSS Code Example - CSS
- Python: Split a String into a Dictionary - Python
- Convert JSON to Gson with type as ArrayList - Java
- No CPU ABI system image available for this target Error Android Virtual Device - Android
- cURL: Show Request and Response Headers - cURL
- View in File Explorer option missing in SharePoint Online Edge browser - SharePoint
- Failed to install Android.apk on device 'emulator-5554': timeout - Android-Studio
- Mac: How to quit Jupyter Notebook from Terminal - MacOS