package org.code2care.generics;
public class Example<K,V> {
public void method() {
K k = new K();
V v = new V();
}
}
If you are working inside a Java IDE like IntelliJ, you would see a compliation error in the above code that reads "Type parameter 'K' cannot be instantiated directly". This is because you cannot create an instance of a generic type parameter directly, it has to be a class.
To better understand this, you can refer to the official Java Generics Tutorial: Restrictions on Generics
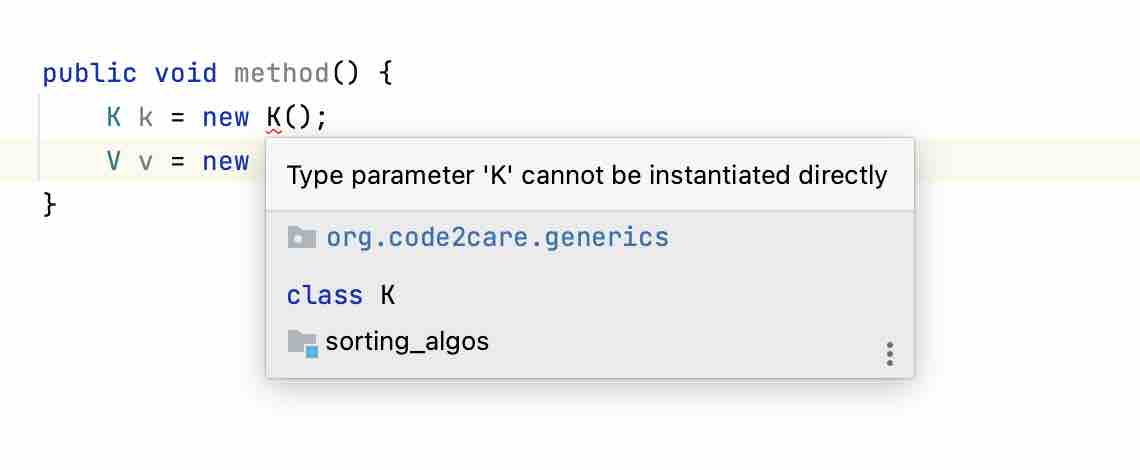
Fix:
One workaround would be to create an instance of an Object class which is the parent of all classes in Java and cast it to your generic type.
public void method() {
K k = (K) new Object();
V v = (V) new Object();
}
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Fix - Microsoft Teams Error Code - 80090016 - Teams
- Create Nested Directories using mkdir Command - Linux
- How to Install npm using Mac Terminal - MacOS
- Fix: Cannot contact reCAPTCHA. Check your connection and try again. - Google
- pwd Command - Print Working Directory - Linux
- Install specific JRE on Ubuntu using apt Command - Ubuntu
- Get the total size and number of objects of a AWS S3 bucket and folders - AWS
- Delete file using PHP code : unlink() - PHP