In order to compress files and folders as a tar.gz file in Java we can make use of the Apache Commons Compress dependency.
Maven Dependency:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-compress</artifactId>
<version>1.23.0</version>
</dependency>
Gradle Dependency:
implementation group: 'org.apache.commons', name: 'commons-compress', version: '1.23.0'
Code Example:
import org.apache.commons.compress.archivers.tar.TarArchiveEntry;
import org.apache.commons.compress.archivers.tar.TarArchiveOutputStream;
import org.apache.commons.compress.compressors.gzip.GzipCompressorOutputStream;
import java.io.*;
import java.util.zip.Deflater;
public class TarGzCreatorWithJava {
public static void main(String[] args) {
String sourceDirPath = "/data";
String tarGzFilePath = "/data/data.tar.gz";
try {
var fileOutputStream = new FileOutputStream(tarGzFilePath);
var gzipCompressorOutputStream = new GzipCompressorOutputStream(fileOutputStream);
var tarArchiveOutputStream = new TarArchiveOutputStream(gzipCompressorOutputStream);
tarArchiveOutputStream.setBigNumberMode(TarArchiveOutputStream.BIGNUMBER_STAR);
tarArchiveOutputStream.setLongFileMode(TarArchiveOutputStream.LONGFILE_GNU);
File sourceDir = new File(sourceDirPath);
addFilesToTar(sourceDir, tarOut, "");
tarOut.finish();
tarOut.close();
System.out.println("File created.. : " + tarGzFilePath);
} catch (IOException e) {
e.printStackTrace();
}
}
private static void addFilesToTar(File source, TarArchiveOutputStream tarOut, String parentDir) throws IOException {
File[] files = source.listFiles();
if (files != null) {
for (File file : files) {
if (file.isDirectory()) {
addFilesToTar(file, tarOut, parentDir + file.getName() + "/");
} else {
TarArchiveEntry entry = new TarArchiveEntry(parentDir + file.getName());
entry.setSize(file.length());
tarOut.putArchiveEntry(entry);
try (FileInputStream fis = new FileInputStream(file)) {
byte[] buffer = new byte[1024];
int read;
while ((read = fis.read(buffer)) != -1) {
tarOut.write(buffer, 0, read);
}
}
tarOut.closeArchiveEntry();
}
}
}
}
}
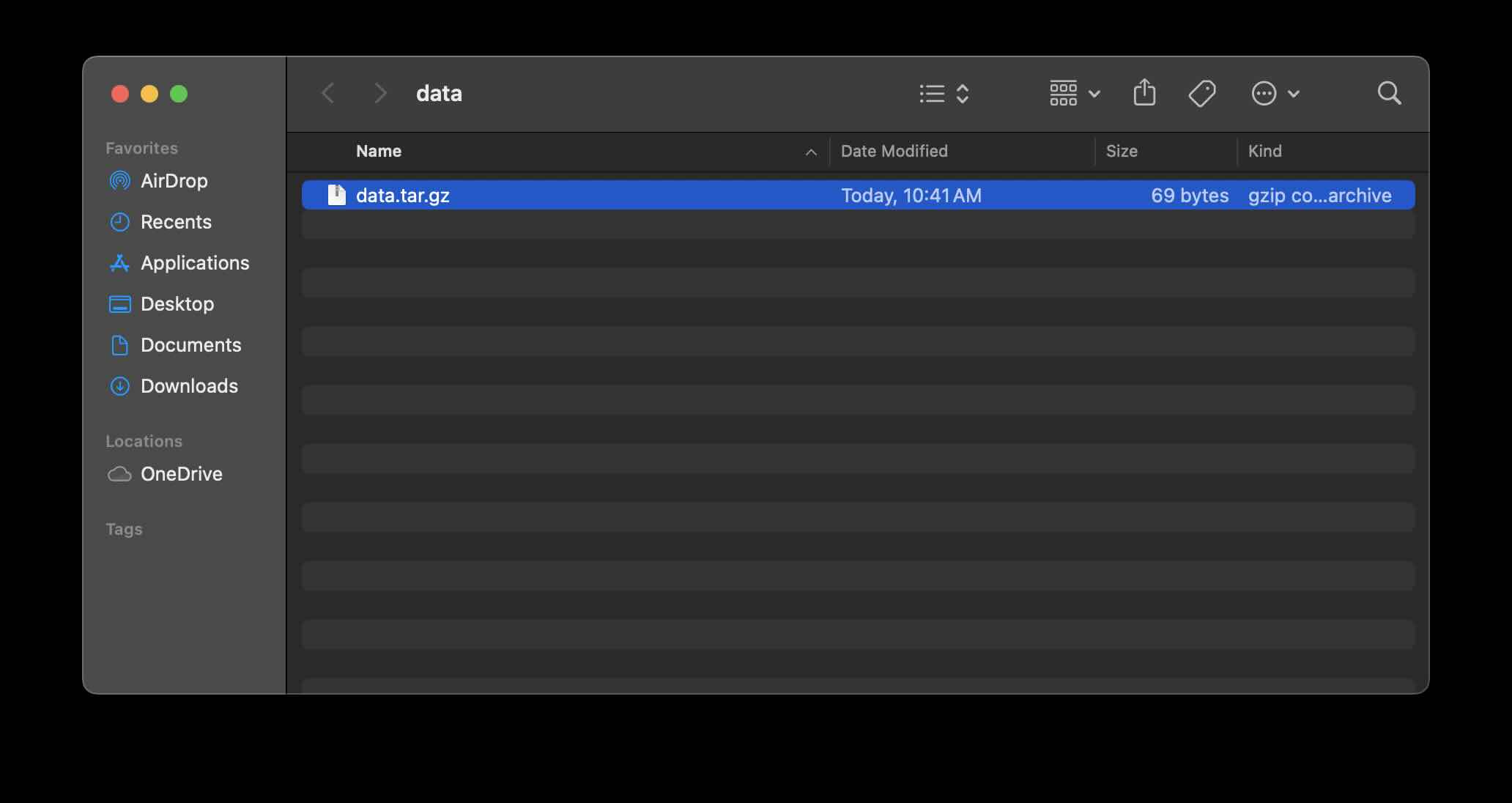
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to know your iPhone Model Name - iOS
- Notepad++ : Cannot load 64 or 32bit plugin Error. - NotepadPlusPlus
- Installation error: INSTALL_PARSE_FAILED_MANIFEST_MALFORMED - Android
- Why I see Download pre-built shared indexes in IntelliJ - HowTos
- Android: programmatically turn Bluetooth on or off using Java code - Android
- How to show or hide columns in SharePoint Online List Library from - SharePoint
- Git Revision Questions Before the Interview - Git
- The Motivation Behind Generics in Java Programming - Java