In Java, the Stream class was introduced as a part of the Java 8 Streams API that provides a functional way of processing collections of objects, i.e. a sequence of elements that can be processed in a functional manner.
With the help of the Stream class, you can perform various operations on collections like filtering, mapping, sorting, reducing, and more, without modifying the original collection.
Converting List to Set using Java 8 Stream API
Let's see the steps,
Step 1: We create a List of Strings (can be any other data type)
Step 2: We make use of the stream() to convert List to Stream.
Step 3: Finally, we make use of the collect() method to collect the Stream as set.
Code Example
package org.example;
import java.util.*;
import java.util.stream.Collectors;
/**
*
* Code Example in Java 8 to convert
* List to Set using Stream API
*
*
*/
public class ListToSet {
public static void main(String[] args) {
List<String> citiesList = new ArrayList<>();
citiesList.add("NYC");
citiesList.add("Chicago");
citiesList.add("Austin");
citiesList.add("Houston");
citiesList.add("Ohio");
citiesList.add("NYC"); //Duplicates
citiesList.add("Chicago"); //Duplicates
System.out.println("List Elements: " + citiesList);
//Map to List
Set<String> citiesSet = citiesList
.stream() //Step 1: Convert Set to Stream
.collect(Collectors.toSet()); // Step 2: Collect Stream as Set
System.out.println("Set Elements: " + citiesSet);
}
}
Output:
List Elements: [NYC, Chicago, Austin, Huston, Ohio, NYC, Chicago]
Set Elements: [Chicago, NYC, Ohio, Austin, Huston]
As you may see in the output, the set does not contain duplicate records.
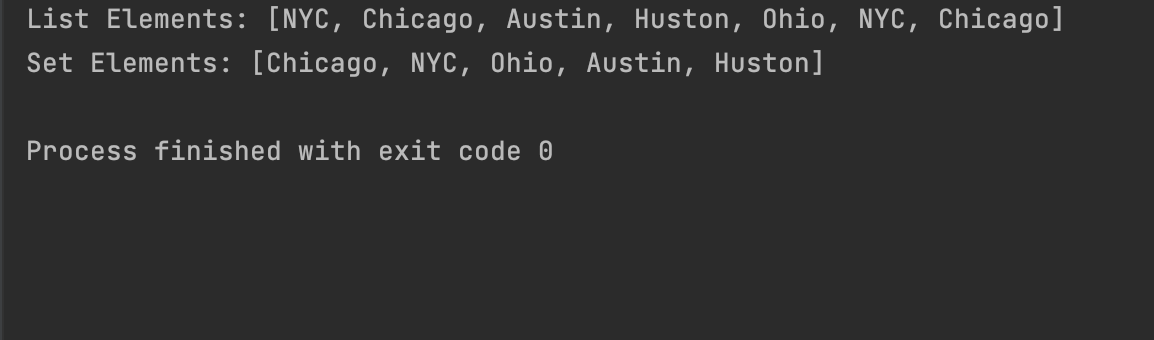
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- How to get Mac Computer Name using Terminal - MacOS
- Center align text in TextView Android Programming - Android
- Java: Reference List of Time Zones and GMT/UTC Offset - Java
- Java TLSv1.3 protocol code example using SSLSocket - Java
- Deep Dive: Java Object Class from java.lang Package - Java
- How to disable SharePoint subsite creation option for owners - SharePoint
- SharePoint Managed Metadata Hidden Taxonomy List - TaxonomyHiddenList - SharePoint
- Grep Alternative for Windows CMD or PowerShell: findstr - Windows