Let's see step by step how to make use of the java.io.BufferedWriter class to write to a file in Java.
- First let's create a String that holds data that we need to write to a file.
String data = "Some data to write to a file";
- Next, let's say we want to write to a file named data.txt
String fileName = "data.txt";
- Next we need to create an object of java.io.FileWriter and pass in the fileName and a boolean flag to say if we want text to be appended to the file or not.
FileWriter fileWriter = new FileWriter(fileName, true);
- Now we can make use of the object of java.io.BufferedWriter and pass the FileWriter object to its constructor. BufferedWriter will create a buffered character-output stream which is a file in our case.
- Next, make use of the write() method from BufferedWriter to write the String data to the file.
- Finally make sure to close the stream using the close() method.
Complete Code:
package org.code2care.example;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
/**
* <pre>
* Example: Write a file using BufferedWriter
*
* Author: Code2care.org
* Version: v1.0
* Date: 5th July 2023
* </pre>
*/
public class WriteFileUsingBufferedWriterExample {
public static void main(String[] args) {
String data = "Some data to write to a file";
String fileName = "data.txt";
try (FileWriter fileWriter = new FileWriter(fileName, true);
BufferedWriter bufferedWriter = new BufferedWriter(fileWriter)) {
bufferedWriter.write(data);
} catch (IOException ioException) {
System.out.println("Error occurred writing the file!");
}
}
}
Output:
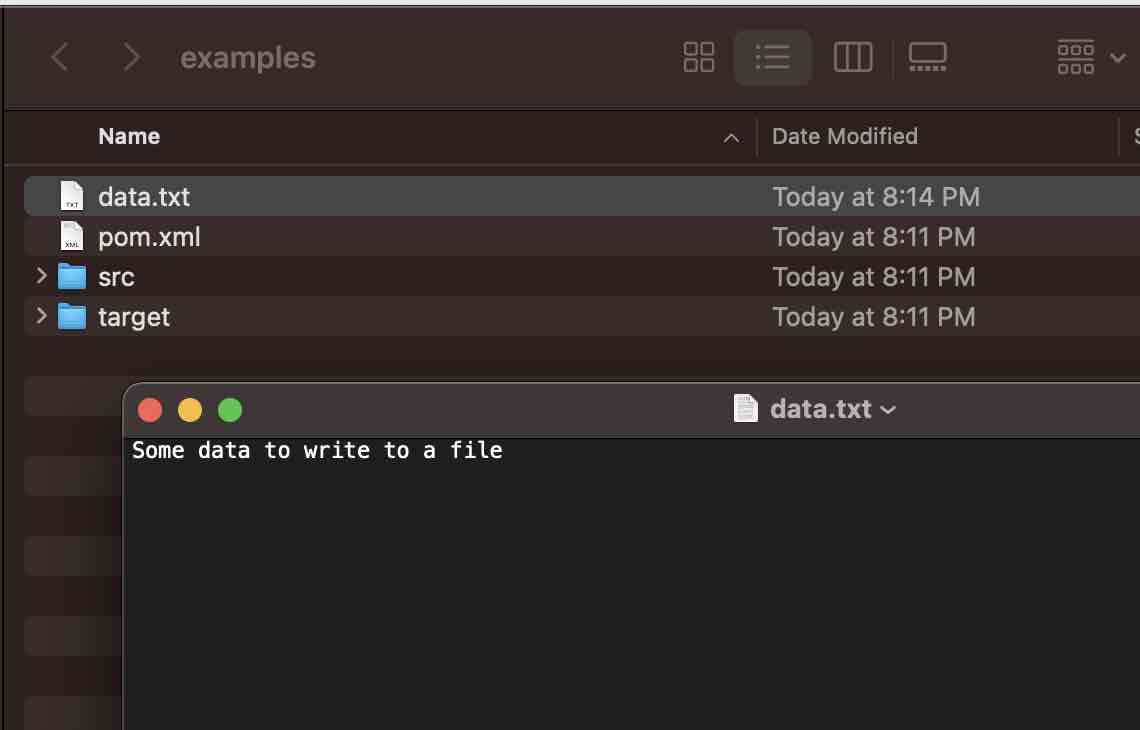
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to install Packages in Sublime Text Editor - Sublime-Text
- How to serialize-deserialize an object in java - Java
- Java 8 Predicate Functional Interface isEqual() Method Example - Java
- [Error] zsh: command not found: mvn - HowTos
- Skip Test Maven while creating package command - Java
- Open New tab using keyboard shortcut in Mac Terminal - Mac-OS-X
- How to Setup Microsoft OneDrive on Mac Sonoma 14 - Microsoft
- Working with Multiple Files in Sessions in Notepad++ - NotepadPlusPlus