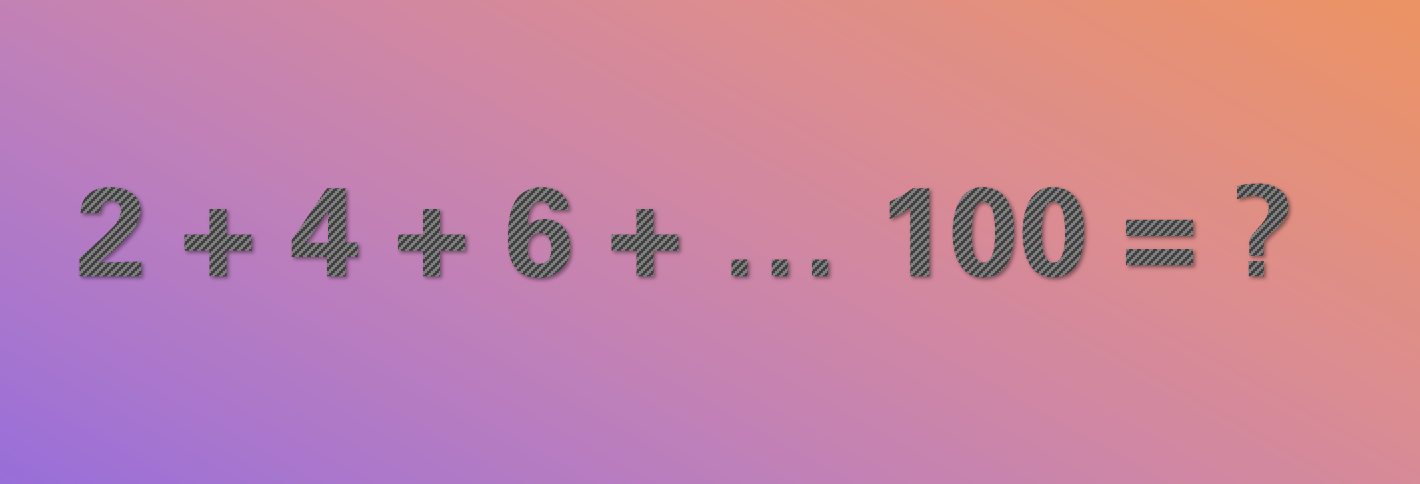
Brainstroming the Problem
We need to find the sum of all even numbers between 1 and 100.
We can do this by iterating through all the numbers from 1 to 100, and adding the even numbers to a variable that stores the sum.
Pseudo code:
1. Initialize a variable to store the sum.
2. Iterate through all the numbers from 1 to 100.
3. If a number is even, add it to the sum variable.
4. After iterating through all numbers, return the sum.
Python Code
sum_of_even_from_1_to_100 = 0
for i in range(1, 101):
if i % 2 == 0:
sum_of_even_from_1_to_100 += i
print(f"Sum of all even numbers from 1 to 100 is: {sum_of_even_from_1_to_100}")
Output:
Sum of all even numbers from 1 to 100 is: 2550
Reference to Python documentation for methods used:
- range() function: https://docs.python.org/3/library/stdtypes.html#range
- % operator: https://docs.python.org/3/reference/expressions.html#binary-arithmetic-operations
- += operator: https://docs.python.org/3/reference/simple_stmts.html#augmented-assignment-statements
- print() function: https://docs.python.org/3/library/functions.html#print
- f-string: https://docs.python.org/3/reference/lexical_analysis.html#f-strings
Related Questions
- Find the sum of all even numbers between 1 and 100 in Python.
- Write a Python program to calculate the sum of even numbers from 1 to 100.
- Given a range of numbers from 1 to 100, find the sum of all even numbers using Python.
- In Python, write a program to add all even numbers between 1 and 100.
- How to use Python to find the sum of even numbers in the range of 1 to 100?
- Write a Python script to compute the sum of all even numbers between 1 and 100.
- Calculate the sum of all even numbers between 1 and 100 using Python.
- Using Python, find the total sum of all even numbers between 1 and 100.
- In Python, write a program to find the sum of all even numbers within the range of 1 to 100.
- What is the sum of all even numbers from 1 to 100 when calculated using Python?
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python-Programs,
- Program 5: Find Sum of Two Integer Numbers - 1000+ Python Programs
- 34: Traverse a List in Reverse Order - 1000+ Python Programming
- 22: Send Yahoo! Email using smtplib - SMTP protocol client using Python Program
- 35: Python Program to find the System Hostname
- 27: Measure Elapsed Time for a Python Program Execution
- Program 7: Find Difference of Two Numbers - 1000+ Python Programs
- Program 12: Calculate Area and Circumference of Circle - 1000+ Python Programs
- Program 9: Divide Two Numbers - 1000+ Python Programs
- Program 2: Print your name using print() function - 1000+ Python Programs
- 25: How to rename a file using Python Program
- 17: Find Factorial of a Number - 1000+ Python Programs
- Program 6: Find Sum of Two Floating Numbers - 1000+ Python Programs
- 23: Python Programs to concatenate two Lists
- 36: Python Program Convert Hex String to Integer
- 20 - Python - Print Colors for Text in Terminal - 1000+ Python Programs
- Python Program: Use NumPy to generate a random number between 0 and 1
- 32: Python Program to Find Square Root of a Number
- Program 8: Multiply Two Numbers - 1000+ Python Programs
- Program 11: Calculate Percentage - 1000+ Python Programs
- 18: Get Sub List By Slicing a Python List - 1000+ Python Programs
- 28: Program to Lowercase a String in Python
- Program 1: Print Hello World! - 1000+ Python Programs
- 21: Program to Delete File or Folder in Python
- 29: Program to convert Python dict to dataframe
- 33: Python Program to find the current time in India (IST)
More Posts:
- Get HTTP Request Response Headers Safari Browser - MacOS
- 🎃 Trending, Popular Halloween hashtags for year 2020 🎃 [Facebook, Twitter, Instagram, Snapchat] - Hashtags
- Write to File in Java using BufferedWriter - Java
- Windows 365: Restore Deleted Distribution Group using Admin Center (Active Directory) - Windows
- Online XML Code Formatter (Prettify) Tool - Tools
- PowerShell: Grep Command Alternative - Select-String - Powershell
- Java: max() and min() methods java.lang.Math - Java
- How to Convert LocalDate to Date in Java 8 and Above - Java