Question 8)
Write a program in Python to divide two numbers.
Write a program in Python to divide numbers 10 by 2.
Write a program to divide two numbers by taking user input using Python Programming.
Program to find the divide two integer values in Python.
Program to find the divide two float values in Python.
Code Example 1: Divide two Integers
# Program 9: Example 1
# Divide two hardcoded int values 2 and 5
# 1000+ Python Programs by Code2care.org
int_no_1 = 10
int_no_2 = 2
divide_value = int_no_1 / int_no_2
print(f"Division of {int_no_1} and {int_no_2} is {divide_value}")
Output:
Division of 10 and 2 is 5
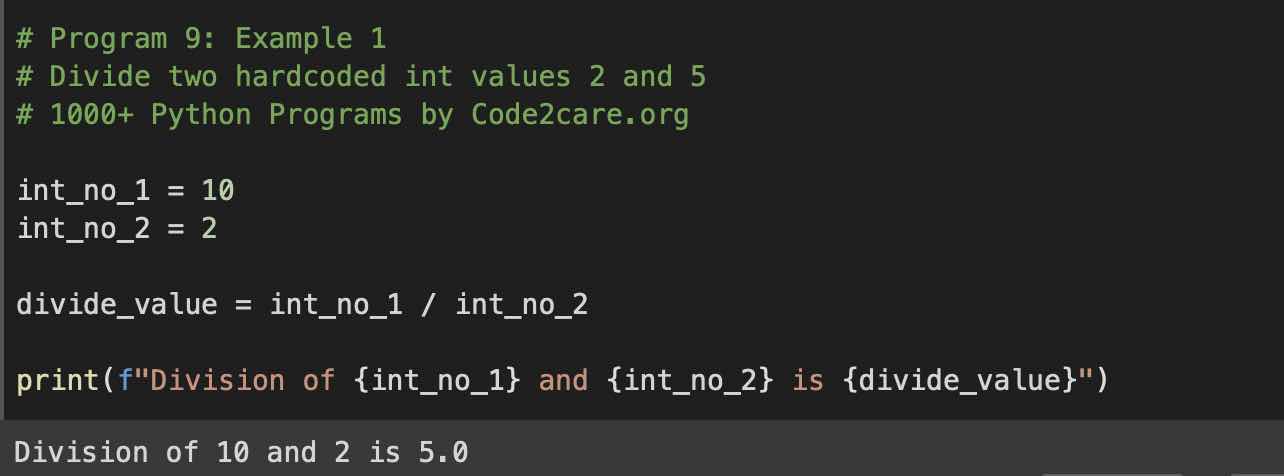
Code Example 2: Divide two integer numbers from user input
# Program 9: Example 2
# Divide two ints from the user input
# 1000+ Python Programs by Code2care.org
int_no_1 = int(input("Enter int 1: "))
int_no_2 = int(input("Enter int 2: "))
divide_value = int_no_1 / int_no_2
print(f"Division of {int_no_1} by {int_no_2} is {divide_value}")
Output:
Enter int 1: 20
Enter int 2: 2
Division of 20 by 2 is 10
Code Example 3: Divide two floats numbers from user input
# floats 8: Example 3
# Divide two ints from the user input
# 1000+ Python Programs by Code2care.org
float_no_1 = float(input("Enter int 1: "))
float_no_2 = float(input("Enter int 2: "))
divide_value = float_no_1 / float_no_2
print(f"Division of {int_no_1} by {int_no_2} is {divide_value}")
Output:
Enter int 1: 10.4
Enter int 2: 2.4
Division of 10.4 and 2.4 is 24.96
Explaination:
List of functions used,
- print(): Built-in function to print a string on the console with f-strings formatting.
- float(): Built-in function to convert String argument to float value.
- input(): Built-in function to accept user input
Steps:
- Create a variable to hold the first integer/float
- Create a variable to hold a second integer/float
- Create a third variable to hold the division of two variables using the / (slash) division operator.
- Print the result using print() function.
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Program 5: Find Sum of Two Integer Numbers - 1000+ Python Programs
- 34: Traverse a List in Reverse Order - 1000+ Python Programming
- 22: Send Yahoo! Email using smtplib - SMTP protocol client using Python Program
- 35: Python Program to find the System Hostname
- 27: Measure Elapsed Time for a Python Program Execution
- Program 7: Find Difference of Two Numbers - 1000+ Python Programs
- Program 12: Calculate Area and Circumference of Circle - 1000+ Python Programs
- Program 9: Divide Two Numbers - 1000+ Python Programs
- Program 2: Print your name using print() function - 1000+ Python Programs
- 25: How to rename a file using Python Program
- 17: Find Factorial of a Number - 1000+ Python Programs
- Program 6: Find Sum of Two Floating Numbers - 1000+ Python Programs
- 23: Python Programs to concatenate two Lists
- 36: Python Program Convert Hex String to Integer
- 20 - Python - Print Colors for Text in Terminal - 1000+ Python Programs
- Python Program: Use NumPy to generate a random number between 0 and 1
- 32: Python Program to Find Square Root of a Number
- Program 8: Multiply Two Numbers - 1000+ Python Programs
- Program 11: Calculate Percentage - 1000+ Python Programs
- 18: Get Sub List By Slicing a Python List - 1000+ Python Programs
- 28: Program to Lowercase a String in Python
- Program 1: Print Hello World! - 1000+ Python Programs
- 21: Program to Delete File or Folder in Python
- 29: Program to convert Python dict to dataframe
- 33: Python Program to find the current time in India (IST)
- SharePoint installation error - Setup is unable to proceed due to the following error This product requires Microsoft .Net Framework 4.5 - SharePoint
- [Fix] reCAPTCHA not working in Web Browser - Google
- Convert Java Object to JSON using Jackson Library - Java
- Fix Microsoft Teams Error code - 107 - Teams
- [Fix] Microsoft Teams No Network Connection Please check your network settings and try again. [2603] - Teams
- How to revert a single file from Git Repo - Git
- How to stop MongoDB Server running on Ubuntu - Ubuntu
- Can we move apps like WhatsApp, Facebook to external MicroSD card - WhatsApp