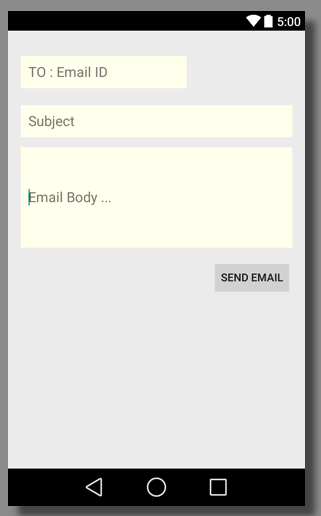
Android Send Email from Activity
Intents are messenger objects that are used to call Activities from a particular activity class. There are two types of Intents that you must know Explicit and Implicit Intents.
Implicit intents are used to call Activities that are defined within your project folder. To call other App Activity we need to use Explicit intents.
The code to send an email through your Android application from a Activity class is pretty simple. When this block of code is executed, a Dialog box is displayed with all the Email clients that are available on the device (e.g. Gmail, Mail, Outlook, Hotmail, Yahoomail etc), If no email client is found that you may get a pop-up saying "No Application can perform this action".
Steps:
- Create an Intent object emailIntent with Intent.ACTION_SEND
- Set type as message/rfc822 i.e. for MIME type
- Use Intent.EXTRA_EMAIL as a key to putExtra and where you need to add the Email-ID of the recipient.
- Use Intent.EXTRA_SUBJECT key and pass the value as the Subject of Email.
- Similarly Intent.EXTRA_TEXT value is the Body of the Email.
- In a try catch block call startActivity()
- In the Catch block if ActivityNotFoundException has occurred, i.e No Email client is found, we display a Toast Message.
Intent emailIntent = new Intent(Intent.ACTION_SEND);
emailIntent.setType("message/rfc822");
emailIntent.putExtra(Intent.EXTRA_EMAIL , ""); // email id can be hardcoded too
emailIntent.putExtra(Intent.EXTRA_SUBJECT, "Hello");
emailIntent.putExtra(Intent.EXTRA_TEXT , "This is the Body!");
try {
startActivity(Intent.createChooser(emailIntent, "Done!"));
} catch (android.content.ActivityNotFoundException ex) {
Toast.makeText(MainActivity.this, "No Email client found!!",
Toast.LENGTH_SHORT).show();
}
package com.code2care.example.emailexample;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends ActionBarActivity {
private EditText emailID,emailSubject,emailBody;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
emailID = (EditText) findViewById(R.id.emailID);
emailSubject = (EditText) findViewById(R.id.emailSubject);
emailBody = (EditText) findViewById(R.id.emailBody);
}
public void sendEmail(View view) {
Intent emailIntent = new Intent(Intent.ACTION_SEND);
emailIntent.setType("message/rfc822");
emailIntent.putExtra(Intent.EXTRA_EMAIL , emailID.getText().toString());
emailIntent.putExtra(Intent.EXTRA_SUBJECT, emailSubject.getText().toString());
emailIntent.putExtra(Intent.EXTRA_TEXT , emailBody.getText().toString());
try {
startActivity(Intent.createChooser(emailIntent, "Select a Email Client"));
} catch (android.content.ActivityNotFoundException ex) {
Toast.makeText(MainActivity.this, "No Email client found!!",
Toast.LENGTH_SHORT).show();
}
}
}
Layout.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.code2care.example.toastasservice.ToastasService" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello_world" />
</RelativeLayout>
More Posts related to Android,
- Increase Android Emulator Timeout time
- Android : Remove ListView Separator/divider programmatically or using xml property
- Error : Invalid key hash.The key hash does not match any stored key hashes
- How to Change Android Toast Position?
- Android Alert Dialog with Checkboxes example
- Android : No Launcher activity found! Error
- Android Development: Spinners with Example
- Failed to sync Gradle project Error:failed to find target android-23
- INSTALL_FAILED_INSUFFICIENT_STORAGE Android Error
- Disable Fading Edges Scroll Effect Android Views
- How to create Toast messages in Android?
- Channel 50 SMSes received every few minutes Android Phones
- Android xml error Attribute is missing the Android namespace prefix [Solution]
- Create Custom Android AlertDialog
- How To Disable Landscape Mode in Android Application
- Android Development - How to switch between two Activities
- incorrect line ending: found carriage return (\r) without corresponding newline (\n)
- Generate Facebook Android SDK keyhash using java code
- Android Error Generating Final Archive - Debug Certificate Expired
- 21 Useful Android Emulator Short-cut Keyboard Keys
- Android RatingBar Example
- 11 Weeks of Android Online Sessions-15-Jun-to-28-Aug-2020
- Download interrupted: Unknown Host dl-ssl.google.com Error Android SDK Manager
- fill_parent vs match_parent vs wrap_content
- Android : Connection with adb was interrupted 0 attempts have been made to reconnect
More Posts:
- Fix: java: void cannot be dereferenced - Java
- Get Current Local Date and Time using Java 8 DateTime API - Java
- Bootstrap Nav Menu Dropdown on hover - Bootstrap
- Obsolete marquee element alternatives html5 - Html
- Docker Commit Command with Examples - Docker
- How to Check AWS SNS Permissions using CLI - AWS
- Android Studio : Change FAB icon color : FloatingActionButton - Android-Studio
- Capitalize the first letter of each word using Notepad++ - NotepadPlusPlus