The preferred way to initialize a HashMap in Java is by using the default constructor and instantiating it as a Map interface.
Map<String, String> map = new HashMap<>();
As Map is an interface in Java, map variable here can store an instance of any class that implements the Map interface, such as HashMap. This allows for flexibility and code maintainability, as you can switch to a different implementation of the Map interface without changing the rest of your code.
Initialize HashMap as an Immutable Map
Map<String, String> map = Collections.unmodifiableMap(map);
Using Java 8 Streams
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class HashMapUsingStreams {
public static void main(String[] args) {
Map<String, String> countryCapitalMap = Stream.of(new String[][] {
{ "USA", "Washington, D.C." },
{ "Canada", "Ottawa" },
{ "France", "Paris" },
{ "Germany", "Berlin" },
{ "Japan", "Tokyo" }
}).collect(Collectors.toMap(data -> data[0], data -> data[1]));
System.out.println(countryCapitalMap);
}
}
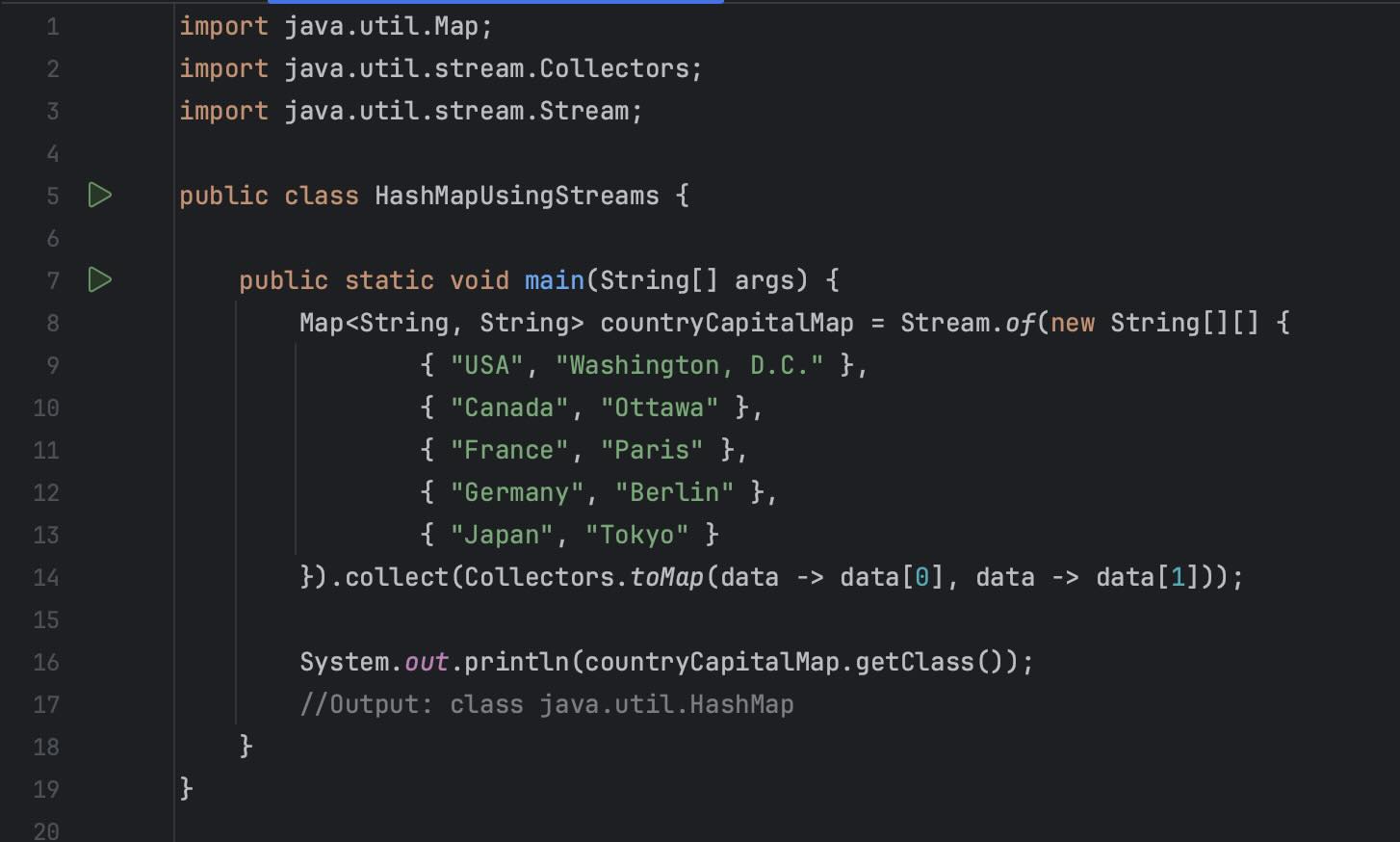
Java 9 or later: Using Initialization with Values
HashMap<KeyType, ValueType> hashMap = new HashMap<>(Map.of(
key1, value1,
key2, value2,
key3, value3
));
another way!
HashMap<KeyType, ValueType> hashMap = new HashMap<>(Map.ofEntries(
entry(key1, value1),
entry(key2, value2),
entry(key3, value3)
));
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Read a file and Split using StringTokenizer in Java - Java
- Run DynamoDB Local on Docker Container - Docker
- Python: Fix command not found pip or pip3 on zsh shell - Python
- Fix 0x80070194 Error When Opening Microsoft OneDrive File - Microsoft
- Android ListView turns Black or Flickers while Scrolling - Android
- Enable Dark Mode in Google Search - Google
- How to run Gradle build in offline mode - Gradle
- Python: Access Environment Variables - Python