At times you may not want to run certain test cases in Junit, in such cases make use of the annotation @Ignore on such test methods.
Greeting.javapackage org.code2care;
public class Greeting {
public String wishMorning(String name) {
return "Hello " + name + ", Good Morning!";
}
public String wishAfternoon(String name) {
return "Hello " + name + ", Good Afternoon!";
}
public String wishEvening(String name) {
return "Hello " + name + ", Good Evening!";
}
}
GreetingTest.java
package myprog;
import static org.junit.Assert.assertEquals;
import org.code2care.Greeting;
import org.junit.Before;
import org.junit.Ignore;
import org.junit.Test;
public class GreetingTest {
Greeting greeting;
@Before
public void init() {
greeting = new Greeting();
}
@Test
@Ignore
public void wishMorningTest() {
assertEquals("Hello Neo, Good Morning!", greeting.wishMorning("Neo"));
}
@Test
public void wishAfternoonTest() {
assertEquals("Hello Neo, Good Afternoon!", greeting.wishAfternoon("Neo"));
}
@Test
public void wishEveningTest() {
assertEquals("Hello Neo, Good Evening!", greeting.wishEvening("Neo"));
}
}
Test Run Results:
-------------------------------------------------------
T E S T S
-------------------------------------------------------
Running myprog.GreetingTest
Tests run: 3, Failures: 0, Errors: 0, Skipped: 1, Time elapsed: 0.012 sec
Results :
Tests run: 3, Failures: 0, Errors: 0, Skipped: 1
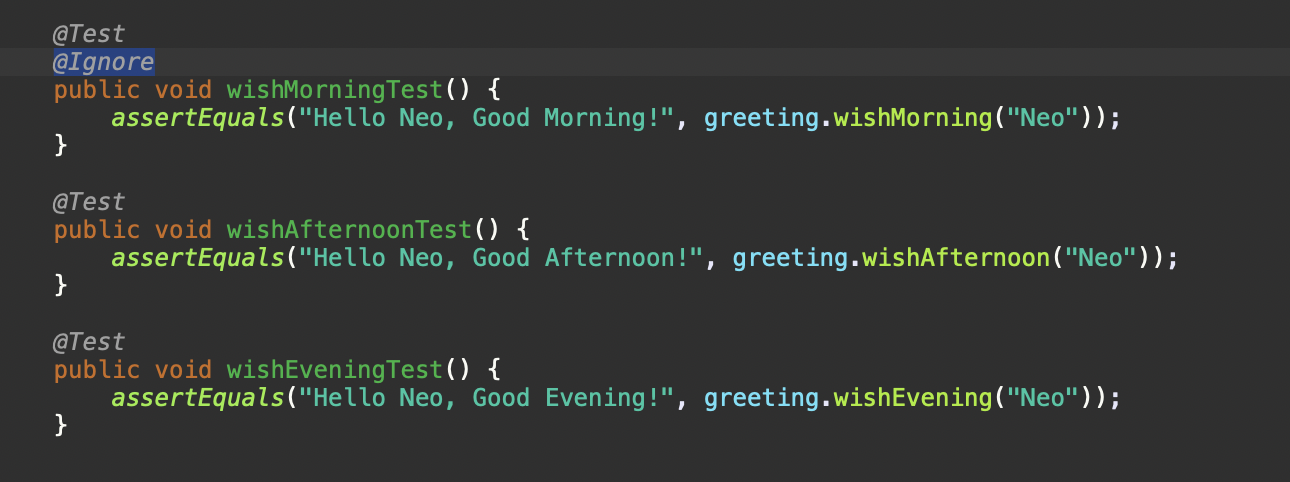
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Failed to find provider info for com.facebook.katana.provider.PlatformProvider - Android
- Install OpenSSL on Linux/Ubuntu - Linux
- How to Check AWS SNS Permissions using CLI - AWS
- Android : IOException: Unable to open sync connection! - Android
- Find your macOS version - MacOS
- Error : Facebook SDK AndroidRuntime?FATAL EXCEPTION: main - Android
- How to switch from bash to zsh shell in macOS Terminal - MacOS
- How to URL Decode a Query String in Python - Python