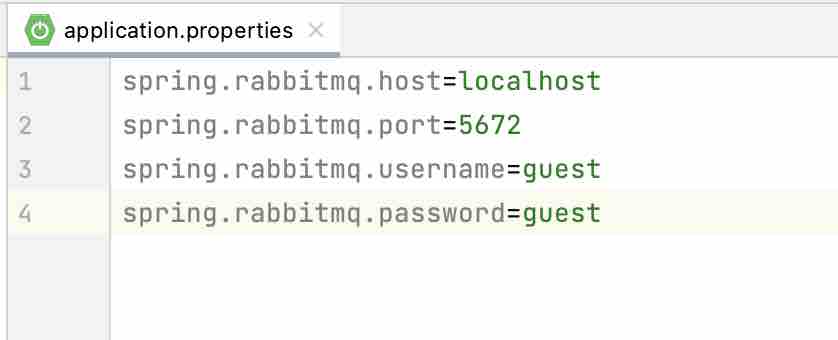
Spring Boot makes it very easy to work on a project by reducing alot of boilerplate code and making the most of the application.properties key/values pairs.
Let us take a look at some of the most important lists of such properties when working with Spring Boot and RabbitMQ.
- RabbitMQ Connection Properties
Below are the four main properties that you will use to make a connect to the RabbitMQ server.
spring.rabbitmq.host=localhost # RabbitMQ Server Host IP/Domain spring.rabbitmq.port=5672 # RabbitMQ Server Port (default port 5672) spring.rabbitmq.username=guest # RabbitMQ username (default guest) spring.rabbitmq.password=guest # RabbitMQ password (default guest)
-
This property allows you to control if the queues, exchanges, or bindings should be declared automatically or not.
spring.rabbitmq.dynamic=true/false
-
Propery to set connection timeout.
spring.rabbitmq.connection-timeout=30000 #Connection timeout in milli-seconds
Using this property you can set the maximum number of allowed channels.
spring.rabbitmq.cache.channel.size=20 #max number fo channels allowed
Use this property to set the maximum number of connections in a cache.
spring.rabbitmq.cache.connection.size=5
Use the below two properties for publisher confirmation and returns.
spring.rabbitmq.publisher-confirms=true/false spring.rabbitmq.publisher-returns=true/false
Complete List:
spring.rabbitmq.address-shuffle-mode
spring.rabbitmq.addresses
spring.rabbitmq.cache.channel.checkout-timeout
spring.rabbitmq.cache.channel.size
spring.rabbitmq.cache.connection.mode
spring.rabbitmq.cache.connection.size
spring.rabbitmq.connection-timeout
spring.rabbitmq.dynamic
spring.rabbitmq.host
spring.rabbitmq.listener.direct.acknowledge-mode
spring.rabbitmq.listener.direct.auto-startup
spring.rabbitmq.listener.direct.consumers-per-queue
spring.rabbitmq.listener.direct.de-batching-enabled
spring.rabbitmq.listener.direct.default-requeue-rejected
spring.rabbitmq.listener.direct.idle-event-interval=
spring.rabbitmq.listener.direct.missing-queues-fatal=false
spring.rabbitmq.listener.direct.prefetch=
spring.rabbitmq.listener.direct.retry.enabled=false
spring.rabbitmq.listener.direct.retry.initial-interval=1000ms
spring.rabbitmq.listener.direct.retry.max-attempts=3
spring.rabbitmq.listener.direct.retry.max-interval=10000ms
spring.rabbitmq.listener.direct.retry.multiplier=1
spring.rabbitmq.listener.direct.retry.stateless=true
spring.rabbitmq.listener.simple.acknowledge-mode=
spring.rabbitmq.listener.simple.auto-startup=true
spring.rabbitmq.listener.simple.batch-size=
spring.rabbitmq.listener.simple.concurrency=
spring.rabbitmq.listener.simple.consumer-batch-enabled=false
spring.rabbitmq.listener.simple.de-batching-enabled=true
spring.rabbitmq.listener.simple.default-requeue-rejected=
spring.rabbitmq.listener.simple.idle-event-interval=
spring.rabbitmq.listener.simple.max-concurrency=
spring.rabbitmq.listener.simple.missing-queues-fatal=true
spring.rabbitmq.listener.simple.prefetch
spring.rabbitmq.listener.simple.retry.enabled=false
spring.rabbitmq.listener.simple.retry.initial-interval=1000ms
spring.rabbitmq.listener.simple.retry.max-attempts=3
spring.rabbitmq.listener.simple.retry.max-interval=10000ms
spring.rabbitmq.listener.simple.retry.multiplier=1
spring.rabbitmq.listener.simple.retry.stateless=true
spring.rabbitmq.listener.stream.auto-startup=true
spring.rabbitmq.listener.stream.native-listener=false
spring.rabbitmq.listener.type
spring.rabbitmq.password
spring.rabbitmq.port
spring.rabbitmq.publisher-confirm-type
spring.rabbitmq.publisher-returns=false
spring.rabbitmq.requested-channel-max
spring.rabbitmq.requested-heartbeat
spring.rabbitmq.ssl.algorithm
spring.rabbitmq.ssl.enabled
spring.rabbitmq.ssl.key-store
spring.rabbitmq.ssl.key-store-algorithm=SunX509
spring.rabbitmq.ssl.key-store-password=
spring.rabbitmq.ssl.key-store-type=PKCS12
spring.rabbitmq.ssl.trust-store-algorithm=SunX509
spring.rabbitmq.ssl.trust-store-password=
spring.rabbitmq.ssl.trust-store-type=JKS
spring.rabbitmq.ssl.trust-store=
spring.rabbitmq.ssl.validate-server-certificate
spring.rabbitmq.ssl.verify-hostname
spring.rabbitmq.stream.host
spring.rabbitmq.stream.name
spring.rabbitmq.stream.password
spring.rabbitmq.stream.port
spring.rabbitmq.stream.username
spring.rabbitmq.template.default-receive-queue
spring.rabbitmq.template.exchange
spring.rabbitmq.template.mandatory
spring.rabbitmq.template.receive-timeout
spring.rabbitmq.template.reply-timeout
spring.rabbitmq.template.retry.enabled
spring.rabbitmq.template.retry.initial-interval
spring.rabbitmq.template.retry.max-attempts
spring.rabbitmq.template.retry.max-interval
spring.rabbitmq.template.retry.multiplier=
spring.rabbitmq.template.routing-key
spring.rabbitmq.username=guest
spring.rabbitmq.virtual-host
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Where is Cron Crontab jobs log file Located in Ubuntu Linux - Ubuntu
- [fix] Java NullPointerException ComparableTimSort countRunAndMakeAscending when sorting a List - Java
- How to Send Email using Java - Java
- How to remove quotes from a String in Python - Python
- How to know Roblox Version Details on Mac - MacOS
- How to change Chrome Spell Check from UK English to US English - Chrome
- How to Toggle Light/Dark Mode in Google Colab - Google
- Get HTML table td, tr or th inner content value with id or name attribute - Html