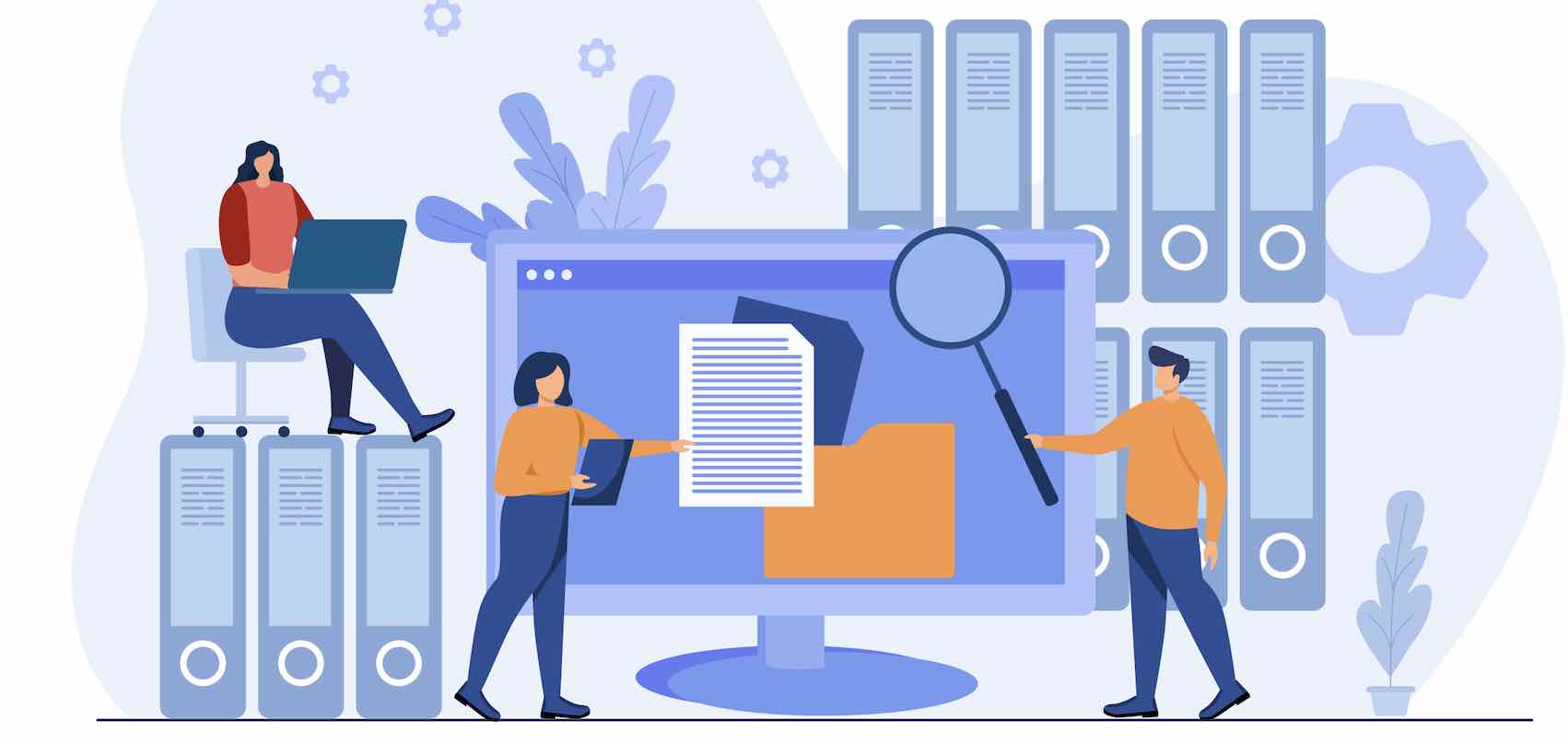
In order to pretty-print a JSON String in Java console output, we can make use of the Jackson Library, let's see an example,
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.IOException;
public class PrettyPrintJson {
public static void main(String[] args) {
try {
String jsonString = "{\n" +
" \"artistName\":\"Michael Jackson\",\n" +
" \"albumName\":\"Thriller\",\n" +
" \"releaseYear\":1982,\n" +
" \"songsList\":[\n" +
" \"Wanna Be Startin Somethin\",\n" +
" \"Baby Be Mine\",\n" +
" \"The Girl Is Mine\",\n" +
" \"Thriller\",\n" +
" \"Beat it\",\n" +
" \"Billie Jean\",\n" +
" \"Human Nature\",\n" +
" \"P.Y.T. (Pretty Young Thing)\",\n" +
" \"The Lady in My Life\"\n" +
" ]\n" +
"}";
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
String prettyPrintedJson = objectMapper.writerWithDefaultPrettyPrinter().writeValueAsString(jsonNode);
System.out.println(prettyPrintedJson);
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
{
"artistName" : "Michael Jackson",
"albumName" : "Thriller",
"releaseYear" : 1982,
"songsList" : [ "Wanna Be Startin Somethin", "Baby Be Mine", "The Girl Is Mine", "Thriller", "Beat it", "Billie Jean", "Human Nature", "P.Y.T. (Pretty Young Thing)", "The Lady in My Life" ]
}
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Convert Java Map Collection Object to JSON String using Jackson - Java
- How to Update Google Chrome Browser on Mac? - Chrome
- INSTALL_FAILED_INSUFFICIENT_STORAGE Error Android Emulator - Android
- How to Show Battery percentage on Big Sur Menu Bar - MacOS
- How to refresh Safari on Mac (macOS) using keyboard shortcut - MacOS
- 46: Take a list of numbers and print each element. [1000+ Python Programs] - Python-Programs
- How to Fix Spelling Errors on Microsoft Word for Mac - HowTos
- IntelliJ Keyboard Shortcut to remove unused imports [Java] - Java