A String is said to be alphanumeric if,
- It contains lowercase alphabets from 'a' to 'z'.
- It contains uppercase alphabets from 'A' to 'Z'.
- It contains digits from '0' to '9'.
- It does not contain any special characters, punctuation marks, or spaces.
- The string may contain a combination of lowercase letters, uppercase letters, and digits in any order.
- The length of the string can be any positive integer, including zero (empty string).
Examples:
String | Validity |
---|---|
"abc123" | Valid |
"HelloWorld" | Valid |
"123456" | Valid |
"AbCdEfG" | Valid |
"aBc123" | Valid |
"a1b2c3" | Valid |
"Alphanumeric123" | Valid |
"Invalid String!" | Invalid |
"Spaces Are NotAllowed" | Invalid |
"Special@Characters" | Invalid |
"123_456" | Invalid |
"" | Valid |
Now that we know what Alphanumerics are, let's take an example using Java RegEx.
package org.code2care.examples;
import java.util.Arrays;
import java.util.List;
import java.util.regex.Pattern;
public class AlphanumericRegExValidation {
public static void main(String[] args) {
List<String> strings = Arrays.asList(
"abc123",
"HelloWorld",
"123456",
"AbCdEfG",
"aBc123",
"a1b2c3",
"Alphanumeric123",
"Invalid String!",
"Spaces Are NotAllowed",
"Special@Characters",
"123_456",
""
);
Pattern pattern = Pattern.compile("^[a-zA-Z0-9]+$");
for (String str : strings) {
boolean isValid = pattern.matcher(str).matches();
System.out.println("String: " + str + " is Alphanumeric: " + isValid);
}
}
}
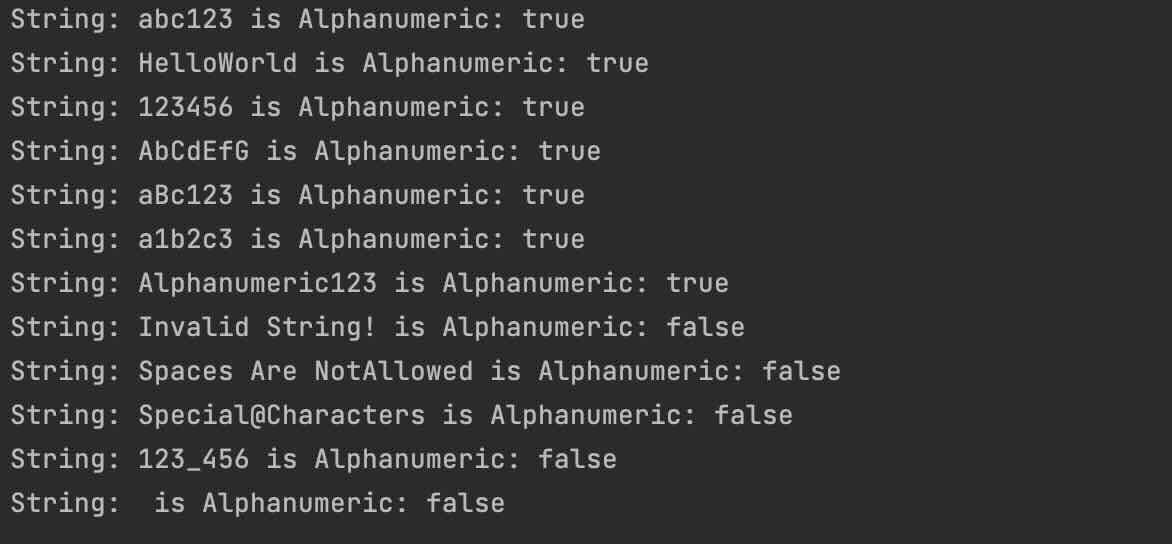
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to create SharePoint Online List Item using REST API - SharePoint
- Mac: How to Open Android Studio from Terminal - MacOS
- How to See Hidden Folders and Files on macOS - Mac-OS-X
- What is $$ in Bash Shell Script- Special Variable - Bash
- How to Create Absolute References in Microsoft Excel for Mac - Microsoft
- How to Select All Text in Vim/Vi editor - vi
- How to install Python 2.7.x version on macOS Ventura/Sonoma - Python
- How to Clear Cache for a website (URL) in Safari for Mac - MacOS