Predicate is a boolean-valued functional interface with test() method that takes one argument and returns either true or false.
Syntax:
@FunctionalInterface
public interface Predicate<T> {
boolean test(T t);
}
Some Examples of Usage of Predicate with Lambadas
Example 1: To know if a number is even or odd:
public static void main(String[] args) {
Predicate<Integer> evenOrOdd = num -> num % 2 == 0;
int testNumberForEvenOdd = 5;
boolean isEven = evenOrOdd.test(testNumberForEvenOdd);
System.out.println(testNumberForEvenOdd + " is Even: " + isEven);
}
Output:
Example 2: To check if today is Weekday or Weekend:
package org.code2care.examples;
import java.time.DayOfWeek;
import java.time.LocalDate;
import java.util.function.Predicate;
public class WeekendChecker {
public static void main(String... args) {
Predicate<LocalDate> isWeekend = date -> {
DayOfWeek dayOfWeek = date.getDayOfWeek();
return dayOfWeek == DayOfWeek.SATURDAY || dayOfWeek == DayOfWeek.SUNDAY;
};
LocalDate currentDate = LocalDate.now();
if (isWeekend.test(currentDate)) {
System.out.println("Today is a weekend day.");
} else {
System.out.println("Today is not a weekend day.");
}
}
}
Output:
Example 3: Check if the provided number is greater than 100
public static void main(String[] args) {
Predicate<Integer> isGreaterThan100 = number -> number > 100;
int numberToCheck = 80;
if (isGreaterThan100.test(numberToCheck)) {
System.out.println(numberToCheck + " is greater than 100.");
} else {
System.out.println(numberToCheck + " is not greater than 100.");
}
}
Output:
Example 4: Check if the object is nul
package org.code2care.examples;
import java.util.function.Predicate;
public class NullChecker {
public static void main(String[] args) {
Predicate<Object> isNull = obj -> obj == null;
Object objectToCheck = null;
if (isNull.test(objectToCheck)) {
System.out.println("The object is null.");
} else {
System.out.println("The object is not null.");
}
}
}
Output:
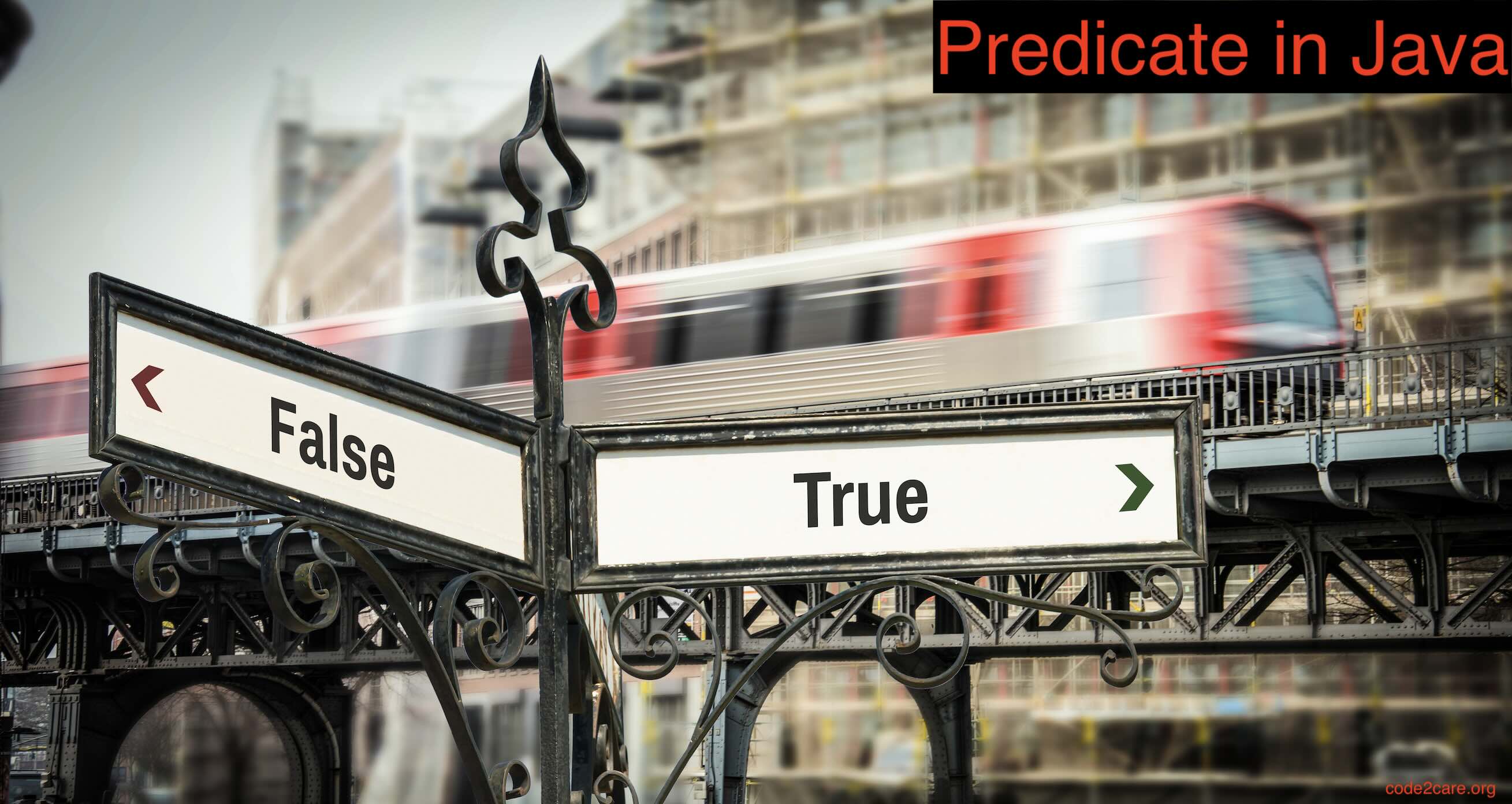
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- [Solution] SFTP Connection closed File 22 not found - FTP
- Make Android Toolbar text center aligned - Android
- RabbitMQ Queue Listener Java Spring Boot Code Example - Java
- Ubuntu Linux: How to Login as root User - Ubuntu
- list of jars required for hibernate 4.x.x - Java
- Health Status Page for OpenAI ChatGPT or GPT 4 - HowTos
- Fix Microsoft Teams Admin Center error - The Security zone setting isnt configured correctly - Teams
- CSS Background Opacity with Examples - CSS