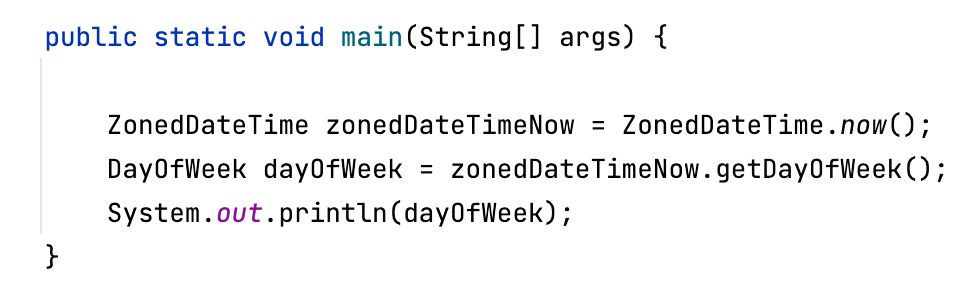
In this article, we will take a look at how to get the day of the week using the Java Date and Time API (Java 8 and above),
1. Get day of the week for LocalDateTime Object
With LocalDateTime you can make use of the getDayOfWeek() method to get the DayOfWeek,
import java.time.DayOfWeek;
import java.time.LocalDateTime;
public class Java8DayOfWeekExample1 {
public static void main(String[] args) {
LocalDateTime currentDateTime = LocalDateTime.now();
DayOfWeek dayOfWeek = currentDateTime.getDayOfWeek();
System.out.println(dayOfWeek);
}
}
SATURDAY
2. Get day of the week for LocalDate Object
import java.time.DayOfWeek;
import java.time.LocalDate;
public class Java8DayOfWeekExample2 {
public static void main(String[] args) {
LocalDate currentDateTime = LocalDate.now();
DayOfWeek dayOfWeek = currentDateTime.getDayOfWeek();
System.out.println(dayOfWeek);
}
}
MONDAY
3. Get day of the week for ZonedDateTime Object
import java.time.DayOfWeek;
import java.time.ZonedDateTime;
public class Java8DayOfWeekExample3 {
public static void main(String[] args) {
ZonedDateTime zonedDateTimeNow = ZonedDateTime.now();
DayOfWeek dayOfWeek = zonedDateTimeNow.getDayOfWeek();
System.out.println(dayOfWeek);
}
}
TUESDAY
4. Get day of the week for Instant Object
As Instant is associated with seconds or mill seconds, we need to convert it to a LocalDate/LocalDateTime/ZonalDateTime in order to get the week of the day!
import java.time.DayOfWeek;
import java.time.Instant;
import java.time.LocalDateTime;
import java.time.ZoneId;
public class Java8DayOfWeekExample4 {
public static void main(String[] args) {
Instant instantNow = Instant.now();
DayOfWeek dayOfWeek = LocalDateTime.ofInstant(instantNow, ZoneId.of("UTC")).getDayOfWeek();
System.out.println(dayOfWeek);
}
SUNDAY
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to check if variable is a number in JavaScript (NaN, typeof, regex) - JavaScript
- PowerShell: How to Get Folder Size - Powershell
- How to install Terraform on M1/M2 Mac - MacOS
- Best way to calculate elapsed time in Java using Java 8 Duration & Instant Class with Nanoseconds precision - Java
- SharePoint Server 2016 setup error - A system restart from a previous installation or update is pending. Restart your computer and run setup to continue. - SharePoint
- How to customize SharePoint Modern list form using JSON formatting - SharePoint
- JavaScript: Convert an Image into Base64 String - JavaScript
- How to find the Length of ArrayList in Java - Java