If you want to hardcode the value for a date in Java then you can make use of the java.time.LocalDate (Java 8 and above) or java.util.Date (pre-Java 8)
Let's take a look at some examples.
Example 1: Using Java 8 or above java.time.LocalDate
LocalDate date = LocalDate.of(2023, 12, 25);
Example 2: Using Java 8 or above Instant
Instant instant = Instant.parse("2023-12-25T00:00:00.00Z");
Example 3: Using Java 6 or above Calendar
Calendar calendar = Calendar.getInstance();
calendar.set(2023, 11, 25);
Example 4: Using Java 7 or before SimpleDateFormat
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
Date date = dateFormat.parse("2023-12-25");
Example 5: Using Java 7 or before java.util.Date
Date date = new Date(123, 11, 25);
Preferred way!
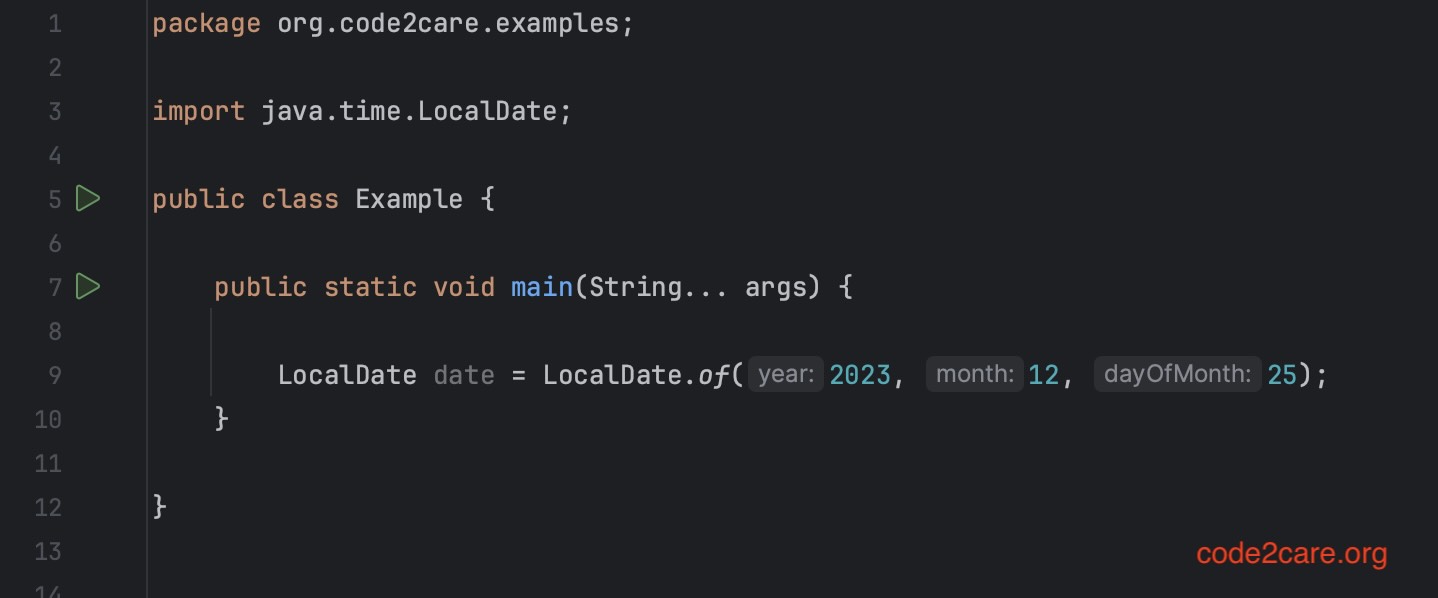
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- BSNL Broadband upgrades speed to minimum 2MBps for all users 512Kbps 1Mbps - HowTos
- How to stop or quit cat command? - HowTos
- How to Find the Location of Notepad on Windows 11 - Windows
- Safari Disable Private Browsing Is Locked macOS Sonoma 14 - MacOS
- Java + Spring JDBC Template + Gradle Example - Java
- Comments in Python Programming - Python
- Round Number up to 2 decimal places in Python - Python
- How to remove JetBrains Toolbox from Mac Startup - HowTos