If you want to get the desc (description) of a table using Java JDBC, you can do that by running a Statment query,
Query:desc students;
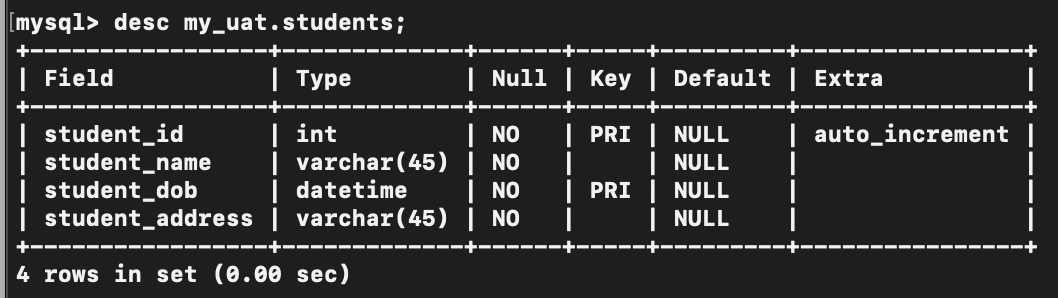
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
public class JDBCTableDescExample {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
String url ="jdbc:mysql://localhost:3306/my_uat";
String userName="root";
String password ="root123";
String insertQuery ="desc students";
Connection connection = DriverManager.getConnection(url,userName,password);
PreparedStatement preparedStatement = connection.prepareStatement(insertQuery);
ResultSet rs = preparedStatement.executeQuery();
ResultSetMetaData resultSetMetaData = rs.getMetaData();
int colCount = resultSetMetaData.getColumnCount();
for(int i=1;i<=colCount;i++) {
System.out.print(resultSetMetaData.getColumnName(i)+"\t |");
}
System.out.println("");
while(rs.next()) {
for(int i=1;i<=colCount;i++) {
System.out.print(rs.getString(i)+"\t |");
}
System.out.println("");
}
}
}
Output:
Field |Type |Null |Key |Default |Extra |
student_id |int |NO |PRI |null |auto_increment |
student_name |varchar(45) |NO | |null | |
student_dob |datetime |NO |PRI |null | |
student_address |varchar(45) |NO | |null | |
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- [Java] Generate Getters and Setters in VS Code - Java
- Unsupported major.minor version 52.0 in java - Java
- Fix: KeyError: exception in Python - Python
- Mac: How to Merge Cells in Excel - MacOS
- Fix: zsh: command not found: aws (Mac/macOS) - AWS
- Terminal display next month Calendar - Linux
- Java Program: Random Number Generator - Java
- How to Check For Updates on Windows 11 (Step-by-Step) - Java