To get the current date and time in Java 8 and above we can make use of LocalDateTime.now().
Example:
package org.code2care.examples;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class Example {
public static void main(String... args) {
LocalDateTime currentDateTime = LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("MM-dd-yyyy HH:mm:ss");
String formattedDateTime = currentDateTime.format(formatter);
System.out.println("Current Date and Time: " + formattedDateTime);
}
}
Output:
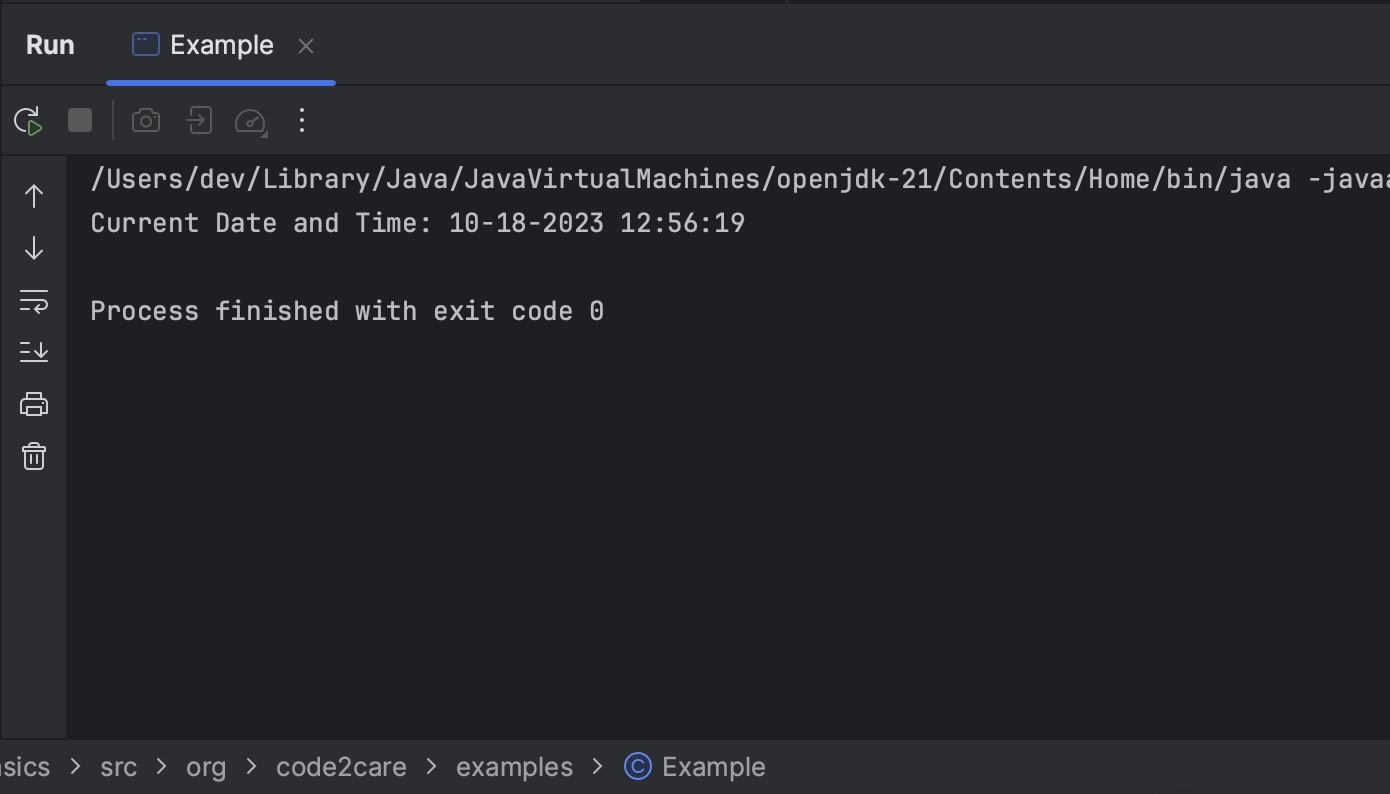
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to Copy Entire Directory to another Directory in Linux - Linux
- FCM Messages Test Notification!!!! - Microsoft Teams, Google Hangouts push alert - News
- Java XML-RPC java.net.BindException: Address already in use - Java
- Power of Print Statements in JavaScript: A Comprehensive Guide - JavaScript
- Fix: Spring Boot + IntelliJ + Gradle : Unsupported class file major version 64 Error - Gradle
- Program 6: Find Sum of Two Floating Numbers - 1000+ Python Programs - Python-Programs
- How to Sign Up for ChatGPT AI Chat Bot with Steps - HowTos
- How to Compare Two SQL Queries in Notepad++ - NotepadPlusPlus