In this example, we take a look at how to generate a SHA-256 hash with a salt for a given input password.
The steps we follow in the code are as follows,
Step 1: We generate a random salt using generateSalt() method. The salt is a random sequence of bytes used to add uniqueness to the hashing process.
Step 2: Next, We combine the user's password with the salt to create a salted password.
Step 3: Finally, we calculate the SHA-256 hash of the salted password using the calculateSHA256() method.
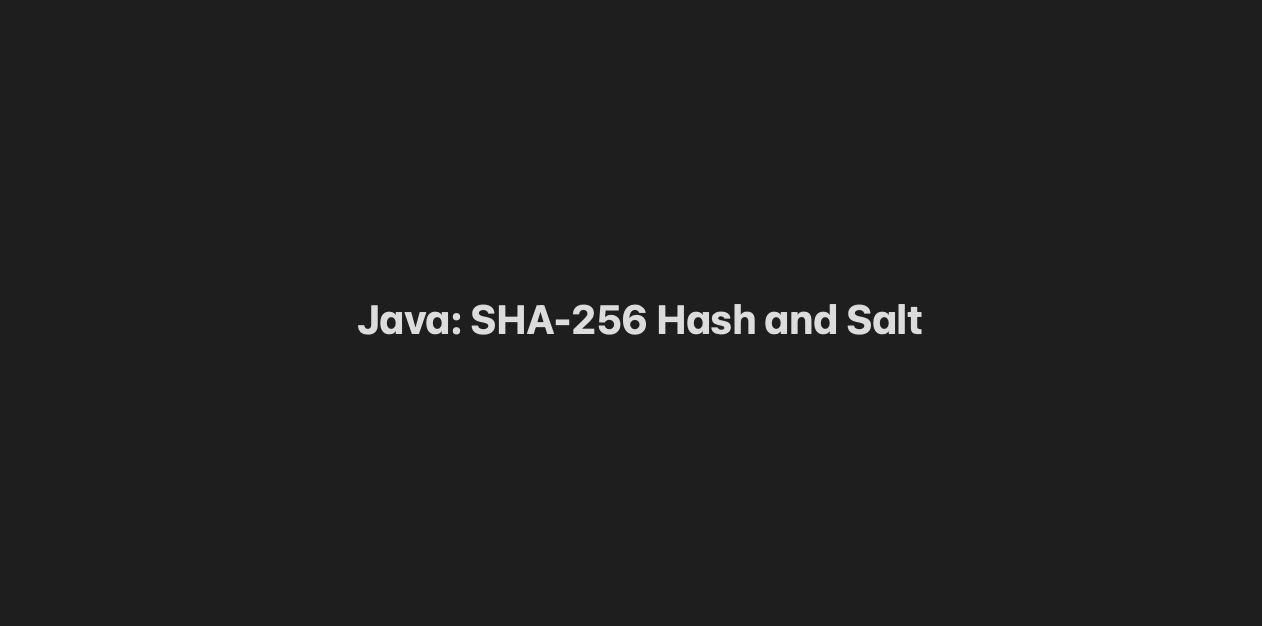
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
public class JavaSHA256HashWithSaltExample {
public static final String SHA256_ALGO = "SHA-256";
public static void main(String[] args) throws NoSuchAlgorithmException {
String password = "Pa$$w*rd@$2_E";
byte[] randomSalt = generateSalt();
String saltedPassword = password + bytesToHex(randomSalt);
String sha256HashedPassword = calculateSHA256(saltedPassword);
System.out.println("Salt: " + bytesToHex(randomSalt));
System.out.println("Hashed Password: " + sha256HashedPassword);
}
public static byte[] generateSalt() {
SecureRandom random = new SecureRandom();
byte[] salt = new byte[64];
random.nextBytes(salt);
return salt;
}
public static String calculateSHA256(String input) throws NoSuchAlgorithmException {
MessageDigest sha256 = MessageDigest.getInstance(SHA256_ALGO);
byte[] hashBytes = sha256.digest(input.getBytes());
return bytesToHex(hashBytes);
}
public static String bytesToHex(byte[] bytes) {
StringBuilder stringBuilder = new StringBuilder();
for (byte b : bytes) {
stringBuilder.append(String.format("%02x", b));
}
return stringBuilder.toString();
}
}
Output:
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode] - Python
- How to know the Version of Notepad App on Windows 11 - Windows-11
- remove div vertical scroll - Html
- [Solution] WslRegisterDistribution failed with error: 0x80370102 VM on Mac - Windows
- How to Pretty Print JSON in PHP - PHP
- Adding Custom ASCII Text Banner in Spring Boot Application - Java
- Python: Convert int to binary String - Python
- SharePoint List redirect user after submitting form NewForm.aspx - SharePoint