In this article, we take a look at how to delete a file using Java with examples.
Exampl 1: Using java.io.File delete() method
package org.code2care.examples;
import java.io.File;
public class DeleteFile {
public static void main(String[] args) {
String fileName = "/Users/c2ctech/Desktop/data.csv";
File dataFile = new File(fileName);
if (dataFile.delete()) {
System.out.println("File " + dataFile.getName() + " deleted successfully!");
} else {
System.out.println("Unable to delete file " + dataFile.getName());
}
}
}
Example 2: Using java.io.File delete()
package org.code2care.examples;
import java.io.File;
public class DeleteFile {
public static void main(String[] args) {
String fileName = "/Users/c2ctech/Desktop/data.csv";
File dataFile = new File(fileName);
if (dataFile.exists()) {
if (dataFile.delete()) {
System.out.println("File " + dataFile.getName() + " deleted successfully!");
} else {
System.out.println("Unable to delete file " + dataFile.getName());
}
} else {
System.out.println("File " + dataFile.getName() + " does not exist.");
}
}
}
Example 3: Using java.nio.file.File
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class DeleteFile {
public static void main(String[] args) {
String fileName = "/Users/c2ctech/Desktop/data.csv";
Path filePath = Paths.get(fileName);
try {
Files.delete(filePath);
System.out.println("File " + fileName + " deleted successfully!");
} catch (Exception e) {
System.out.println("Unable to delete file " + fileName);
e.printStackTrace();
}
}
}
Example 4: Using java.nio.file.Path
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class DeleteFile {
public static void main(String[] args) {
String fileName = "/Users/c2ctech/Desktop/data.csv";
Path filePath = Paths.get(fileName);
try {
Files.deleteIfExists(filePath);
System.out.println("File " + fileName + " deleted successfully!");
} catch (Exception e) {
System.out.println("Unable to delete file " + fileName);
e.printStackTrace();
}
}
}
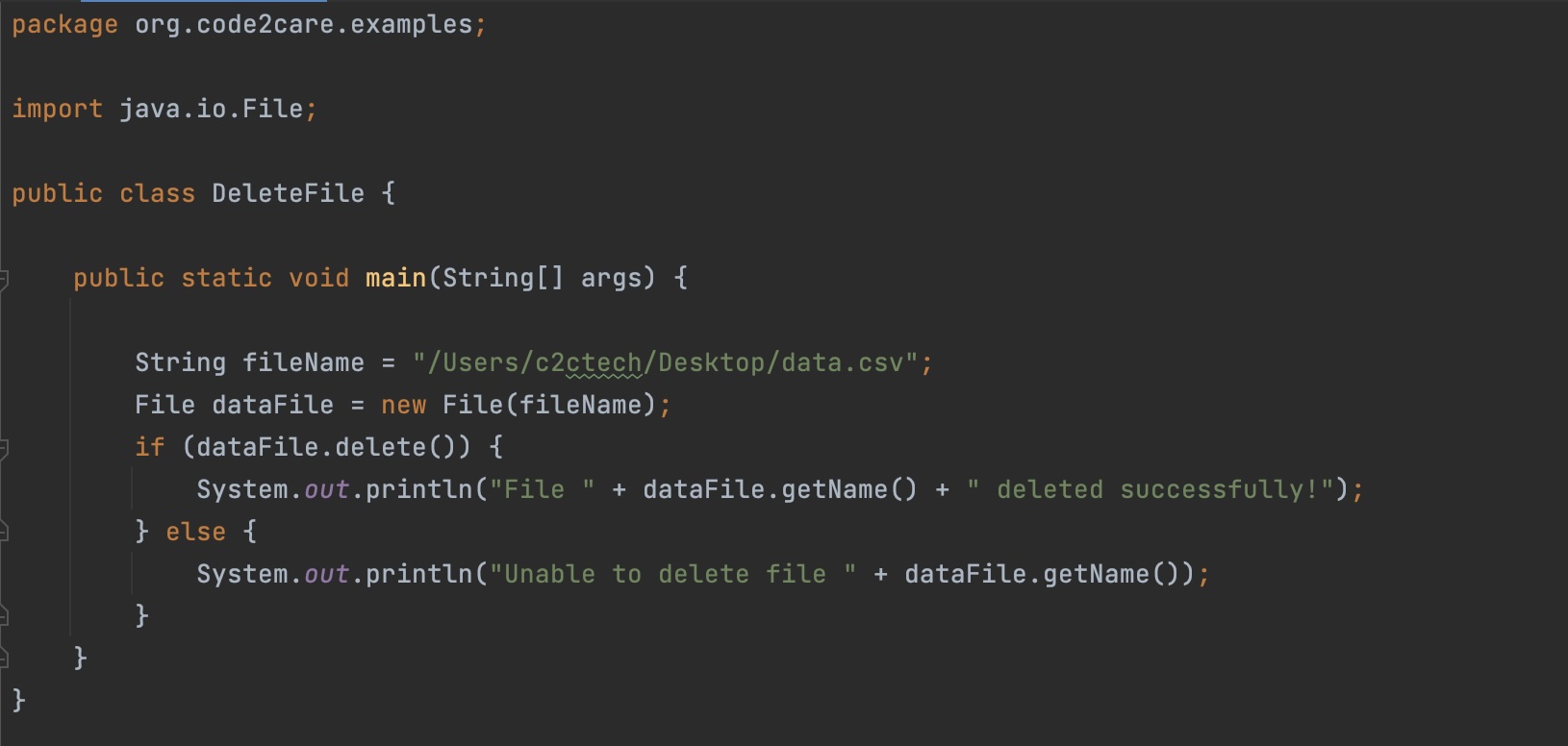
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Cut, Copy and Paste Keyboard Shortcuts on Mac Keyboard - MacOS
- 'pwd' is not recognized as an internal or external command, operable program or batch file. [Windows] - Bash
- How to Turn Dark Mode On in Jupyter Notebook - Python
- How to convert a Excel to PDF file in Mac - MacOS
- ls: .: Operation not permitted - Mac Terminal ZSH Error - MacOS
- Android Toolbar example with appcompat_v7 21 - Android
- How to Create and Run a Command Line Application using Spring Boot - Java
- Android Alert Dialog with Checkboxes example - Android