If you have a requirement to develop a simple application using Spring Boot that runs on the Console (Terminal) then you can follow below two easy steps to get started,
Step 1: Project Dependencies
Go to start.spring.io and do not choose any dependencies and hit Generate.
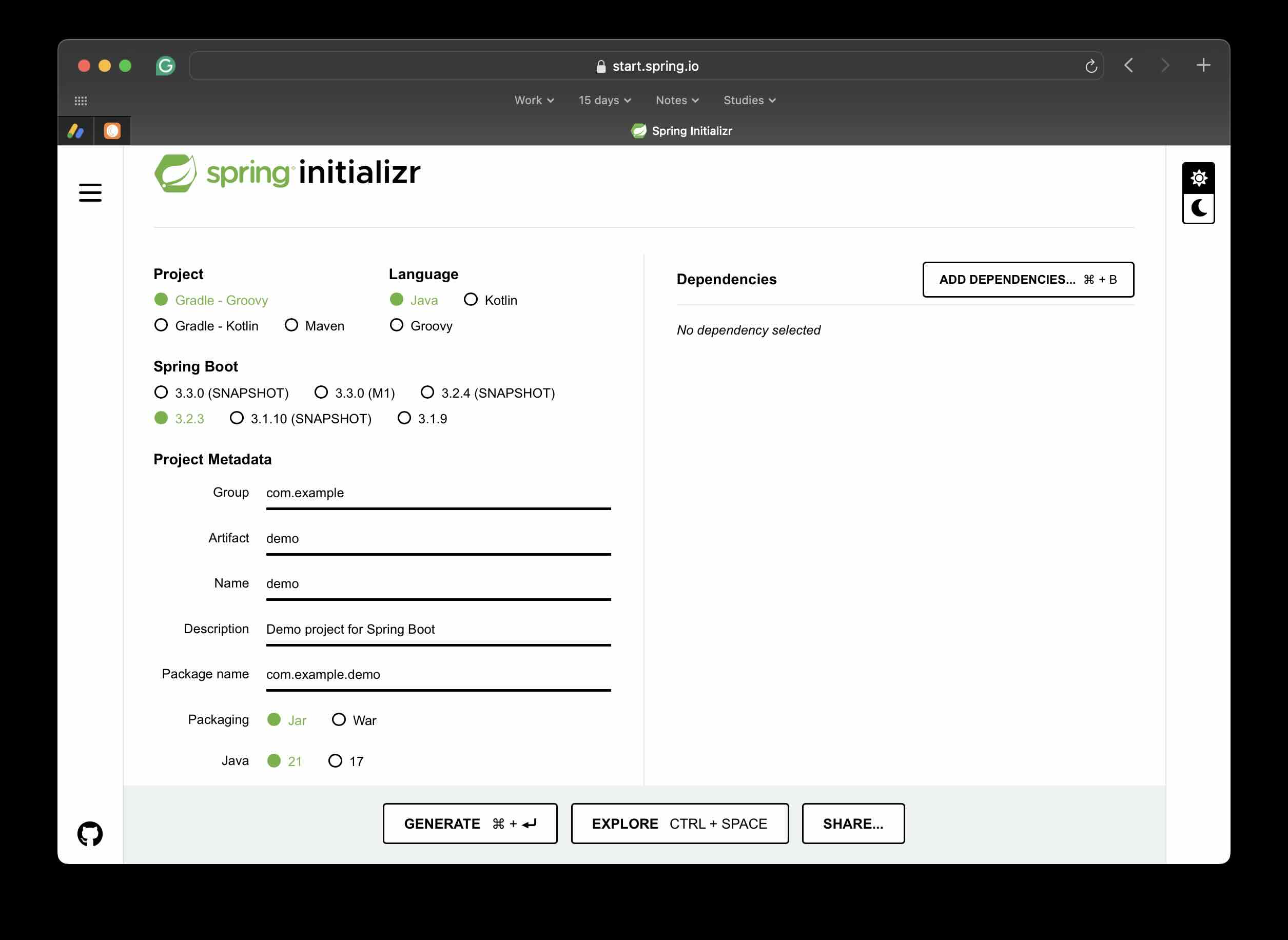
You only need one starter dependency i.e. 'org.springframework.boot:spring-boot-starter'
Build.gradle:dependencies {
implementation 'org.springframework.boot:spring-boot-starter'
}
Maven: pom.xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
</dependencies>
Step 2: Make Application class implement CommandLineRunner
Now all you need to do is open the Application class (one with annotation @SpringBootApplication) and make it implement CommandLineRunner.
Next, you need to override the run() method where you add your logic for your command-line application.
package org.code2care.demo;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
@SpringBootApplication
public class DemoApplication implements CommandLineRunner {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationConfig.xml");
Employee employee = applicationContext.getBean("employee", Employee.class);
}
@Override
public void run(String... args) throws Exception {
System.out.println("This is it!");
}
}
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Fix Generics: error unexpected type required: class found: type parameter - Java
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0 - Java
- How to add a Task List to Quick View Menu in SharePoint Online Site - SharePoint
- Install GitHub Command Line Tool on Mac - Git
- How to Write Code in Windows Notepad - Windows
- Working with Bluetooth on Mac Terminal using blueutil Commands - MacOS
- How to change Android EditText Cursor Color - Android
- Ubuntu: How to set Environment Variable - Ubuntu