To calculate age from the date of birth in Java, you should make use of the preferred java.time.LocalDate class from the new Date and Time API. But we will cover also the older java.util.Date class for legacy code.
Using java.time.LocalDate (Java 8 and above):
Example:package org.code2care.examples;
import java.time.LocalDate;
import java.time.Period;
public class CurrentAgeCalculator {
public static void main(String[] args) {
LocalDate dateOfBirth = LocalDate.of(1992, 12, 25);
int age = calculateCurrentAge(dateOfBirth);
System.out.println("Current Age is " + age);
}
public static int calculateCurrentAge(LocalDate dateOfBirth) {
LocalDate currentDate = LocalDate.now();
Period period = Period.between(dateOfBirth, currentDate);
return period.getYears();
}
}
Output:
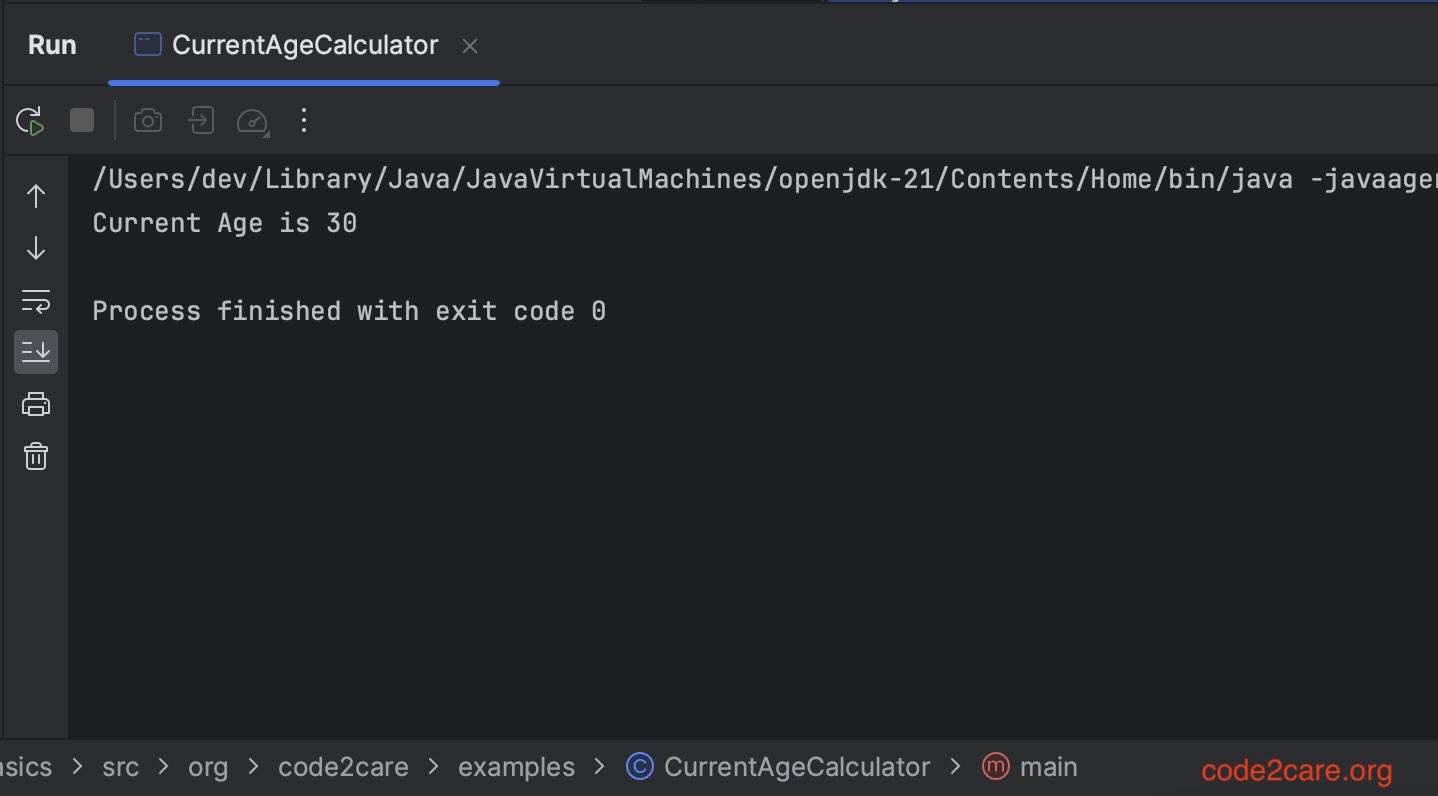
Using java.util.Date (legacy):
Date currentDate = new Date();
long diff = currentDate.getTime() - dateOfBirth.getTime();
long ageInMilliseconds = diff;
int ageInYears = (int) (ageInMilliseconds / (365.25 * 24 * 60 * 60 * 1000));
As you may understand from this code, why Java 8 way is preferred and recommended.
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Where is Cron Crontab jobs log file Located in Ubuntu Linux - Ubuntu
- [fix] Java NullPointerException ComparableTimSort countRunAndMakeAscending when sorting a List - Java
- How to Send Email using Java - Java
- How to remove quotes from a String in Python - Python
- How to know Roblox Version Details on Mac - MacOS
- How to change Chrome Spell Check from UK English to US English - Chrome
- How to Toggle Light/Dark Mode in Google Colab - Google
- Get HTML table td, tr or th inner content value with id or name attribute - Html