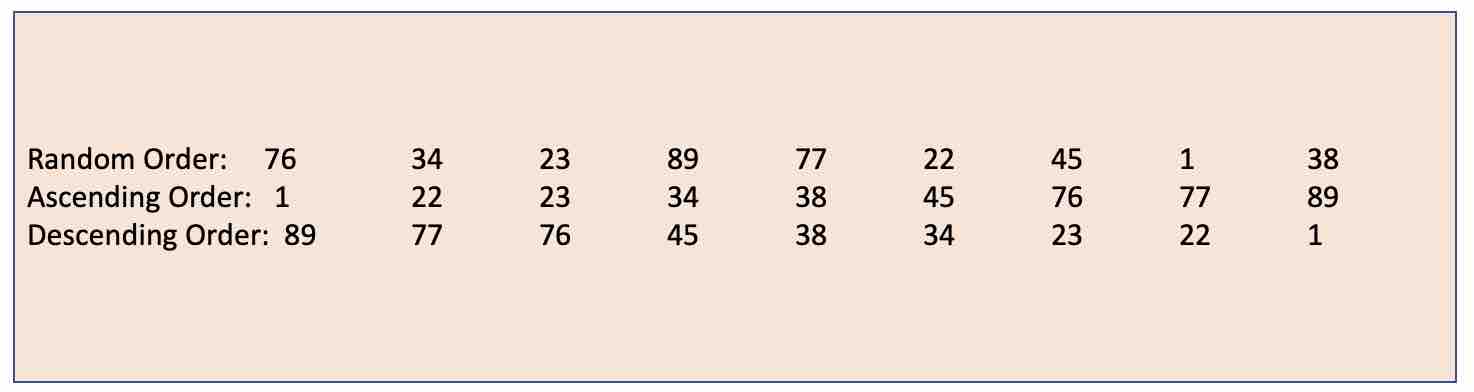
In this program, we will take a look at how you can sort an Array in Java in Ascending or Descending order
First let's create an array with random numbers.
int[] randomNumberArray = {76,34,23,89,77,22,45,1,38};
Sorting in Ascending Order
Now to sort this array of numbers in ascending order we can make use of the Arrays.sort method.
Arrays.sort(randomNumberArray);
Finally let's iterate through the array and print the sorted array values
for (int no: randomNumberArray) {
System.out.print(no +"\t");
}
Sorting in Descending Order
Note that there is no revere function in the Arrays utility class to reverse an Array and make it in descending order. So we have to either use Collections class or write our own logic.
public static void main(String[] args) {
int[] randomNumberArray = {76,34,23,89,77,22,45,1,38};
Arrays.sort(randomNumberArray);
// Reverse the array
for (int i = 0; i < randomNumberArray.length / 2; i++) {
int temp = randomNumberArray[i];
randomNumberArray[i] = randomNumberArray[randomNumberArray.length - i - 1];
randomNumberArray[randomNumberArray.length - i - 1] = temp;
}
for (int no: randomNumberArray) {
System.out.print(no +"\t");
}
}
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Create S3 bucket using AWS CLI Command mb - AWS
- How to resolve Certificate Expired WhatsApp Error - WhatsApp
- [fix] Java Spring Boot JPA SQLSyntaxErrorException: Encountered user at line 1 column 14 - Java
- [Fix] java: incompatible types: incompatible parameter types in lambda expression error - Java
- How to Update Device Drivers on Windows 10/11 Manually - Windows
- Location of eclipse.ini file on Mac OS X - Mac-OS-X
- hibernate.cfg.xml Configuration and Mapping xml Example - Java
- How to delete SNS Topic using AWS CLI - AWS