You might have made use of Toast to display messages that fades in stays for few seconds and fades away. If you want to display some message and let user select the response (Yes or No) then we can make use of Dialog called as AlertDialog.
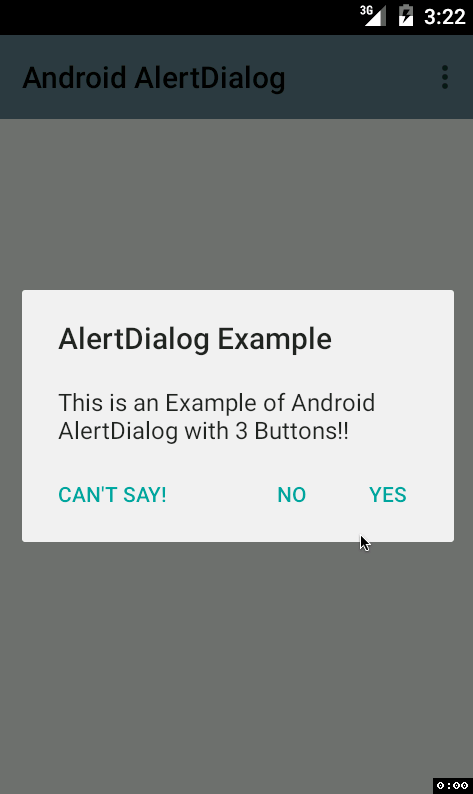
AlertDialog is a subclass of Dialog from android.app package. You can create a dialog with one, two or even three buttons. It is also possible to display only text message using setMessage() method.
There are three functions for adding Buttons to Android Dialog,
setPositiveButton(int textId, DialogInterface.OnClickListener listener) :
This is Yes button, when clicked the code written in the OnClickListener onClick() method will be displayed.
setNegativeButton(int textId, DialogInterface.OnClickListener listener) :
This is just like the setPositiveButton method which acts a "NO" negative button, we will write the logic in OnClickListener anonymous class.
setNeutralButton(int textId, DialogInterface.OnClickListener listener) :
This is how we can set the 3rd button. It is a called the Neutral button.
Android AlertDialog SnippetAlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
builder.setTitle("AlertDialog Example");
builder.setMessage("This is an Example of Android AlertDialog with 3 Buttons!!");
//Button One : Yes
builder.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this, "Yes button Clicked!", Toast.LENGTH_LONG).show();
}
});
//Button Two : No
builder.setNegativeButton("No", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this, "No button Clicked!", Toast.LENGTH_LONG).show();
dialog.cancel();
}
});
//Button Three : Neutral
builder.setNeutralButton("Can't Say!", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this, "Neutral button Clicked!", Toast.LENGTH_LONG).show();
dialog.cancel();
}
});
AlertDialog diag = builder.create();
diag.show();
- Increase Android Emulator Timeout time
- Android : Remove ListView Separator/divider programmatically or using xml property
- Error : Invalid key hash.The key hash does not match any stored key hashes
- How to Change Android Toast Position?
- Android Alert Dialog with Checkboxes example
- Android : No Launcher activity found! Error
- Android Development: Spinners with Example
- Failed to sync Gradle project Error:failed to find target android-23
- INSTALL_FAILED_INSUFFICIENT_STORAGE Android Error
- Disable Fading Edges Scroll Effect Android Views
- How to create Toast messages in Android?
- Channel 50 SMSes received every few minutes Android Phones
- Android xml error Attribute is missing the Android namespace prefix [Solution]
- Create Custom Android AlertDialog
- How To Disable Landscape Mode in Android Application
- Android Development - How to switch between two Activities
- incorrect line ending: found carriage return (\r) without corresponding newline (\n)
- Generate Facebook Android SDK keyhash using java code
- Android Error Generating Final Archive - Debug Certificate Expired
- 21 Useful Android Emulator Short-cut Keyboard Keys
- Android RatingBar Example
- 11 Weeks of Android Online Sessions-15-Jun-to-28-Aug-2020
- Download interrupted: Unknown Host dl-ssl.google.com Error Android SDK Manager
- fill_parent vs match_parent vs wrap_content
- Android : Connection with adb was interrupted 0 attempts have been made to reconnect
- How to check if variable is a number in JavaScript (NaN, typeof, regex) - JavaScript
- PowerShell: How to Get Folder Size - Powershell
- How to install Terraform on M1/M2 Mac - MacOS
- Best way to calculate elapsed time in Java using Java 8 Duration & Instant Class with Nanoseconds precision - Java
- SharePoint Server 2016 setup error - A system restart from a previous installation or update is pending. Restart your computer and run setup to continue. - SharePoint
- How to customize SharePoint Modern list form using JSON formatting - SharePoint
- JavaScript: Convert an Image into Base64 String - JavaScript
- How to find the Length of ArrayList in Java - Java