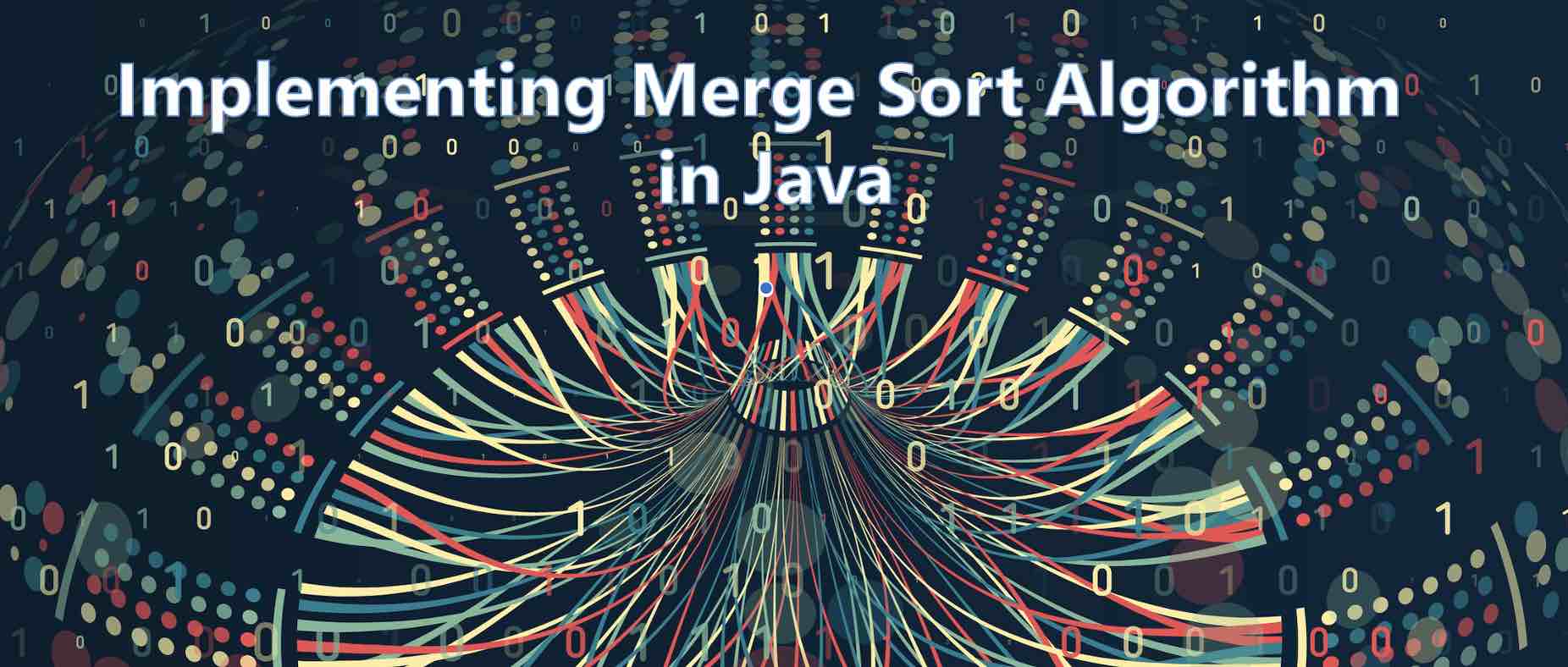
package org.code2care.java.sorting.algo;
import java.util.Arrays;
public class MergeSortJavaExample {
public static void main(String[] args) {
//Unsorted Array
int[] unsortedArray = {4, 99, 2, 11, 34, 67, 54, 12, 45, 245, 234, 12, 200};
MergeSortJavaExample mergeSort = new MergeSortJavaExample();
int[] sortedArray = mergeSort.mergeSortAlgorithm(unsortedArray);
System.out.println(Arrays.toString(sortedArray));
}
public int[] mergeSortAlgorithm(int[] arrayOfNumbers) {
int arrayLength = arrayOfNumbers.length;
if (arrayLength == 1) {
return arrayOfNumbers;
}
//Divide the array into two halves
int midIndex = arrayLength / 2;
int[] leftArray = Arrays.copyOfRange(arrayOfNumbers, 0, midIndex);
int[] rightArray = Arrays.copyOfRange(arrayOfNumbers, midIndex, arrayLength);
leftArray = mergeSortAlgorithm(leftArray);
rightArray = mergeSortAlgorithm(rightArray);
return mergeArrays(leftArray, rightArray);
}
public int[] mergeArrays(int[] leftArray, int[] rightArray) {
int leftLength = leftArray.length;
int rightLength = rightArray.length;
int[] mergedArray = new int[leftLength + rightLength];
int leftIndex = 0, rightIndex = 0, mergedIndex = 0;
while (leftIndex < leftLength && rightIndex < rightLength) {
if (leftArray[leftIndex] <= rightArray[rightIndex]) {
mergedArray[mergedIndex] = leftArray[leftIndex];
leftIndex++;
} else {
mergedArray[mergedIndex] = rightArray[rightIndex];
rightIndex++;
}
mergedIndex++;
}
while (leftIndex < leftLength) {
mergedArray[mergedIndex] = leftArray[leftIndex];
leftIndex++;
mergedIndex++;
}
while (rightIndex < rightLength) {
mergedArray[mergedIndex] = rightArray[rightIndex];
rightIndex++;
mergedIndex++;
}
return mergedArray;
}
}
Output:
Time Complexity | |||||
---|---|---|---|---|---|
Best Case | Average Case | Worst Case | Space Complexity | ||
Merge Sort | Ω(n log n) | Θ(n log n) | O(n log n) | O(n) |
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to install maven in macOS using Terminal Command - MacOS
- How to turn off Automatically adjust brightness on Mac Ventura 13 - MacOS
- What does b prefix before a String mean in Python? - Python
- TextEdit: Disable Autocorrect Option (Mac) - MacOS
- [Fix] ValueError: substring not found in Python - Python
- How to take a Screenshot on iPhone with iOS 17 - iOS
- Connect to 3270 host IBM Mainframe using Mac Terminal (c3270) - MacOS
- ls Command to See Hidden Files - Linux