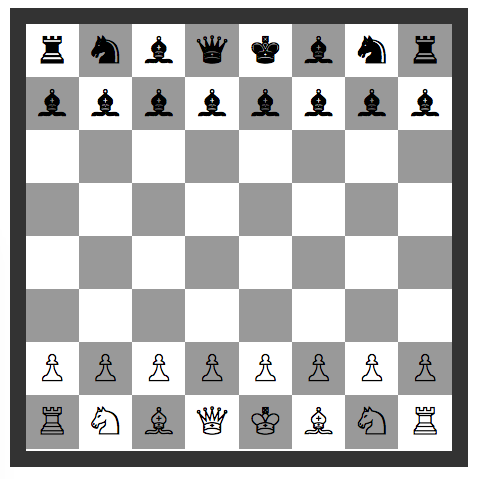
Chessboard using pure css and html
To create a Chessboard with Chess pieces we first need to know their Unicode or HTML equivalent codes.
There are around 12 Symbols that are needed to create a Chessboard in HTML.
These symbols are available in Unicode range U+2654 to U+265F.
⚡️ Note: To display these Symbols you need to have the font that supports this Unicode Range.
1. White Chess King ♔Unicode : U+2654
HTML Code : ♔
2. Black Chess King ♚
Unicode : U+265A
HTML Code : ♚
3. White Chess Queen ♕
Unicode : U+2655
HTML Code : ♕
4. Black Chess Queen ♛
Unicode : U+265B
HTML Code : ♛
5. White Chess Rock ♖
Unicode : U+2656
HTML Code : ♖
6. Black Chess Rock ♜
Unicode : U+265C
HTML Code : ♜
7. White Chess Bishop ♗
Unicode : U+2657
HTML Code : ♗
8. Black Chess Bishop ♝
Unicode : U+265D
HTML Code : ♝
9. White Chess Knight ♘
Unicode : U+2658
HTML Code : ♘
10. Black Chess Knight ♞
Unicode : U+265E
HTML Code : ♞
11. White Chess Pawn ♙
Unicode : U+2659
HTML Code : ♙
12. Black Chess Pawn ♟
Unicode : U+265F
HTML Code : ♟
Source Code HTML + CSS
<html>
<head>
<meta charset="UTF-8">
<title>Chessboard using Pure CSS and HTML</title>
<style type="text/css">
.chessboard {
width: 640px;
height: 640px;
margin: 20px;
border: 25px solid #333;
}
.black {
float: left;
width: 80px;
height: 80px;
background-color: #999;
font-size:50px;
text-align:center;
display: table-cell;
vertical-align:middle;
}
.white {
float: left;
width: 80px;
height: 80px;
background-color: #fff;
font-size:50px;
text-align:center;
display: table-cell;
vertical-align:middle;
}
</style>
</head>
<body>
<div class="chessboard">
<!-- 1st -->
<div class="white">♜</div>
<div class="black">♞</div>
<div class="white">♝</div>
<div class="black">♛</div>
<div class="white">♚</div>
<div class="black">♝</div>
<div class="white">♞</div>
<div class="black">♜</div>
<!-- 2nd -->
<div class="black">♝</div>
<div class="white">♝</div>
<div class="black">♝</div>
<div class="white">♝</div>
<div class="black">♝</div>
<div class="white">♝</div>
<div class="black">♝</div>
<div class="white">♝</div>
<!-- 3th -->
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<!-- 4st -->
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<!-- 5th -->
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<!-- 6th -->
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
<!-- 7th -->
<div class="white">♙</div>
<div class="black">♙</div>
<div class="white">♙</div>
<div class="black">♙</div>
<div class="white">♙</div>
<div class="black">♙</div>
<div class="white">♙</div>
<div class="black">♙</div>
<!-- 8th -->
<div class="black">♖</div>
<div class="white">♘</div>
<div class="black">♗</div>
<div class="white">♕</div>
<div class="black">♔</div>
<div class="white">♗</div>
<div class="black">♘</div>
<div class="white">♖</div>
</div>
</body>
</html>
Note: You can simply use the Unicode pieces within your Html code provided your page supports UTF-8 Character set. To do so just include <meta charset="UTF-8" > in the <head> section of your webpage.
More Posts related to Html,
- How to remove old 404 pages ulrs from Google crawler
- W3 HTML validator warning Unable to Determine Parse Mode
- Comprehensive 256 Ascii code table with Html Hex IBM Microsoft Key
- Align html element at the center of page vertically and horizontally
- HTML Images - Attributes and Formats
- 9 Border to DIV Element in HTML Examples
- Create HTML button that looks like a href hyperlink
- What is the doctype for HTML5?
- Simple Crossword Puzzle example using Pure HTML, CSS and JavaScript
- How to make a div tag clickable
- The author stylesheet specified in tag script is too long - document contains 21759 bytes whereas the limit is 10000 bytes
- W3 : character data is not allowed here html validation error
- How to set background color in HTML page?
- Auto Refresh Webpage after every x Second or Minute using Meta Tag?
- Default speed of Marquee tag : SCROLLAMOUNT
- Get HTML table td, tr or th inner content value with id or name attribute
- How to add multiple spaces between html page text
- Chessboard with pieces using pure HTML and CSS
- How to turn off autocomplete in input fields in HTML Form
- remove div vertical scroll
- Fibonacci series from 1 to 500 table
- Remove Html head and body tags from ckeditor source
- reCaptcha Verification expired. Check the checkbox again
- HTML5 CSS3 Color Codes List
- All directional arrows codes for HTML
More Posts:
- Json Serialization and Deserialization using Java Jackson - Java
- Capture cURL Request Output to a File - cURL
- How to Open a New Notebook in Google Colab - Google
- Share Image to WhatsApp with Caption from your Android App - WhatsApp
- Install OpenJDK Java (8/11/17) versions using brew on Mac (Intel/M1/M2) - MacOS
- Android App Showing Two Toolbars Issue fix - Android
- Float built-in function in Python - Python
- Get Absolute Relative Path of File in IDEA IntelliJ - Java