There is a list of Special Parameters or Variables that are available when working with Bash Shell that can be used with scripts or command line arguments.
Bash Parameter | Description | Usage |
---|---|---|
$0 |
Name of the script or the shell | To Identify the script or shell that is currently running |
$1 , $2 , $3 , ... |
Positional parameters or arguments passed to the script | To access individual command-line arguments |
$* |
All positional parameters as a single string | To represent all command-line arguments as a single string |
$@ |
All positional parameters as separate strings | To represent all command-line arguments as separate strings |
$# |
Number of positional parameters | To count the total number of command-line arguments |
$? |
Exit status of the last executed command | To indicate the success or failure of the last command |
$$ |
Process ID (PID) of the current script or shell | To represent the unique identifier of the running process |
$! |
Process ID (PID) of the last background command | To store the PID of the most recent background process |
$- |
Current options set for the shell | To show the currently active options for the shell |
$IFS |
Internal Field Separator | To determine how Bash splits words into fields |
$PWD |
Current working directory | To represent the directory in which the script is running |
$OLDPWD |
Previous working directory | To hold the path of the previous working directory |
$HOME |
Home directory of the current user | To point to the user's home directory |
$USER |
Username of the current user | To store the name of the user executing the script |
$HOSTNAME |
Hostname of the system | To represent the name of the system |
$RANDOM |
A random number | To provide a random number each time it is accessed |
Let's see examples of each of them
#!/bin/bash
# Special Parameters Example Script
# $0 - Name of the script or the shell
echo "Script name: $0"
# $1, $2, $3, ... - Positional parameters or arguments passed to the script
echo "First argument: $1"
echo "Second argument: $2"
echo "Third argument: $3"
# $* - All positional parameters as a single string
echo "All arguments as a single string: $*"
# $@ - All positional parameters as separate strings
echo "All arguments as separate strings:"
for arg in "$@"; do
echo "$arg"
done
# $# - Number of positional parameters
echo "Total number of arguments: $#"
# $? - Exit status of the last executed command
echo "Exit status of last command: $?"
# $$ - Process ID (PID) of the current script or shell
echo "Process ID: $$"
# $! - Process ID (PID) of the last background command
echo "Process ID of last background command: $!"
# $- - Current options set for the shell
echo "Current options: $-"
# $IFS - Internal Field Separator
echo "Internal Field Separator: $IFS"
# $PWD - Current working directory
echo "Current working directory: $PWD"
# $OLDPWD - Previous working directory
echo "Previous working directory: $OLDPWD"
# $HOME - Home directory of the current user
echo "Home directory: $HOME"
# $USER - Username of the current user
echo "Username: $USER"
# $HOSTNAME - Hostname of the system
echo "Hostname: $HOSTNAME"
# $RANDOM - A random number
echo "Random number: $RANDOM"
Output:
./special_parameters_example.sh
Script name: ./special_parameters_example.sh
First argument: hello
Second argument: 1
Third argument: 2
All arguments as a single string: hello 1 2 3
All arguments as separate strings:
hello
1
2
3
Total number of arguments: 4
Exit status of last command: 0
Process ID: 4662
Process ID of last background command:
Current options: hB
Internal Field Separator:
Current working directory: /Users/c2ctech/Desktop
Previous working directory:
Home directory: /Users/c2ctech
Username: c2ctech
Hostname: C2C-Tech-Mac.local
Random number: 14105
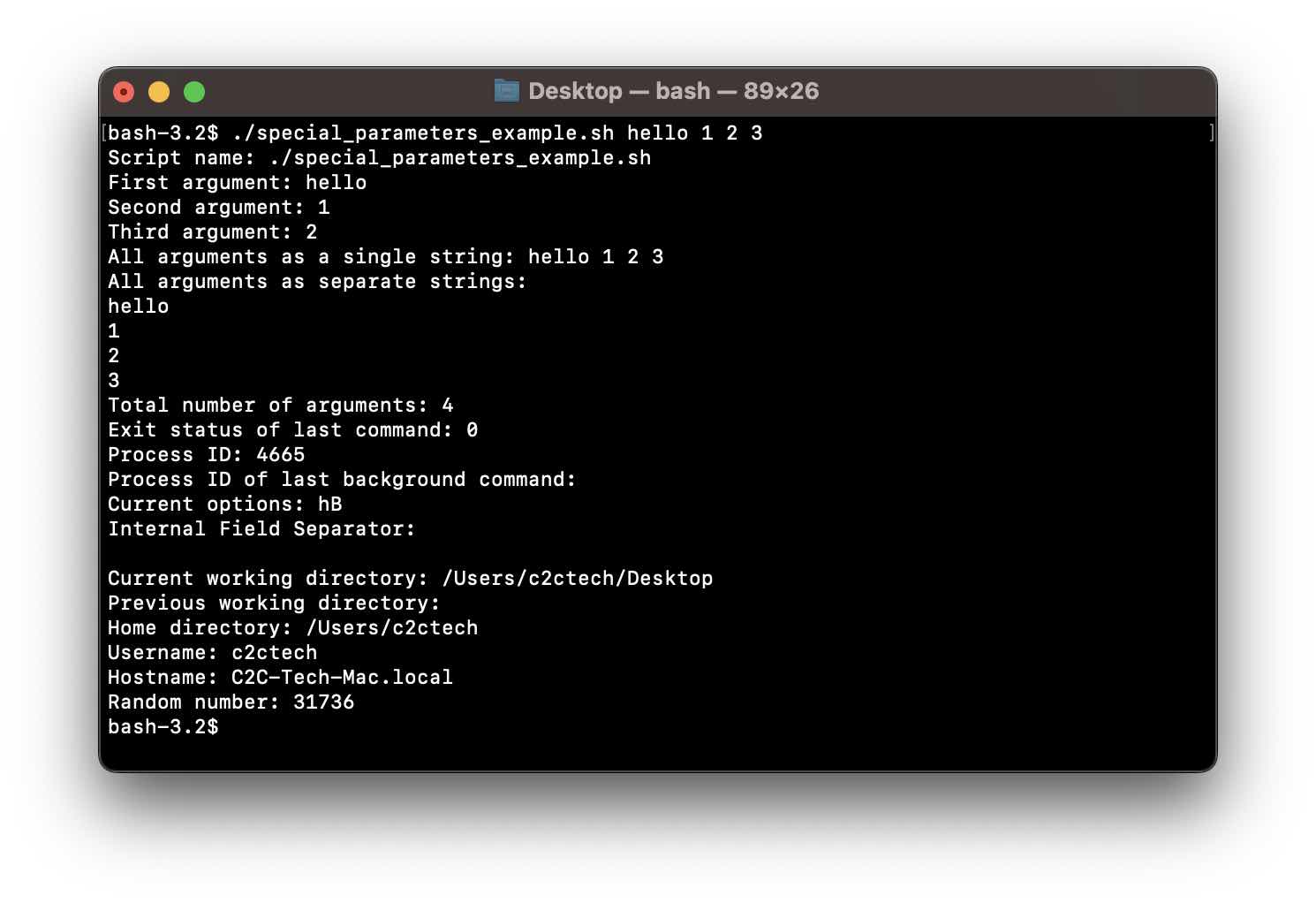
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Linux,
- Command to know the Available Memory on Linux System
- How to install curl on Alpine Linux
- How to backup a file in Linux/Unix
- Install Java Runtime Environment (Oracle or open JRE) on Ubuntu
- What is the Default Admin user and Password for Jenkins
- How to tar.gz a directory or folder Command
- Copy entire directory using Terminal Command [Linux, Mac, Bash]
- Fix: bash: ipconfig: command not found on Linux
- Command to check Last Login or Reboot History of Users and TTYs
- Linux: Create a New User and Password and Login Example
- ls command to list only directories
- bash: cls: command not found
- How to exit from nano command
- Installing and using unzip Command to unzip a zip file using Terminal
- What does apt-get update command does?
- ls command: sort files by name alphabetically A-Z or Z-A [Linux/Unix/macOS/Bash]
- How to remove or uninstall Java from Ubuntu using apt-get
- scp: ssh: connect to host xxxx port 22: Connection refused Error
- Sort ls command by last modified date and time
- Create Nested Directories using mkdir Command
- How to Exit a File in Terminal (Bash/Zsh)
- Command to know the installed Debian version?
- How to connect to SSH port other than default 22
- How to save a file in Nano Editor and Exit
- Install OpenSSL on Linux/Ubuntu
More Posts:
- How to Make a Windows Notepad File Read-Only - Windows
- Fix: Notepad++ bottom status bar not visible - NotepadPlusPlus
- Convert Collection List to Set using Java 8 Stream API - Java
- Android Studio Native typeface cannot be made error - Android
- How to install Jupyter Notebook on macOS Sonoma - MacOS
- How to create classic site in SharePoint Online - SharePoint
- Connect to 3270 host IBM Mainframe using Mac Terminal (c3270) - MacOS
- Copy file from a remote server to current local directory system using SCP command - HowTos