- In Eclipse, go to File -> New -> Other...
- Now Select Maven -> Maven Project
- Click Next,
- Select "Create a simple project (Skip archetype selection)", Next
- Enter Group Id and Artifact Id and click Finish.
- Now open the pom.xml and add the following dependency for Junit 4 under dependencies,
<dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.8.1</version> <scope>test</scope> </dependency> </dependencies>
- Create a java file,
package myprog; public class Addition { public int add (int no1, int no2) { return no1 + no2; } }
- Now write a text class for the class we created under src/test/java package,
package myprog; import static org.junit.Assert.assertEquals; import org.junit.Test; public class AdditionTest { @Test public void addTwoNumbers() { Addition addition = new Addition(); assertEquals(5, addition.add(2, 3)); } }
Now let's run the maven test command to run the test cases, simply right click on your project folder and select Run As -> Maven test
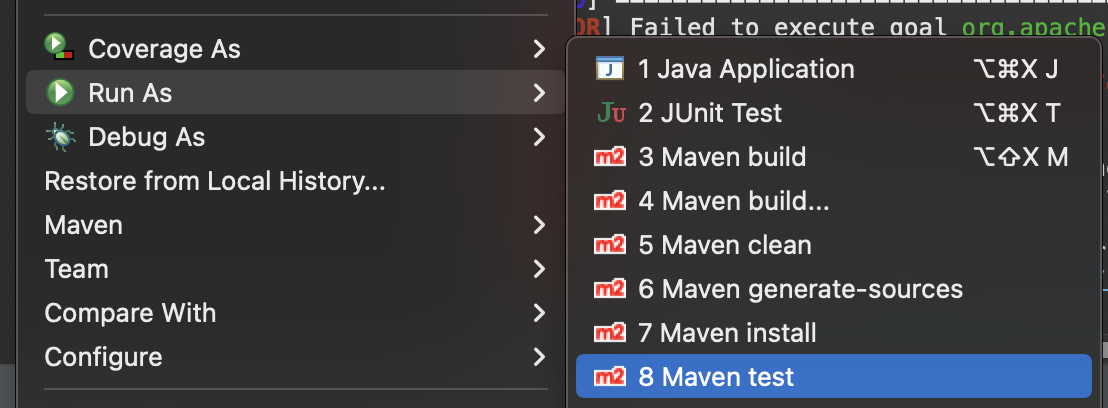

Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- JSON Text to JavaScript Object using eval() Example: JSON Tutorial - Json-Tutorial
- How to Pretty Print JSON in PHP - PHP
- Notepad++ Search Across Multiple Lines - NotepadPlusPlus
- [Fix] ./gradlew: Permission denied - Gradle
- How to place two div elements next to each other - CSS
- Shortcut to Open Snipping Tool on Windows and Take Screenshot - Windows
- Obsolete marquee element alternatives html5 - Html
- Quick way to setup AWS DynamoDB locally on macOS - AWS