The Object class from the java.util package is a container object that may or may not contain a non-null value. If a value is present then the isPresent() method returns true.
If you want to set a default value for the Optional object, then you can make use of the orElse() method with a default value passed as an argument to it.
Let's take a look at an example.
import java.util.Optional;
public class Example {
public static void main(String[] args) {
Optional<String> optionalWithValue = Optional.of("Mike");
Optional<String> optionalEmpty = Optional.empty(); // empty
Optional<String> optionalNull = Optional.ofNullable(null); // null
String value1 = optionalWithValue.orElse("No Name");
String value2 = optionalEmpty.orElse("Default Value");
String value3 = optionalNull.orElse("0");
System.out.println("Value 1: " + value1); // Output: Value 1: Mike
System.out.println("Value 2: " + value2); // Output: Value 2: Default Value
System.out.println("Value 3: " + value3); // Output: Value 3: 0
}
}
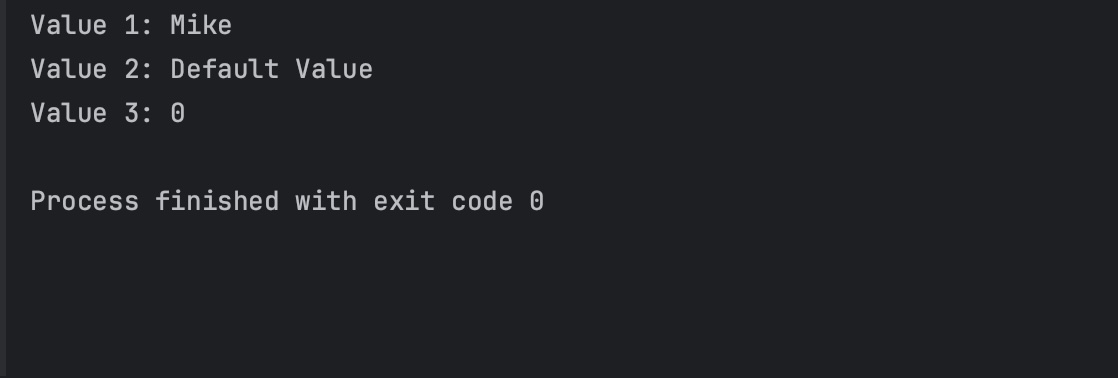
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Check Bluetooth is turned on or off on Android device programmatically [Java Code] - Android
- How to Subtract Days From Date in Java 8 and Above - Java
- Best Free Gif screen capture app now available for M1 Chip Mac - LICECap - MacOS
- How to check if a Command Exists using Bash Script - Bash
- Change Current User Password using Mac Terminal Command - MacOS
- Install SonarLint on Visual Studio Code - HowTos
- Change default language highlighting in Notepad++ - NotepadPlusPlus
- How to Sort a LinkedList in Java - Java