At times you may want to know what is the name of the Class for a runtime Object in Java, in such case make use of the getClass() method from the java.lang.Object Class.
Let's take a look at some examples:
Example:
-
Employee.java
package org.example;
public class Employee {
private int employeeId;
private String employeeName;
public Employee(int employeeId, String employeeName) {
this.employeeId = employeeId;
this.employeeName = employeeName;
}
public int getEmployeeId() {
return employeeId;
}
public void setEmployeeId(int employeeId) {
this.employeeId = employeeId;
}
public String getEmployeeName() {
return employeeName;
}
public void setEmployeeName(String employeeName) {
this.employeeName = employeeName;
}
}
Main.java
1. package org.example;
2.
3. public class Main {
4.
5. public static void main(String[] args) {
6.
7. Employee emp = new Employee(1,"Mikey");
8. Class empClass = emp.getClass(); //get the class for the runtime object emp
9.
10. System.out.println("Class Name: "+ empClass.getSimpleName());
11. System.out.println("Class Name as Classpath: " + empClass.getName());
12. System.out.println("Package Name: "+ empClass.getPackage());
13.
14. }
15. }
Output:
Employee
org.example.Employee
package org.example
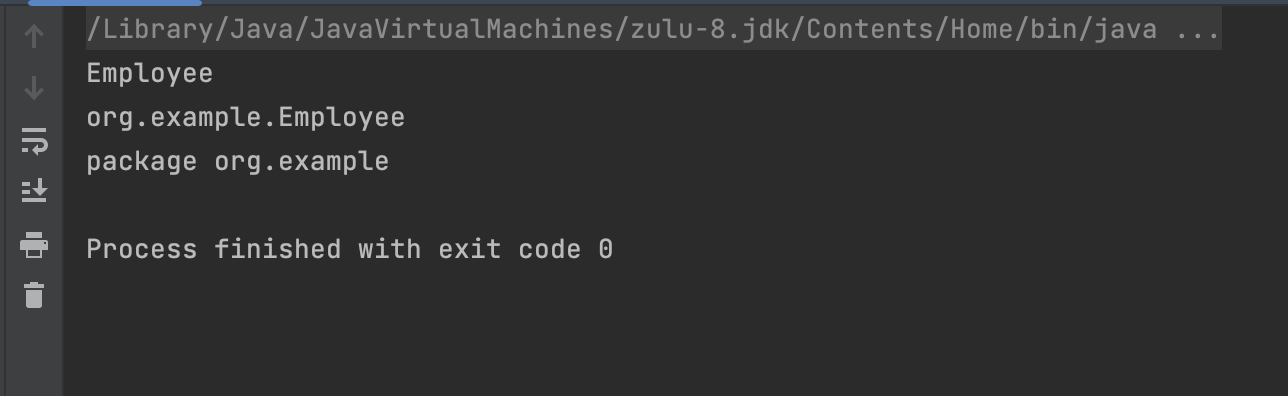
As you may see on line number 8, we have made use of the getClass() method from the Object class to return the runtime class of this Object emp.
Next on line 10, we have made use of the reflection method getSimpleName() just to print the name of the Class (note: this does work for class, interface, array class, primitive type, or void)
On line 11, getName() returns the Class name and the path of the class (classpath) as a response String.
On line 12, getPackage() returns the Package name of the class Employee.
Let us take a look at some more examples:
package org.example;
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> countries = new ArrayList<>();
countries.add("USA");
countries.add("Canada");
countries.add("France");
countries.add("Sweden");
System.out.println(countries.getClass());
System.out.println(countries.get(2).getClass());
}
}
Output:
class java.util.ArrayList
class java.lang.String
Note: The Class.java class has toString() method overridden so you can simply use getClass to print out the class/interface name.
toString method implementation of Class.java file: public String toString() {
return (isInterface() ? "interface " : (isPrimitive() ? "" : "class "))
+ getName();
}
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Install Node on Mac Ventura 13 - MacOS
- Correct way to Get the Current Date in Java 8 or above - Java
- How to create a dictionary comprehension in Python - Python
- Python Switch-Case Statement equivalent like Java Example - Python
- How to Check Installed Python version in Windows, Linux & macOS - Python
- ls command: sort files by name alphabetically A-Z or Z-A [Linux/Unix/macOS/Bash] - Linux
- Device not compatible error Android Google Play Store - Android
- How to install and Configure sar sysstat tools in Ubuntu Linux - Linux