In Java flatmap() is a function in the Stream class that can be used to flatten a Stream of Collections into a single Stream of Objects.
βοΈNote: flatmap() is available only since Java 1.8
Example 1: Concat a 2-Dimensional Integer Array into a single 1-Dimentaional Array
import java.util.Arrays;
import java.util.stream.Stream;
public class JavaFlatMapExample {
public static void main(String[] args) {
Integer[][] array2D = new Integer[][]{{100, 200,300}, {400,500,600}, {700, 800}};
System.out.println(Arrays.deepToString(array2D));
Stream<Integer[]> streamOf2DArray = Arrays.stream(array2D);
Integer[] array1D = streamOf2DArray.flatMap(Stream::of).toArray(Integer[]::new);
System.out.println(Arrays.toString(array1D));
}
}
Output:
Input: [[100, 200, 300], [400, 500, 600], [700, 800]]
Result: [100, 200, 300, 400, 500, 600, 700, 800]
Example 2: Concat a 2-Dimensional String Array into a single 1-Dimentaional Array
public static void main(String[] args) {
String[][] array2D = new String[][]{{"one", "two"}, {"three","four","five"}, {"six", "seven","eight","nine","ten"}};
System.out.println(Arrays.deepToString(array2D));
Stream<String[]> streamOf2DArray = Arrays.stream(array2D);
String[] array1D = streamOf2DArray.flatMap(Stream::of).toArray(String[]::new);
System.out.println(Arrays.toString(array1D));
}
Output:
Input: [[one, two], [three, four, five], [six, seven, eight, nine, ten]]
Result: [one, two, three, four, five, six, seven, eight, nine, ten]
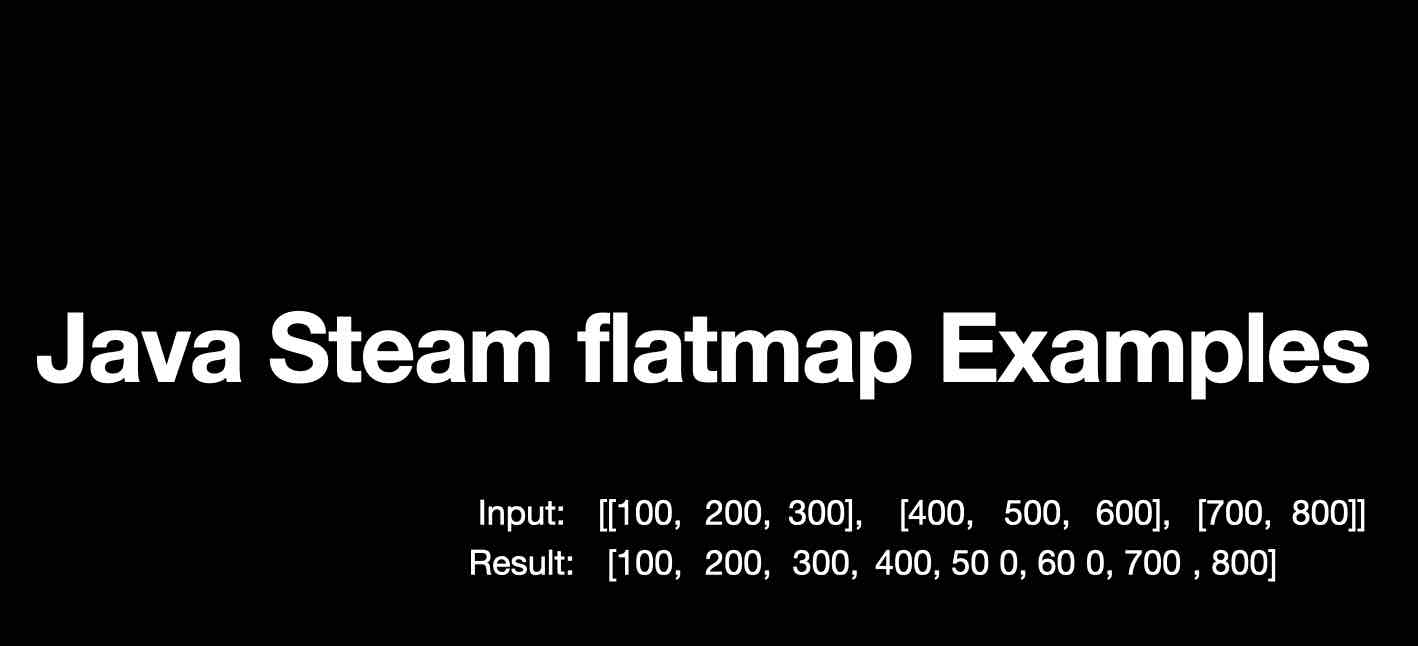
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- eclipse maven m2e : Cannot complete the install - Eclipse
- M365 service Europe outage - AADSTS90033 A transient error has occurred. Please try again. - Microsoft
- How to install Node using Brew on Mac - MacOS
- Closest Alternate to Notepad on Mac - MacOS
- Java Code to check if Twitter app is installed on Android device - Android
- List of PowerShell Cmdlet Commands for Mac - Powershell
- Compare two lists in Python and return matches - Python
- How to turn off autocomplete in input fields in HTML Form - Html