In Java, the Stream class was introduced as a part of the Java 8 Streams API that provides a functional way of processing collections of objects, i.e. a sequence of elements that can be processed in a functional manner.
With the help of the Stream class, you can perform various operations on collections like filtering, mapping, sorting, reducing, and more, without modifying the original collection.
Convert Map<K,V> as List<E>
To convert a Map to a List in Java 8 using Stream,
Step 1: We can use the entrySet() method of the Map interface to get a set of the map's key-value pairs.
Step 2: We can then use the stream() method to convert the set to a stream,
Step 3: We use the map() method to transform each entry into an object of your desired type
Step 4: Lastly, we can make use of the collect() method to collect the stream into a List.
Example:package org.example;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
/**
*
* Code Example in Java 8 to convert
* Map to List using Stream API
*
*
*/
public class MapToList {
public static void main(String[] args) {
Map<String,String> cityTempMap = new HashMap<>();
cityTempMap.put("NYC","34");
cityTempMap.put("Chicago","32");
cityTempMap.put("Austin","42");
cityTempMap.put("Huston","31");
cityTempMap.put("Ohio","30");
//Map to List
List<String> cities = cityTempMap
.entrySet() //Step 1:entrySet() to get K-V Pair
.stream() //Step 2: Convert Set to Stream
.map(entry -> entry.getKey()) // Step 3: Use map() to transform
.collect(Collectors.toList()); // Step 4: Collect Stream as List
System.out.println(cities);
}
}
Output:
[Chicago, NYC, Ohio, Austin, Huston]
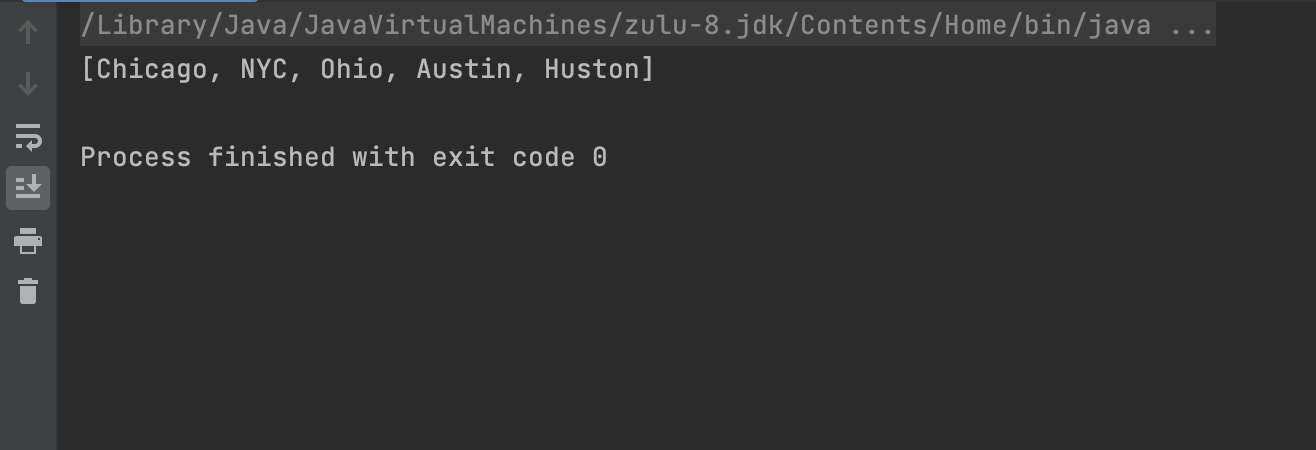
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Java Generics explained with simple definition and examples - Java
- Perform Basic Authentication using cURL with Examples - cURL
- Android Studio emulator/Device logCat logs not displayed - Android-Studio
- [Solution] Exception in thread main java.util.EmptyStackException - Java
- Call a Stored Procedure using Java JDBC CallableStatement Example - Java
- Not receiving email notification alert in SharePoint Online workflow - Power Automate, FLOW - SharePoint
- Change Font Size in Visual Studio Code - HowTos
- List of All 35 Reserved Keywords in Python Programming Language 3.11 - Python