To add hours or minutes to the Java Instant Class object we can make use of the plus methods from the Instant class.
Example:import java.time.Instant;
import java.time.temporal.ChronoUnit;
public class JavaInstantClassExample {
public static void main(String[] args) {
int hoursToAdd = 4;
int minutesToAdd = 30;
Instant currentTimeStampInUTC = Instant.now();
System.out.println("Current Time: "+currentTimeStampInUTC);
System.out.println("Adding 4 hours and 30 minutes to current time");
Instant laterTime = currentTimeStampInUTC.plus(hoursToAdd,ChronoUnit.HOURS)
.plus(minutesToAdd,ChronoUnit.MINUTES);
System.out.println("Later Time: "+laterTime);
}
}
Output:
Current Time: 2022-05-13T18:37:55.991272Z
Adding 4 hours and 30 minutes to current time
Later Time: 2022-05-13T23:07:55.991272Z
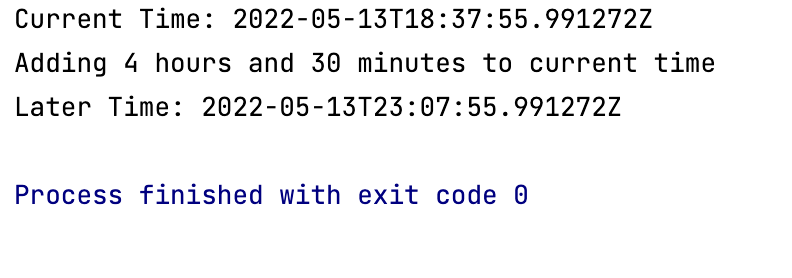
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to Download Jira App for Mac - Jira
- Multiple Microsoft 365 Services Down (Outlook, Teams, SharePoint, OneDrive) - 25 January 2023 (Solved) - Microsoft
- Power BI error Something went wrong, unable to read the application metadata - Microsoft
- [Fix] MySQL ERROR 1054 (42S22): Unknown Column - MySQL
- How to add Back Button on Toolbar in Android [Tutorial] - Android
- Java: How to convert a file to String - Java
- How to migrate SharePoint Designer 2010 workflow to Power Automate FLOW (Microsoft Office 365) - SharePoint
- Microsoft Sign-in Error Code: 50058 (Request Id, Correlation Id and Timestamp) - Microsoft