The one word answer to this question is "yes!" constructors in java can be overloaded.
Let us see this by a code example:
public class Employee {
private int empId;
private String empName;
private int empAge;
public Employee(int empId) {
this.empId = empId;
}
public Employee(String empName) {
this.empName = empName;
}
public Employee(int empId, String empName, int empAge) {
this.empId = empId;
this.empName = empName;
this.empAge = empAge;
}
public Employee() {
//default constructor
}
public static void main(String[] args) {
//default constructor call
Employee employee = new Employee();
//overloaded constructor calls
Employee employee1 = new Employee(1);
Employee employee2 = new Employee("Sam");
Employee employee3 = new Employee(1,"Sam",22);
}
}
As you may see we have defined multiple constructors, in the above code. Note there are some rules that you should remember, as they may come up as follow-up questions in the interview.
Some Rules to remember:
- Overloaded constructors must have different parameters.
- Each overloaded constructor should have a unique signature.
- If a constructor is defined by you, Java does not provide a default constructor, you have to explicitly add it by yourself.
- The order of constructor definition does not matter.
- Constructors are invoked based on argument types or the number of arguments during object creation.
- Constructors can call other constructors using this() for chaining.
- Constructor overloading is specific to object creation, while method overloading involves multiple methods in a class do not get confused.
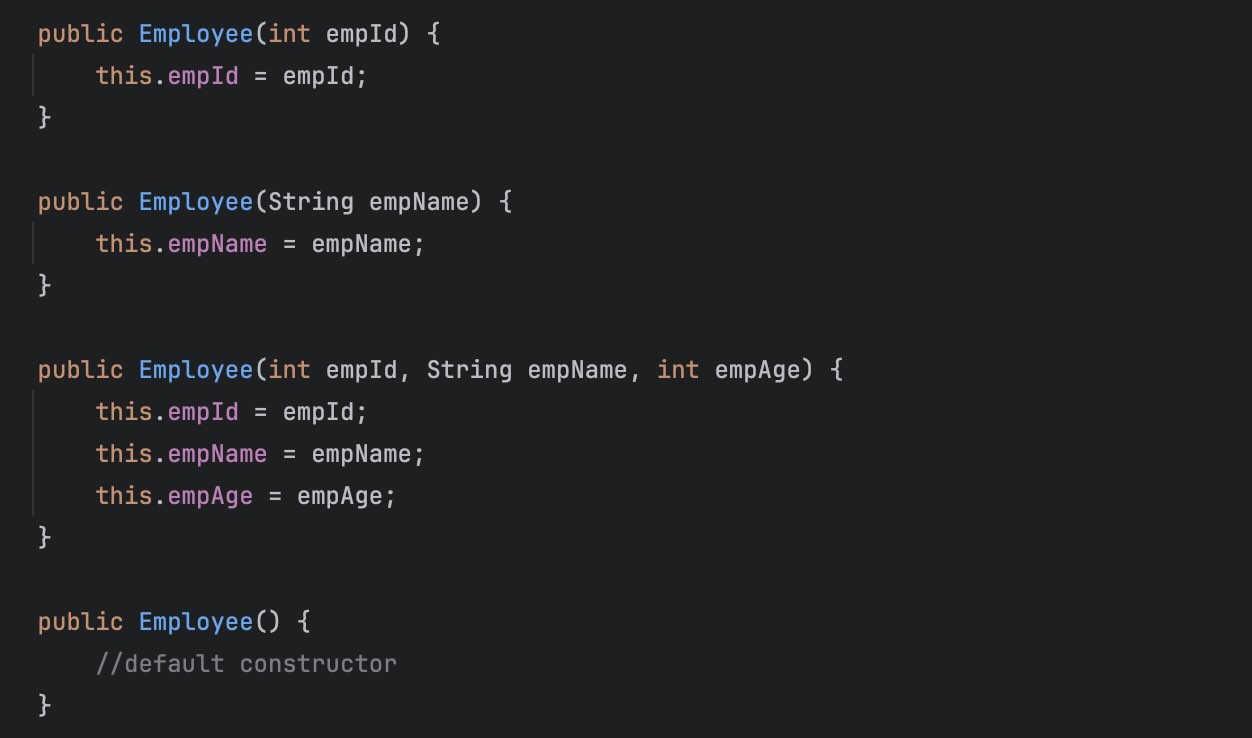
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to Update Microsoft Teams to the latest Version - Teams
- Perform Find and Replace in Nano Text Editor - Linux
- How to Display Current Date and Time in UTC/GMT in Mac Terminal - MacOS
- Bash Command to Get Absolute Path for a File - Bash
- PowerShell 1..10 foreach Example - Powershell
- Solution: AWS S3 CLI Command AccessDenied - S3
- [Fix] Cannot resolve No versions available for org.osgi.service:org.osgi.service.prefs:jar:[1.1.0,1.2.0) within specified range - Java
- How to Stop/Cancel/kill docker image pull - Docker