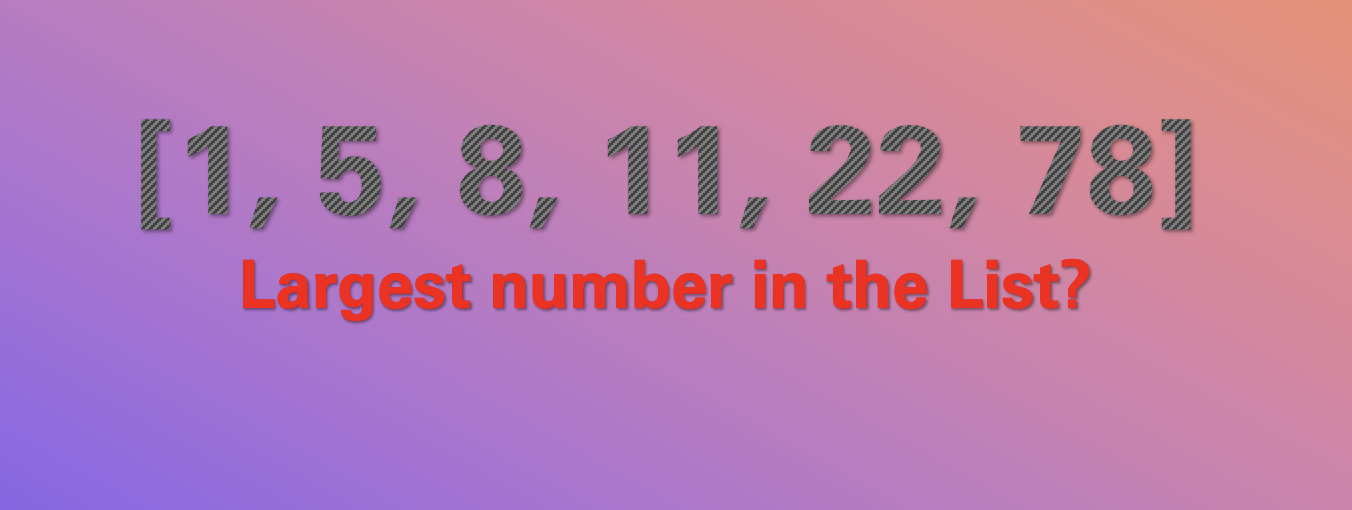
In this program, we will see how to find the largest element in a list of numbers.
Pseudo Logic:
- We take a list of numbers as input from the user.
- Next we initialize a variable max_number to the first element of the list.
- Then we traverse through the list using a loop.
- Next we, compare each element of the list with the max_number variable.
- If the element is greater than max_number, we update the max_number
variable with the new value.
- After traversing the entire list, we print the value of max_number
as this is the max number we were looking for.
Python Code
# Python Program no. 16
# Find Largest Number from List
# 1000+ Python Programs by Code2care.org
# User input as List
input_numers_list = list(map(int, input("Enter a List of Numbers to find Largest: ").split()))
# Let's assume 1st number is max
max_number = input_numers_list[0]
# Traverse the List
for num in input_numers_list:
if num > max_number:
max_number = num
# Print result
print("The Largest Number in the List is:", max_number)
Sample Run Result:
Enter a List of Numbers to find Largest: 19 8 2 4 11 44
The Largest Number in the List is: 44
Python Methods/Concepts used:
- List - to store the elements of the list. Python Doc: https://docs.python.org/3/tutorial/introduction.html#lists
- map() function - to convert the input string to a list of integers. Python Doc: https://docs.python.org/3/library/functions.html#map
- split() method - to split the input string into a list of substrings. Python Doc: https://docs.python.org/3/library/stdtypes.html#str.split
- for loop - to traverse through the list. Python Doc: https://docs.python.org/3/reference/compound_stmts.html#for
- if statement - to compare each element with the max_num variable. Python Doc: https://docs.python.org/3/reference/compound_stmts.html#if
- Program 5: Find Sum of Two Integer Numbers - 1000+ Python Programs
- 34: Traverse a List in Reverse Order - 1000+ Python Programming
- 22: Send Yahoo! Email using smtplib - SMTP protocol client using Python Program
- 35: Python Program to find the System Hostname
- 27: Measure Elapsed Time for a Python Program Execution
- Program 7: Find Difference of Two Numbers - 1000+ Python Programs
- Program 12: Calculate Area and Circumference of Circle - 1000+ Python Programs
- Program 9: Divide Two Numbers - 1000+ Python Programs
- Program 2: Print your name using print() function - 1000+ Python Programs
- 25: How to rename a file using Python Program
- 17: Find Factorial of a Number - 1000+ Python Programs
- Program 6: Find Sum of Two Floating Numbers - 1000+ Python Programs
- 23: Python Programs to concatenate two Lists
- 36: Python Program Convert Hex String to Integer
- 20 - Python - Print Colors for Text in Terminal - 1000+ Python Programs
- Python Program: Use NumPy to generate a random number between 0 and 1
- 32: Python Program to Find Square Root of a Number
- Program 8: Multiply Two Numbers - 1000+ Python Programs
- Program 11: Calculate Percentage - 1000+ Python Programs
- 18: Get Sub List By Slicing a Python List - 1000+ Python Programs
- 28: Program to Lowercase a String in Python
- Program 1: Print Hello World! - 1000+ Python Programs
- 21: Program to Delete File or Folder in Python
- 29: Program to convert Python dict to dataframe
- 33: Python Program to find the current time in India (IST)
- Connect Azure AD (Active Directory) for PowerShell - Powershell
- [Solution] POI: Cannot get a NUMERIC value from a STRING cell - Java
- Java: Round Up to 2 decimal places examples - Java
- How to know the version of OpenSSL - HowTos
- Check Internet Connection WIFI 4G is active on Android Programmatically - Android
- Eclipse : The type java.lang.CharSequence cannot be resolved. Indirectly referenced from required .class files - Java
- How to show Videos on SharePoint Page - SharePoint
- How to Customize Ribbon in Excel for Mac - MacOS
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python-Programs,
More Posts: