If you have a string and you want to extract numbers from it as a list of integers, you can make use of the re module (regular expressions)
Let us take a look at some examples.
Example 1:
Firstly we need to import the re module.
import re
Let's say we have a string with marks for each subject.
marks_string = "Math: 95, Science: 88, English: 92, History: 87"
We can make use of re.findall() method to extract all numbers (d+) as a list of numbers as strings.
marks_list = re.findall(r'\d+', marks_string)
If you want the numbers to be a list of int then you can do that by the below step.
marks_list_int = [int(mark) for mark in marks_list]
Let's take a look at the complete code in action.
Complete code:import re
marks_string = "Math: 95, Science: 88, English: 92, History: 87"
marks_list = re.findall(r'\d+', marks_string)
marks_list_int = [int(mark) for mark in marks_list]
print(marks_list)
Output:
[95, 88, 92, 87]
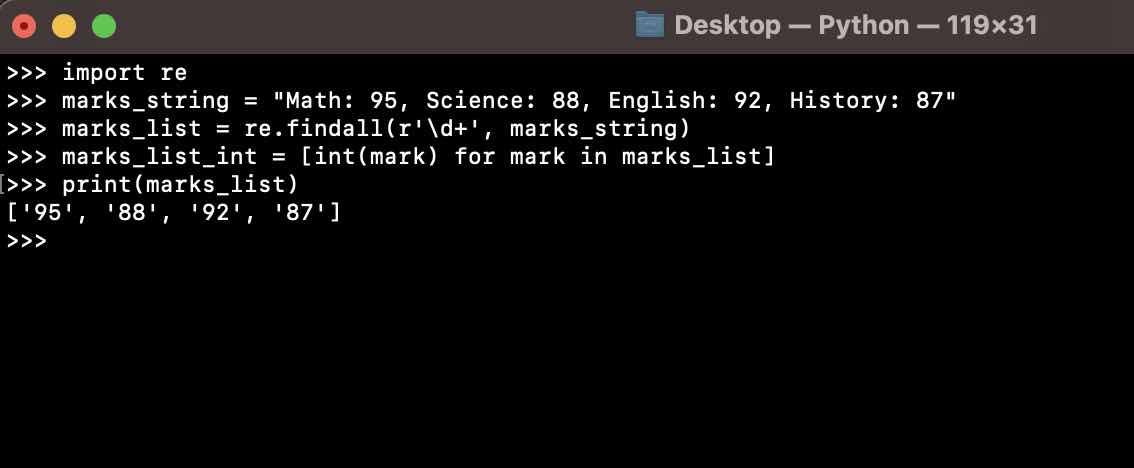
Let's take a look at another example.
Example 2: Extract List of Decimal Numbers from a String.import re
text = "Carrots: $1.99, Tomatoes: $2.50, Cabbage: $0.99, Bell Pepper: $1.25"
decimal_list = re.findall(r'\d+\.\d+', text)
decimal_list = [float(decimal) for decimal in decimal_list]
print(decimal_list)
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
- Write JSON to file in pretty-printed Format using Java Jackson - Java
- [Fix] TypeError: str object is not callable in Python - Python
- How to Validate String Date Format in Java - Java
- How to display Line Number in Eclipse IDE - Eclipse
- Install Node on Mac Ventura 13 - MacOS
- Bash Command to Check Disk Space - Bash
- Installing MongoDB on Linux/Unix/macOS/Ubuntu - MacOS
- Big Sur unsupported Mac [macOS] - MacOS