Tl;dr Summary of this Article:
- Code Complexity and Bugs: As software projects grow, complexity increases, making it easier for bugs to creep in. Bugs, akin to misplaced puzzle pieces, can lead to runtime issues, especially in production.
- Runtime Bugs: Runtime bugs, often caused by human errors, occur during program execution and can lead to crashes or unexpected behavior. They are not checked by the compiler.
- Compile-Time Bugs: Compile-time bugs are detected and reported by the compiler during code compilation, preventing the project from being completed until they are fixed.
- Generics' Role: Generics in Java, introduced in version 1.5, play a crucial role in improving type safety and mitigating runtime bugs.
- Type Safety Enhancement: Generics allow developers to specify the type of elements a collection can hold at compile time, preventing potential runtime type errors like ClassCastException.
- Type Casting: Generics eliminate the need for explicit type casting when retrieving elements from collections, improving code readability and reducing the risk of errors.
Code Complexity and Bugs
Imagine that you are writing some Java code, at first, your project is like a small puzzle. You know exactly what each piece looks like, and you can easily put them together.
But as your project grows, it's no longer just a simple puzzle. It becomes a massive, complex one with hundreds or even thousands of pieces (lines of code). This can get confusing, and it's easy to make mistakes, like putting the wrong pieces together.
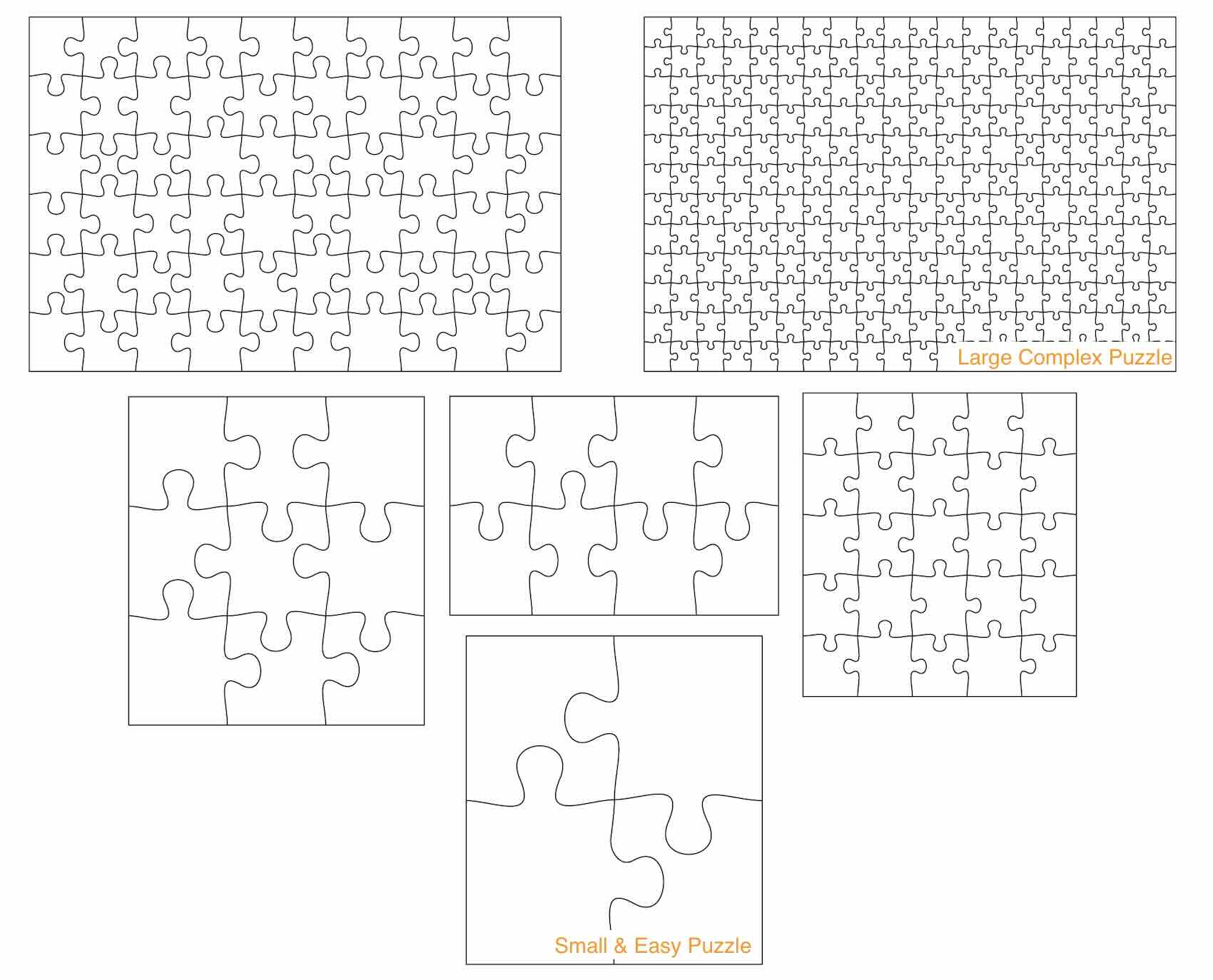
Some of these pieces can be tricky, they might fit in well at a place, but it may be the wrong match. Now, think about these pieces as bugs in your code, they don't show up until your program is running. That's when things can go wrong, and it's not fun to deal with problems when your software is already out there in the production environment.
It is a fact that software bugs are part and parcel of development. Smaller projects make spotting and fixing them easier, but as projects grow and evolve with changing teams and new features, project complexity increases, and bugs inevitably creep in, it is our job to minimize them as much as possible before the project moves to higher environments - Staging/UAT/Production.
The Creepy Runtime Bugs
The runtime bugs (exceptions) occur during the execution of a program and are typically indicative of errors in the program's logic. Unlike Checked Exceptions, which must be explicitly declared or caught, Runtime Exceptions are unchecked, meaning they do not need to be declared in a method's signature or caught explicitly.
Runtime Exceptions are the result of human errors introduced by developers during the coding process. These errors may include improper input validation, null pointer dereferences, improper casting, or arithmetic operations that can lead to unexpected behavior. Since the compiler does not check for RTEs at compile time, they can often go unnoticed until runtime, potentially causing program crashes or undesirable behavior in production.
The Compile time Bugs!
The compile time bugs are shown as an error message during code compilation. All IDEs like Eclipse, IntelliJ, or VS Code will show the line in red where there is a compilation error and the project will not complete until you fix them.
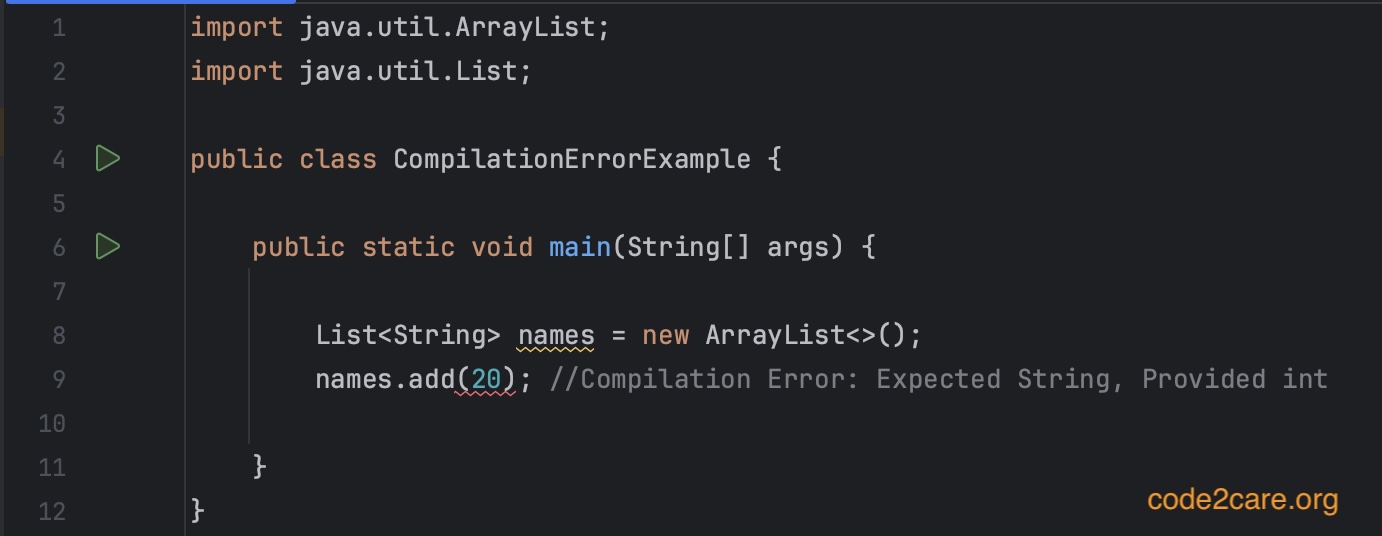
Now Let's Talk About Generics
Now that we have looked at how Runtime Exceptions can creep into production, the way to mitigate them is by converting them into CompileTime Exceptions so they can be avoided, below are some key motivations behind the introduction of Generics in Java 1.5.
Type Safety:
import java.util.ArrayList;
import java.util.List;
public class TypeSafetyExample {
public static void main(String[] args) {
List assortedList = new ArrayList();
assortedList.add("Hello");
assortedList.add(123);
for (Object item : assortedList) {
String element = (String) item;
System.out.println(element);
}
}
}
import java.util.ArrayList;
import java.util.List;
public class TypeSafetyExample {
public static void main(String[] args) {
List assortedList = new ArrayList();
assortedList.add("Hello");
assortedList.add(123);
for (Object item : assortedList) {
String element = (String) item;
System.out.println(element);
}
}
}
As you can see in the above code there was no Compilation error, but failed during runtime.
This is one of the primary motivations for introducing Generics was to introduce type safety in Java programs.
Before Generics, collections like ArrayList and HashMap could hold objects of any type, leading to potential runtime type errors (ClassCastException). Generics allow developers to specify the type of elements a collection can hold at compile time, preventing such errors.
List<String> list = new ArrayList<>();
list.add("Hello");
list.add(123);
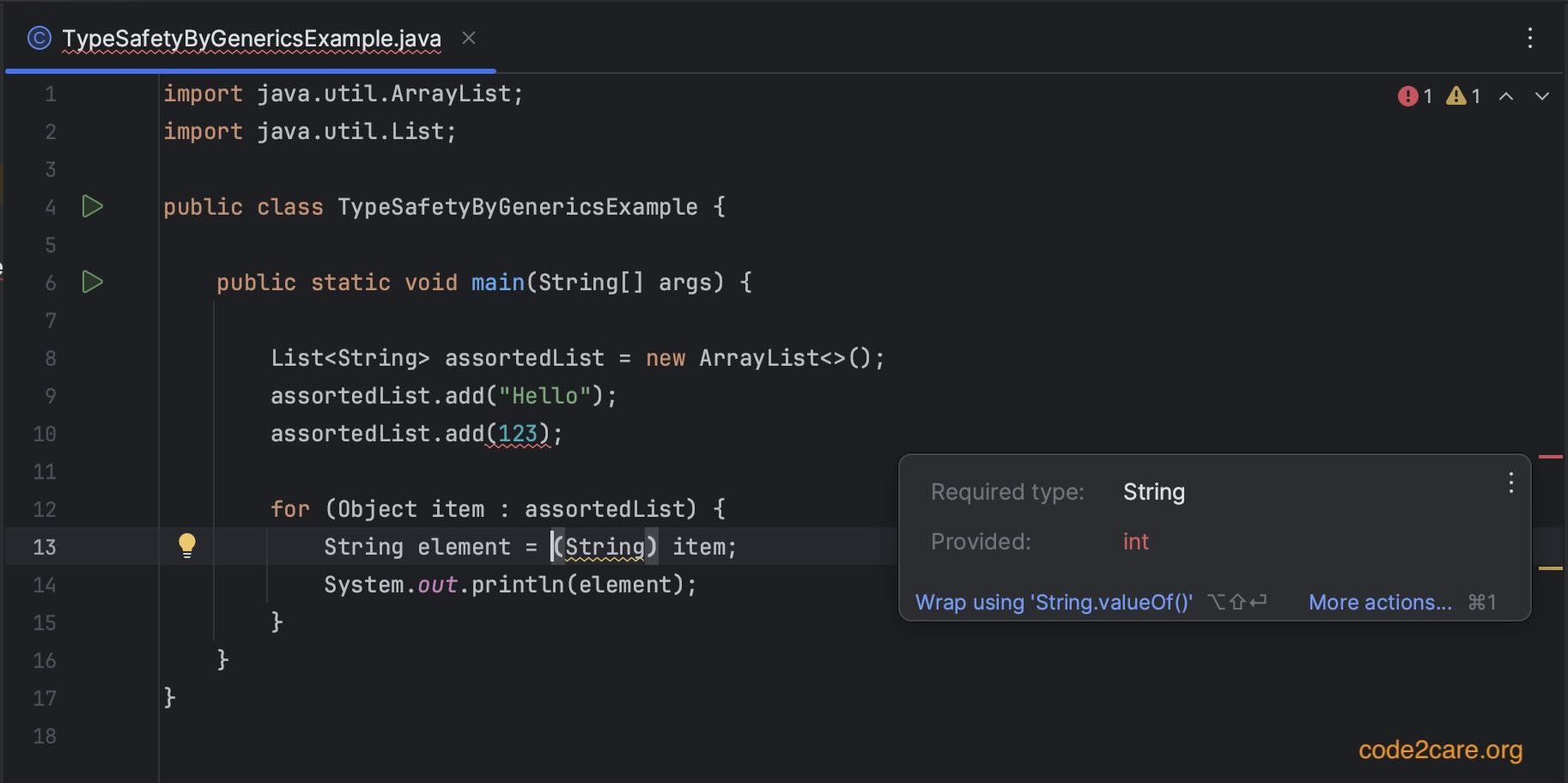
Eliminating Type Casting:
This can be seen as a byproduct of type casting, prior to Generics, developers often had to use explicit type casting when retrieving elements from collections. Generics eliminate the need for such casts, making code more readable and less error-prone.
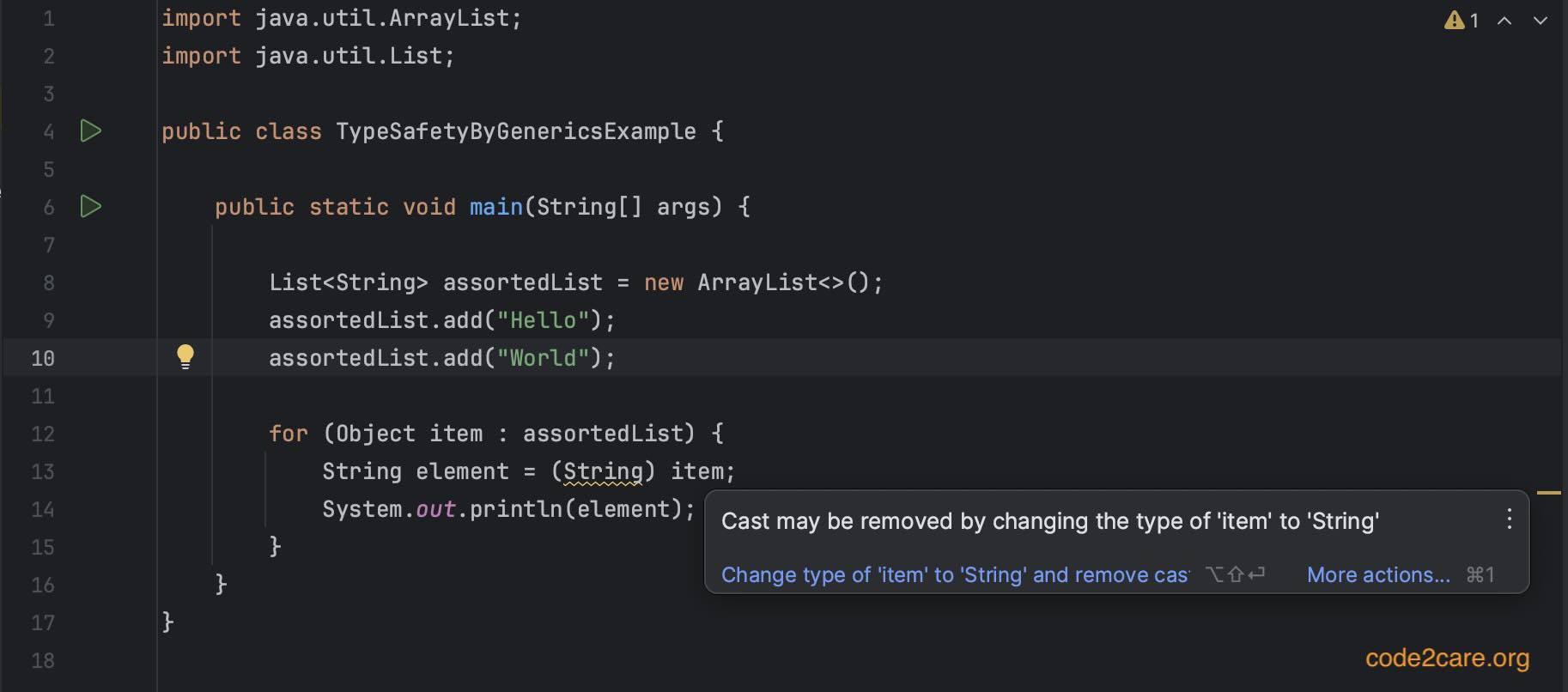
As you can see the compiler says that "casting can be removed" when we make use of collections with Generics.
Summary
Generics in Java address code complexity and improve type safety by detecting and preventing runtime bugs at compile time, leading to more reliable and maintainable software projects.
Some more advantages of Genetics
Generics are not just about collections, they help you write reusable code and algorithms, thus helping write lean & robust code. We shall cover these topics in our next article.
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- How to Clear Mac Terminal History - MacOS
- Audio Video Network protocols supported by Android OS Devices - Android
- The Zsh Shell - Mac Tutorial - MacOS
- Channel 50 SMSes received every few minutes Android Phones - Android
- Fix - Error:Invalid Gradle JDK configuration found (Android Studio) - Gradle
- ChatGPT Outage: Hmm...something seems to have gone wrong. Maybe try me again in a little bit. - HowTos
- Setup synonyms or alias or thesaurus in SharePoint - SharePoint
- How To Remove Only Conditional Formatting in Excel - Microsoft