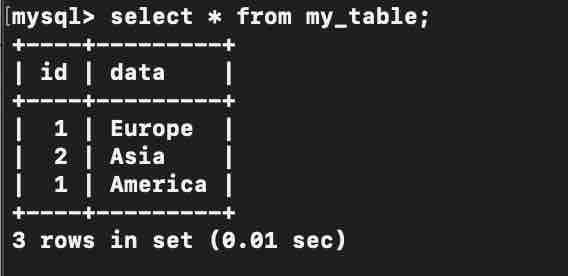
DELIMITER $$
CREATE DEFINER=`root`@`%` PROCEDURE `my_stored_proc`()
BEGIN
Select count(*) from my_table;
END$$
DELIMITER ;
Java Code:
import java.sql.CallableStatement;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
public class JdbcStoredProcedureExample {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
String url ="jdbc:mysql://localhost:3306/my_uat";
String userName="root";
String password ="root123";
Connection connection = DriverManager.getConnection(url,userName,password);
//Create the stored procedure call as a String
String call="call my_stored_proc()";
//Prepare CallableStatement
CallableStatement callableStatement = connection.prepareCall(call);
//Execute and fetch resultSet
ResultSet resultSet = callableStatement.executeQuery();
//Converting resultSet to ArrayList
while(resultSet.next()) {
System.out.println(resultSet.getInt(1));
}
}
}
Output: 3
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to Setup maven on Mac (macOS) - Mac-OS-X
- Nano Show Line Numbers - Linux
- wget Command on macOS Terminal - MacOS
- [fix] macOS Ventura - Python3 xcrun: error: invalid active developer path missing xcrun at CommandLineTools - MacOS
- Make Bootstrap Button look like a link - Bootstrap
- Safari appends .html extension to files that are downloaded - Mac-OS-X
- How to tar.gz a directory or folder Command - Linux
- [JEP 431] Java JDK 21 New Feature - Sequenced Collections - Java-JDK-21